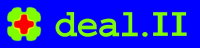 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
42 template <
int dim,
int spacedim>
45 const typename ::Triangulation<dim, spacedim>::MeshSmoothing
47 const bool check_for_distorted_cells)
49 check_for_distorted_cells)
50 , mpi_communicator(mpi_communicator)
54 #ifndef DEAL_II_WITH_MPI
61 template <
int dim,
int spacedim>
64 const ::Triangulation<dim, spacedim> &other_tria)
66 #ifndef DEAL_II_WITH_MPI
72 if (const ::parallel::TriangulationBase<dim, spacedim> *other_tria_x =
73 dynamic_cast<const ::parallel::TriangulationBase<dim, spacedim>
86 template <
int dim,
int spacedim>
95 number_cache.n_global_active_cells) +
100 template <
int dim,
int spacedim>
109 template <
int dim,
int spacedim>
111 : n_locally_owned_active_cells(0)
115 template <
int dim,
int spacedim>
122 template <
int dim,
int spacedim>
129 template <
int dim,
int spacedim>
136 template <
int dim,
int spacedim>
143 #ifdef DEAL_II_WITH_MPI
144 template <
int dim,
int spacedim>
168 if (cell->is_ghost())
185 this->mpi_communicator);
207 cell->level_subdomain_id());
220 std::vector<MPI_Request> requests(
222 unsigned int dummy = 0;
223 unsigned int req_counter = 0;
225 for (std::set<types::subdomain_id>::iterator it =
227 it != this->number_cache.level_ghost_owners.end();
230 ierr = MPI_Isend(&
dummy,
236 &requests[req_counter]);
240 for (std::set<types::subdomain_id>::iterator it =
242 it != this->number_cache.level_ghost_owners.end();
246 ierr = MPI_Recv(&
dummy,
256 if (requests.size() > 0)
258 ierr = MPI_Waitall(requests.size(),
260 MPI_STATUSES_IGNORE);
277 template <
int dim,
int spacedim>
286 template <
int dim,
int spacedim>
295 template <
int dim,
int spacedim>
296 const std::set<types::subdomain_id> &
304 template <
int dim,
int spacedim>
305 const std::set<types::subdomain_id> &
313 template <
int dim,
int spacedim>
314 std::map<unsigned int, std::set<::types::subdomain_id>>
323 template <
int dim,
int spacedim>
324 std::vector<types::boundary_id>
334 template <
int dim,
int spacedim>
335 std::vector<types::manifold_id>
345 template <
int dim,
int spacedim>
348 const typename ::Triangulation<dim, spacedim>::MeshSmoothing
362 #include "tria_base.inst"
unsigned int n_locally_owned_active_cells
MPI_Comm mpi_communicator
const std::set< types::subdomain_id > & level_ghost_owners() const
std::set< types::subdomain_id > level_ghost_owners
virtual std::vector< types::boundary_id > get_boundary_ids() const override
const std::set< types::subdomain_id > & ghost_owners() const
static ::ExceptionBase & ExcNotImplemented()
virtual std::vector< types::manifold_id > get_manifold_ids() const override
const bool check_for_distorted_cells
DistributedTriangulationBase(MPI_Comm mpi_communicator, const typename ::Triangulation< dim, spacedim >::MeshSmoothing smooth_grid=(::Triangulation< dim, spacedim >::none), const bool check_for_distorted_cells=false)
virtual std::size_t memory_consumption() const
virtual bool is_multilevel_hierarchy_constructed() const =0
static ::ExceptionBase & ExcNeedsMPI()
std::vector< T > compute_set_union(const std::vector< T > &vec, const MPI_Comm &comm)
types::global_cell_index n_global_active_cells
std::map< unsigned int, std::set<::types::subdomain_id > > compute_vertices_with_ghost_neighbors(const Triangulation< dim, spacedim > &tria)
#define DEAL_II_NAMESPACE_OPEN
std::enable_if< std::is_fundamental< T >::value, std::size_t >::type memory_consumption(const T &t)
T sum(const T &t, const MPI_Comm &mpi_communicator)
#define AssertThrowMPI(error_code)
void release_all_unused_memory()
types::subdomain_id locally_owned_subdomain() const override
virtual void update_number_cache()
virtual void copy_triangulation(const Triangulation< dim, spacedim > &other_tria)
const types::global_dof_index * dummy()
unsigned int n_locally_owned_active_cells() const
static ::ExceptionBase & ExcInternalError()
virtual const MPI_Comm & get_communicator() const
TriangulationBase(MPI_Comm mpi_communicator, const typename ::Triangulation< dim, spacedim >::MeshSmoothing smooth_grid=(::Triangulation< dim, spacedim >::none), const bool check_for_distorted_cells=false)
#define Assert(cond, exc)
unsigned int this_mpi_process(const MPI_Comm &mpi_communicator)
virtual unsigned int n_global_levels() const override
virtual std::map< unsigned int, std::set<::types::subdomain_id > > compute_vertices_with_ghost_neighbors() const
unsigned int n_mpi_processes(const MPI_Comm &mpi_communicator)
types::subdomain_id my_subdomain
const types::subdomain_id artificial_subdomain_id
cell_iterator begin(const unsigned int level=0) const
IteratorRange< active_cell_iterator > active_cell_iterators() const
#define DEAL_II_NAMESPACE_CLOSE
unsigned int n_global_levels
unsigned int n_levels() const
std::set< types::subdomain_id > ghost_owners
MeshSmoothing smooth_grid
T max(const T &t, const MPI_Comm &mpi_communicator)
virtual types::global_cell_index n_global_active_cells() const override
cell_iterator end() const