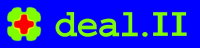 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_exceptions_h
17 #define dealii_exceptions_h
24 #include <type_traits>
26 #ifdef DEAL_II_WITH_CUDA
27 # include <cusolverSp.h>
28 # include <cusparse.h>
84 const char *exc_name);
91 what()
const noexcept
override;
133 const char *
function;
157 #ifdef DEAL_II_HAVE_GLIBC_STACKTRACE
197 # define DeclException0(Exception0) \
198 class Exception0 : public ::ExceptionBase \
221 # define DeclExceptionMsg(Exception, defaulttext) \
222 class Exception : public ::ExceptionBase \
225 Exception(const std::string &msg = defaulttext) \
228 virtual ~Exception() noexcept \
231 print_info(std::ostream &out) const override \
233 out << " " << arg << std::endl; \
237 const std::string arg; \
257 # define DeclException1(Exception1, type1, outsequence) \
258 class Exception1 : public ::ExceptionBase \
261 Exception1(type1 const &a1) \
264 virtual ~Exception1() noexcept \
267 print_info(std::ostream &out) const override \
269 out << " " outsequence << std::endl; \
294 # define DeclException2(Exception2, type1, type2, outsequence) \
295 class Exception2 : public ::ExceptionBase \
298 Exception2(type1 const &a1, type2 const &a2) \
302 virtual ~Exception2() noexcept \
305 print_info(std::ostream &out) const override \
307 out << " " outsequence << std::endl; \
333 # define DeclException3(Exception3, type1, type2, type3, outsequence) \
334 class Exception3 : public ::ExceptionBase \
337 Exception3(type1 const &a1, type2 const &a2, type3 const &a3) \
342 virtual ~Exception3() noexcept \
345 print_info(std::ostream &out) const override \
347 out << " " outsequence << std::endl; \
374 # define DeclException4(Exception4, type1, type2, type3, type4, outsequence) \
375 class Exception4 : public ::ExceptionBase \
378 Exception4(type1 const &a1, \
387 virtual ~Exception4() noexcept \
390 print_info(std::ostream &out) const override \
392 out << " " outsequence << std::endl; \
420 # define DeclException5( \
421 Exception5, type1, type2, type3, type4, type5, outsequence) \
422 class Exception5 : public ::ExceptionBase \
425 Exception5(type1 const &a1, \
436 virtual ~Exception5() noexcept \
439 print_info(std::ostream &out) const override \
441 out << " " outsequence << std::endl; \
473 # define DeclException0(Exception0) \
475 static ::ExceptionBase &Exception0()
496 # define DeclExceptionMsg(Exception, defaulttext) \
499 static ::ExceptionBase &Exception()
518 # define DeclException1(Exception1, type1, outsequence) \
521 static ::ExceptionBase &Exception1(type1 arg1)
541 # define DeclException2(Exception2, type1, type2, outsequence) \
544 static ::ExceptionBase &Exception2(type1 arg1, type2 arg2)
564 # define DeclException3(Exception3, type1, type2, type3, outsequence) \
567 static ::ExceptionBase &Exception3(type1 arg1, type2 arg2, type3 arg3)
587 # define DeclException4(Exception4, type1, type2, type3, type4, outsequence) \
590 static ::ExceptionBase &Exception4(type1 arg1, \
613 # define DeclException5( \
614 Exception5, type1, type2, type3, type4, type5, outsequence) \
617 static ::ExceptionBase &Exception5( \
618 type1 arg1, type2 arg2, type3 arg3, type4 arg4, type5 arg5)
645 "A piece of code is attempting a division by zero. This is "
646 "likely going to lead to results that make no sense.");
660 std::complex<double>,
661 <<
"In a significant number of places, deal.II checks that some intermediate "
662 <<
"value is a finite number (as opposed to plus or minus infinity, or "
663 <<
"NaN/Not a Number). In the current function, we encountered a number "
664 <<
"that is not finite (its value is " << arg1 <<
" and therefore "
665 <<
"violates the current assertion).\n\n"
666 <<
"This may be due to the fact that some operation in this function "
667 <<
"created such a value, or because one of the arguments you passed "
668 <<
"to the function already had this value from some previous "
669 <<
"operation. In the latter case, this function only triggered the "
670 <<
"error but may not actually be responsible for the computation of "
671 <<
"the number that is not finite.\n\n"
672 <<
"There are two common cases where this situation happens. First, your "
673 <<
"code (or something in deal.II) divides by zero in a place where this "
674 <<
"should not happen. Or, you are trying to solve a linear system "
675 <<
"with an unsuitable solver (such as an indefinite or non-symmetric "
676 <<
"linear system using a Conjugate Gradient solver); such attempts "
677 <<
"oftentimes yield an operation somewhere that tries to divide "
678 <<
"by zero or take the square root of a negative value.\n\n"
679 <<
"In any case, when trying to find the source of the error, "
680 <<
"recall that the location where you are getting this error is "
681 <<
"simply the first place in the program where there is a check "
682 <<
"that a number (e.g., an element of a solution vector) is in fact "
683 <<
"finite, but that the actual error that computed the number "
684 <<
"may have happened far earlier. To find this location, you "
685 <<
"may want to add checks for finiteness in places of your "
686 <<
"program visited before the place where this error is produced. "
687 <<
"One way to check for finiteness is to use the 'AssertIsFinite' "
695 "Your program tried to allocate some memory but this "
696 "allocation failed. Typically, this either means that "
697 "you simply do not have enough memory in your system, "
698 "or that you are (erroneously) trying to allocate "
699 "a chunk of memory that is simply beyond all reasonable "
700 "size, for example because the size of the object has "
701 "been computed incorrectly."
703 "In the current case, the request was for "
704 << arg1 <<
" bytes.");
712 <<
"Destroying memory handler while " << arg1
713 <<
" objects are still allocated.");
719 "An input/output error has occurred. There are a number of "
720 "reasons why this may be happening, both for reading and "
721 "writing operations."
723 "If this happens during an operation that tries to read "
724 "data: First, you may be "
725 "trying to read from a file that doesn't exist or that is "
726 "not readable given its file permissions. Second, deal.II "
727 "uses this error at times if it tries to "
728 "read information from a file but where the information "
729 "in the file does not correspond to the expected format. "
730 "An example would be a truncated file, or a mesh file "
731 "that contains not only sections that describe the "
732 "vertices and cells, but also sections for additional "
733 "data that deal.II does not understand."
735 "If this happens during an operation that tries to write "
736 "data: you may be trying to write to a file to which file "
737 "or directory permissions do not allow you to write. A "
738 "typical example is where you specify an output file in "
739 "a directory that does not exist.");
749 <<
"Could not open file " << arg1 <<
".");
760 "You are trying to use functionality in deal.II that is "
761 "currently not implemented. In many cases, this indicates "
762 "that there simply didn't appear much of a need for it, or "
763 "that the author of the original code did not have the "
764 "time to implement a particular case. If you hit this "
765 "exception, it is therefore worth the time to look into "
766 "the code to find out whether you may be able to "
767 "implement the missing functionality. If you do, please "
768 "consider providing a patch to the deal.II development "
769 "sources (see the deal.II website on how to contribute).");
792 "This exception -- which is used in many places in the "
793 "library -- usually indicates that some condition which "
794 "the author of the code thought must be satisfied at a "
795 "certain point in an algorithm, is not fulfilled. An "
796 "example would be that the first part of an algorithm "
797 "sorts elements of an array in ascending order, and "
798 "a second part of the algorithm later encounters an "
799 "element that is not larger than the previous one."
801 "There is usually not very much you can do if you "
802 "encounter such an exception since it indicates an error "
803 "in deal.II, not in your own program. Try to come up with "
804 "the smallest possible program that still demonstrates "
805 "the error and contact the deal.II mailing lists with it "
816 "You (or a place in the library) are trying to call a "
817 "function that is declared as a virtual function in a "
818 "base class but that has not been overridden in your "
821 "This exception happens in cases where the base class "
822 "cannot provide a useful default implementation for "
823 "the virtual function, but where we also do not want "
824 "to mark the function as abstract (i.e., with '=0' at the end) "
825 "because the function is not essential to the class in many "
826 "contexts. In cases like this, the base class provides "
827 "a dummy implementation that makes the compiler happy, but "
828 "that then throws the current exception."
830 "A concrete example would be the 'Function' class. It declares "
831 "the existence of 'value()' and 'gradient()' member functions, "
832 "and both are marked as 'virtual'. Derived classes have to "
833 "override these functions for the values and gradients of a "
834 "particular function. On the other hand, not every function "
835 "has a gradient, and even for those that do, not every program "
836 "actually needs to evaluate it. Consequently, there is no "
837 "*requirement* that a derived class actually override the "
838 "'gradient()' function (as there would be had it been marked "
839 "as abstract). But, since the base class cannot know how to "
840 "compute the gradient, if a derived class does not override "
841 "the 'gradient()' function and it is called anyway, then the "
842 "default implementation in the base class will simply throw "
845 "The exception you see is what happens in cases such as the "
846 "one just illustrated. To fix the problem, you need to "
847 "investigate whether the function being called should indeed have "
848 "been called; if the answer is 'yes', then you need to "
849 "implement the missing override in your class.");
869 <<
"You are trying to execute functionality that is "
870 <<
"impossible in " << arg1
871 <<
"d or simply does not make any sense.");
883 <<
"You are trying to execute functionality that is "
884 <<
"impossible in dimensions <" << arg1 <<
"," << arg2
885 <<
"> or simply does not make any sense.");
892 "In a check in the code, deal.II encountered a zero in "
893 "a place where this does not make sense. See the condition "
894 "that was being checked and that is printed further up "
895 "in the error message to get more information on what "
896 "the erroneous zero corresponds to.");
903 "The object you are trying to access is empty but it makes "
904 "no sense to attempt the operation you are trying on an "
917 <<
"Dimension " << arg1 <<
" not equal to " << arg2 <<
".");
927 <<
"Dimension " << arg1 <<
" neither equal to " << arg2
928 <<
" nor to " << arg3 <<
".");
947 <<
"Index " << arg1 <<
" is not in the half-open range [" << arg2 <<
","
950 " In the current case, this half-open range is in fact empty, "
951 "suggesting that you are accessing an element of an empty "
952 "collection such as a vector that has not been set to the "
971 template <
typename T>
977 <<
"Index " << arg1 <<
" is not in the half-open range [" << arg2 <<
","
980 " In the current case, this half-open range is in fact empty, "
981 "suggesting that you are accessing an element of an empty "
982 "collection such as a vector that has not been set to the "
992 <<
"Number " << arg1 <<
" must be larger than or equal "
998 template <
typename T>
1002 <<
"Number " << arg1 <<
" must be larger than or equal "
1012 <<
"Division " << arg1 <<
" by " << arg2
1013 <<
" has remainder different from zero.");
1024 "You are trying to use an iterator, but the iterator is "
1025 "in an invalid state. This may indicate that the iterator "
1026 "object has not been initialized, or that it has been "
1027 "moved beyond the end of the range of valid elements.");
1034 "You are trying to use an iterator, but the iterator is "
1035 "pointing past the end of the range of valid elements. "
1036 "It is not valid to dereference the iterator in this "
1058 "You are trying an operation on a vector that is only "
1059 "allowed if the vector has no ghost elements, but the "
1060 "vector you are operating on does have ghost elements. "
1061 "Specifically, vectors with ghost elements are read-only "
1062 "and cannot appear in operations that write into these "
1065 "See the glossary entry on 'Ghosted vectors' for more "
1076 "You are trying an operation of the form 'vector=s' with "
1077 "a nonzero scalar value 's'. However, such assignments "
1078 "are only allowed if the right hand side is zero.");
1085 "You are attempting to use functionality that is only available "
1086 "if deal.II was configured to use LAPACK, but cmake did not "
1087 "find a valid LAPACK library.");
1094 "You are attempting to use functionality that is only available "
1095 "if deal.II was configured to use MPI.");
1104 "You are attempting to use functionality that is only available "
1105 "if deal.II was configured to use NetCDF, but cmake did not "
1106 "find a valid NetCDF library.");
1113 "You are attempting to use functionality that is only available "
1114 "if deal.II was configured to use the function parser which "
1115 "relies on the muparser library, but cmake did not "
1116 "find a valid muparser library on your system and also did "
1117 "not choose the one that comes bundled with deal.II.");
1124 "You are attempting to use functionality that is only available "
1125 "if deal.II was configured to use Assimp, but cmake did not "
1126 "find a valid Assimp library.");
1128 #ifdef DEAL_II_WITH_CUDA
1141 <<
"There was an error in a cuSPARSE function: " << arg1);
1145 #ifdef DEAL_II_WITH_MPI
1174 print_info(std::ostream &out)
const override;
1178 #endif // DEAL_II_WITH_MPI
1303 template <
class ExceptionType>
1308 const char *
function,
1310 const char * exc_name,
1313 e.set_fields(file, line,
function, cond, exc_name);
1351 template <
class ExceptionType>
1355 const char *
function,
1357 const char * exc_name,
1358 ExceptionType
e) noexcept
1361 "The provided exception must inherit from ExceptionBase.");
1363 e.set_fields(file, line,
function, cond, exc_name);
1368 #ifdef DEAL_II_WITH_CUDA
1418 # ifdef DEAL_II_HAVE_BUILTIN_EXPECT
1419 # define Assert(cond, exc) \
1421 if (__builtin_expect(!(cond), false)) \
1422 ::deal_II_exceptions::internals::issue_error_noreturn( \
1423 ::deal_II_exceptions::internals:: \
1424 abort_or_throw_on_exception, \
1427 __PRETTY_FUNCTION__, \
1433 # define Assert(cond, exc) \
1436 ::deal_II_exceptions::internals::issue_error_noreturn( \
1437 ::deal_II_exceptions::internals:: \
1438 abort_or_throw_on_exception, \
1441 __PRETTY_FUNCTION__, \
1448 # define Assert(cond, exc) \
1482 # ifdef DEAL_II_HAVE_BUILTIN_EXPECT
1483 # define AssertNothrow(cond, exc) \
1485 if (__builtin_expect(!(cond), false)) \
1486 ::deal_II_exceptions::internals::issue_error_nothrow( \
1487 __FILE__, __LINE__, __PRETTY_FUNCTION__, #cond, #exc, exc); \
1490 # define AssertNothrow(cond, exc) \
1493 ::deal_II_exceptions::internals::issue_error_nothrow( \
1494 __FILE__, __LINE__, __PRETTY_FUNCTION__, #cond, #exc, exc); \
1498 # define AssertNothrow(cond, exc) \
1530 #ifdef DEAL_II_HAVE_BUILTIN_EXPECT
1531 # define AssertThrow(cond, exc) \
1533 if (__builtin_expect(!(cond), false)) \
1534 ::deal_II_exceptions::internals::issue_error_noreturn( \
1535 ::deal_II_exceptions::internals::throw_on_exception, \
1538 __PRETTY_FUNCTION__, \
1544 # define AssertThrow(cond, exc) \
1547 ::deal_II_exceptions::internals::issue_error_noreturn( \
1548 ::deal_II_exceptions::internals::throw_on_exception, \
1551 __PRETTY_FUNCTION__, \
1579 #define AssertDimension(dim1, dim2) \
1580 Assert(static_cast<typename ::internal::argument_type<void( \
1581 typename std::common_type<decltype(dim1), \
1582 decltype(dim2)>::type)>::type>(dim1) == \
1583 static_cast<typename ::internal::argument_type<void( \
1584 typename std::common_type<decltype(dim1), \
1585 decltype(dim2)>::type)>::type>(dim2), \
1586 ::ExcDimensionMismatch((dim1), (dim2)))
1607 #define AssertVectorVectorDimension(VEC, DIM1, DIM2) \
1608 AssertDimension(VEC.size(), DIM1); \
1609 for (const auto &subvector : VEC) \
1612 AssertDimension(subvector.size(), DIM2); \
1620 template <
typename T>
1622 template <
typename T,
typename U>
1649 #define AssertIndexRange(index, range) \
1651 static_cast<typename ::internal::argument_type<void( \
1652 typename std::common_type<decltype(index), decltype(range)>::type)>:: \
1654 static_cast<typename ::internal::argument_type<void( \
1655 typename std::common_type<decltype(index), decltype(range)>::type)>:: \
1657 ExcIndexRangeType<typename ::internal::argument_type<void( \
1658 typename std::common_type<decltype(index), decltype(range)>::type)>:: \
1659 type>((index), 0, (range)))
1681 #define AssertIsFinite(number) \
1682 Assert(::numbers::is_finite(number), \
1683 ::ExcNumberNotFinite(std::complex<double>(number)))
1685 #ifdef DEAL_II_WITH_MPI
1707 # define AssertThrowMPI(error_code) \
1708 AssertThrow(error_code == MPI_SUCCESS, ::ExcMPI(error_code))
1710 # define AssertThrowMPI(error_code) \
1712 #endif // DEAL_II_WITH_MPI
1714 #ifdef DEAL_II_WITH_CUDA
1734 # define AssertCuda(error_code) \
1735 Assert(error_code == cudaSuccess, \
1736 ::ExcCudaError(cudaGetErrorString(error_code)))
1738 # define AssertCuda(error_code) \
1740 (void)(error_code); \
1762 # define AssertNothrowCuda(error_code) \
1763 AssertNothrow(error_code == cudaSuccess, \
1764 ::ExcCudaError(cudaGetErrorString(error_code)))
1766 # define AssertNothrowCuda(error_code) \
1768 (void)(error_code); \
1791 # define AssertCudaKernel() \
1793 cudaError_t local_error_code = cudaPeekAtLastError(); \
1794 AssertCuda(local_error_code); \
1795 local_error_code = cudaDeviceSynchronize(); \
1796 AssertCuda(local_error_code) \
1799 # define AssertCudaKernel() \
1822 # define AssertCusparse(error_code) \
1824 error_code == CUSPARSE_STATUS_SUCCESS, \
1826 deal_II_exceptions::internals::get_cusparse_error_string( \
1829 # define AssertCusparse(error_code) \
1831 (void)(error_code); \
1853 # define AssertNothrowCusparse(error_code) \
1855 error_code == CUSPARSE_STATUS_SUCCESS, \
1857 deal_II_exceptions::internals::get_cusparse_error_string( \
1860 # define AssertNothrowCusparse(error_code) \
1862 (void)(error_code); \
1885 # define AssertCusolver(error_code) \
1887 error_code == CUSOLVER_STATUS_SUCCESS, \
1889 deal_II_exceptions::internals::get_cusolver_error_string( \
1892 # define AssertCusolver(error_code) \
1894 (void)(error_code); \
#define DeclExceptionMsg(Exception, defaulttext)
void issue_error_noreturn(ExceptionHandling handling, const char *file, int line, const char *function, const char *cond, const char *exc_name, ExceptionType e)
static ::ExceptionBase & ExcNeedsAssimp()
static ::ExceptionBase & ExcIO()
bool allow_abort_on_exception
static ::ExceptionBase & ExcNeedsNetCDF()
static ::ExceptionBase & ExcNotImplemented()
static ::ExceptionBase & ExcOutOfMemory(std::size_t arg1)
static ::ExceptionBase & ExcInvalidState()
virtual const char * what() const noexcept override
void generate_message() const
SymmetricTensor< 2, dim, Number > e(const Tensor< 2, dim, Number > &F)
static ::ExceptionBase & ExcDivideByZero()
static ::ExceptionBase & ExcFileNotOpen(std::string arg1)
static ::ExceptionBase & ExcLowerRange(int arg1, int arg2)
@ abort_or_throw_on_exception
std::string get_cusparse_error_string(const cusparseStatus_t error_code)
static ::ExceptionBase & ExcDimensionMismatch2(int arg1, int arg2, int arg3)
static ::ExceptionBase & ExcInvalidIterator()
const char * get_exc_name() const
static ::ExceptionBase & ExcPureFunctionCalled()
virtual void print_info(std::ostream &out) const override
static ::ExceptionBase & ExcNeedsMPI()
void abort(const ExceptionBase &exc) noexcept
static ::ExceptionBase & ExcIndexRange(int arg1, int arg2, int arg3)
static ::ExceptionBase & ExcMessage(std::string arg1)
static ::ExceptionBase & ExcEmptyObject()
ExcMPI(const int error_code)
static ::ExceptionBase & ExcMemoryLeak(int arg1)
static ::ExceptionBase & ExcLowerRangeType(T arg1, T arg2)
void * raw_stacktrace[25]
#define DEAL_II_NAMESPACE_OPEN
void disable_abort_on_exception()
static ::ExceptionBase & ExcIndexRangeType(T arg1, T arg2, T arg3)
#define DeclException3(Exception3, type1, type2, type3, outsequence)
#define DeclException1(Exception1, type1, outsequence)
static ::ExceptionBase & ExcNotInitialized()
void suppress_stacktrace_in_exceptions()
void do_issue_error_nothrow(const ExceptionBase &e) noexcept
static ::ExceptionBase & ExcCudaError(const char *arg1)
static ::ExceptionBase & ExcInternalError()
static ::ExceptionBase & ExcNotMultiple(int arg1, int arg2)
void issue_error_nothrow(const char *file, int line, const char *function, const char *cond, const char *exc_name, ExceptionType e) noexcept
static ::ExceptionBase & ExcImpossibleInDim(int arg1)
#define DeclException0(Exception0)
static ::ExceptionBase & ExcImpossibleInDimSpacedim(int arg1, int arg2)
virtual void print_info(std::ostream &out) const
static ::ExceptionBase & ExcGhostsPresent()
void set_fields(const char *file, const int line, const char *function, const char *cond, const char *exc_name)
static ::ExceptionBase & ExcZero()
void print_exc_data(std::ostream &out) const
static ::ExceptionBase & ExcDimensionMismatch(std::size_t arg1, std::size_t arg2)
ExceptionBase operator=(const ExceptionBase &)=delete
static ::ExceptionBase & ExcScalarAssignmentOnlyForZeroValue()
static ::ExceptionBase & ExcCusparseError(std::string arg1)
std::string get_cusolver_error_string(const cusolverStatus_t error_code)
static ::ExceptionBase & ExcNumberNotFinite(std::complex< double > arg1)
void set_additional_assert_output(const char *const p)
#define DEAL_II_NAMESPACE_CLOSE
static ::ExceptionBase & ExcNeedsLAPACK()
#define DeclException2(Exception2, type1, type2, outsequence)
virtual ~ExceptionBase() noexcept override
void print_stack_trace(std::ostream &out) const
static ::ExceptionBase & ExcIteratorPastEnd()
static ::ExceptionBase & ExcNeedsFunctionparser()