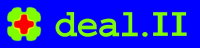 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_tria_iterator_h
17 # define dealii_tria_iterator_h
37 template <
int dim,
int spacedim>
39 template <
int,
int,
int>
231 template <
typename Accessor>
271 template <
typename OtherAccessor>
283 const typename AccessorType::AccessorData *local_data =
nullptr);
294 template <
typename OtherAccessor>
305 Accessor::space_dimension> &tria_accessor,
306 const typename Accessor::AccessorData * local_data);
312 template <
typename OtherAccessor>
319 template <
typename OtherAccessor>
481 template <
class StreamType>
483 print(StreamType &out)
const;
513 <<
"You tried to dereference a cell iterator for which this "
514 <<
"is not possible. More information on this iterator: "
515 <<
"level=" << arg1.level() <<
", index=" << arg1.index()
528 <<
"You tried to dereference an iterator for which this "
529 <<
"is not possible. More information on this iterator: "
530 <<
"index=" << arg1.index() <<
", state="
561 template <
typename SomeAccessor>
563 template <
typename SomeAccessor>
565 template <
typename SomeAccessor>
577 template <
typename Accessor>
616 const typename Accessor::AccessorData *local_data =
nullptr);
622 template <
typename OtherAccessor>
634 template <
typename OtherAccessor>
644 Accessor::space_dimension> &tria_accessor,
645 const typename Accessor::AccessorData * local_data);
652 template <
typename OtherAccessor>
659 template <
typename OtherAccessor>
679 template <
class OtherAccessor>
688 template <
class OtherAccessor>
758 template <
typename Accessor>
808 const typename Accessor::AccessorData *local_data = 0);
819 template <
typename OtherAccessor>
830 Accessor::space_dimension> &tria_accessor,
831 const typename Accessor::AccessorData * local_data);
844 template <
typename OtherAccessor>
871 template <
class OtherAccessor>
880 template <
class OtherAccessor>
889 template <
class OtherAccessor>
952 template <
typename Accessor>
959 template <
typename Accessor>
960 template <
typename OtherAccessor>
967 template <
typename Accessor>
968 template <
typename OtherAccessor>
971 : accessor(i.accessor)
976 template <
typename Accessor>
977 template <
typename OtherAccessor>
980 : accessor(i.accessor)
985 template <
typename Accessor>
986 template <
typename OtherAccessor>
989 : accessor(i.accessor)
994 template <
typename Accessor>
997 Assert(Accessor::structure_dimension != Accessor::dimension ||
999 ExcDereferenceInvalidCell(accessor));
1000 Assert(Accessor::structure_dimension == Accessor::dimension ||
1009 template <
typename Accessor>
1012 Assert(Accessor::structure_dimension != Accessor::dimension ||
1014 ExcDereferenceInvalidCell(accessor));
1015 Assert(Accessor::structure_dimension == Accessor::dimension ||
1024 template <
typename Accessor>
1033 template <
typename Accessor>
1041 template <
typename Accessor>
1049 template <
typename Accessor>
1053 return accessor.state();
1058 template <
typename Accessor>
1068 Assert(&accessor.get_triangulation() == &other.
accessor.get_triangulation(),
1069 ExcInvalidComparison());
1077 return ((**
this) < (*other));
1082 template <
typename Accessor>
1087 return (other < *
this);
1092 template <
typename Accessor>
1104 template <
typename Accessor>
1116 template <
typename Accessor>
1117 template <
class StreamType>
1121 if (Accessor::structure_dimension == Accessor::dimension)
1122 out << accessor.level() <<
"." << accessor.index();
1124 out << accessor.index();
1129 template <
typename Accessor>
1138 template <
typename Accessor>
1139 template <
typename OtherAccessor>
1147 template <
typename Accessor>
1148 template <
typename OtherAccessor>
1156 template <
typename Accessor>
1157 template <
typename OtherAccessor>
1174 template <
typename Accessor>
1175 template <
typename OtherAccessor>
1191 template <
typename Accessor>
1192 template <
typename OtherAccessor>
1200 template <
typename Accessor>
1201 template <
typename OtherAccessor>
1228 template <
typename Accessor>
1229 inline std::ostream &
1245 template <
typename Accessor>
1246 inline std::ostream &
1262 template <
typename Accessor>
1263 inline std::ostream &
1276 # include "tria_iterator.templates.h"
std::size_t memory_consumption() const
TriaIterator< Accessor > & operator=(const TriaIterator< Accessor > &)
const Accessor & operator*() const
static ::ExceptionBase & ExcInvalidComparison()
typename TriaRawIterator< Accessor >::difference_type difference_type
static ::ExceptionBase & ExcDereferenceInvalidObject(AccessorType arg1)
bool operator>(const TriaRawIterator &) const
MatrixTableIterators::Accessor< TransposeTable< T >, Constness, MatrixTableIterators::Storage::column_major > Accessor
typename TriaRawIterator< Accessor >::iterator_category iterator_category
typename TriaRawIterator< Accessor >::value_type value_type
TriaIterator< Accessor > & operator++()
@ valid
Iterator points to a valid object.
typename TriaRawIterator< Accessor >::pointer pointer
TriaActiveIterator< Accessor > & operator++()
void print(StreamType &out) const
Point< dim, typename ProductType< Number, typename EnableIfScalar< OtherNumber >::type >::type > operator*(const OtherNumber) const
@ past_the_end
Iterator reached end of container.
TriaRawIterator & operator--()
bool operator!=(const TriaRawIterator &) const
#define DEAL_II_NAMESPACE_OPEN
TriaRawIterator & operator=(const TriaRawIterator &)
const Accessor & access_any() const
static ::ExceptionBase & ExcDereferenceInvalidCell(Accessor arg1)
static ::ExceptionBase & ExcAssignmentOfUnusedObject()
#define DeclException1(Exception1, type1, outsequence)
bool operator<(const TriaRawIterator &) const
IteratorState::IteratorStates state() const
static ::ExceptionBase & ExcAssignmentOfInactiveObject()
TriaIterator< Accessor > & operator--()
#define Assert(cond, exc)
friend class TriaRawIterator
OutputOperator< VectorType > & operator<<(OutputOperator< VectorType > &out, unsigned int step)
std::bidirectional_iterator_tag iterator_category
#define DeclException0(Exception0)
@ invalid
Iterator is invalid, probably due to an error.
const Accessor * operator->() const
static ::ExceptionBase & ExcAdvanceInvalidObject()
typename TriaRawIterator< Accessor >::reference reference
static ::ExceptionBase & ExcDereferenceInvalidObject(Accessor arg1)
#define DEAL_II_NAMESPACE_CLOSE
TriaActiveIterator< Accessor > & operator=(const TriaActiveIterator< Accessor > &)
TriaRawIterator & operator++()
TriaActiveIterator< Accessor > & operator--()
bool operator==(const TriaRawIterator &) const