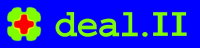 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_dof_accessor_h
17 #define dealii_dof_accessor_h
34 template <
typename number>
36 template <
typename number>
38 template <
typename number>
41 template <
typename Accessor>
49 namespace DoFCellAccessorImplementation
51 struct Implementation;
54 namespace DoFHandlerImplementation
56 struct Implementation;
59 struct Implementation;
65 namespace DoFHandlerImplementation
67 struct Implementation;
81 namespace DoFAccessorImplementation
100 template <
int structdim,
int dim,
int spacedim>
116 template <
int dim,
int spacedim>
210 template <
int structdim,
typename DoFHandlerType,
bool level_dof_access>
214 DoFHandlerType::dimension,
222 static const unsigned int dimension = DoFHandlerType::dimension;
234 using BaseClass = typename ::internal::DoFAccessorImplementation::
235 Inheritance<structdim, dimension, space_dimension>::BaseClass;
271 DoFHandlerType::space_dimension> *tria,
288 template <
int structdim2,
int dim2,
int spacedim2>
295 template <
int dim2,
class DoFHandlerType2,
bool level_dof_access2>
301 template <
bool level_dof_access2>
325 const DoFHandlerType &
331 template <
bool level_dof_access2>
341 DoFHandlerType::dimension,
342 DoFHandlerType::space_dimension> &da);
362 child(
const unsigned int c)
const;
369 typename ::internal::DoFHandlerImplementation::
370 Iterators<DoFHandlerType, level_dof_access>::line_iterator
371 line(
const unsigned int i)
const;
378 typename ::internal::DoFHandlerImplementation::
379 Iterators<DoFHandlerType, level_dof_access>::quad_iterator
380 quad(
const unsigned int i)
const;
429 std::vector<types::global_dof_index> &dof_indices,
430 const unsigned int fe_index = DoFHandlerType::default_fe_index)
const;
441 std::vector<types::global_dof_index> &dof_indices,
442 const unsigned int fe_index = DoFHandlerType::default_fe_index)
const;
450 const std::vector<types::global_dof_index> &dof_indices,
451 const unsigned int fe_index = DoFHandlerType::default_fe_index);
472 const unsigned int vertex,
473 const unsigned int i,
474 const unsigned int fe_index = DoFHandlerType::default_fe_index)
const;
484 const unsigned int vertex,
485 const unsigned int i,
486 const unsigned int fe_index = DoFHandlerType::default_fe_index)
const;
517 const unsigned int i,
518 const unsigned int fe_index = DoFHandlerType::default_fe_index)
const;
566 std::set<unsigned int>
586 DoFHandlerType::space_dimension> &
587 get_fe(
const unsigned int fe_index)
const;
599 "This accessor object has not been "
600 "associated with any DoFHandler object.");
654 template <
int dim2,
class DoFHandlerType2,
bool level_dof_access2>
662 template <
int dim2,
class DoFHandlerType2,
bool level_dof_access2>
693 const unsigned int i,
695 const unsigned int fe_index = DoFHandlerType::default_fe_index)
const;
699 const unsigned int i,
721 const unsigned int vertex,
722 const unsigned int i,
724 const unsigned int fe_index = DoFHandlerType::default_fe_index)
const;
729 const unsigned int vertex,
730 const unsigned int i,
732 const unsigned int fe_index = DoFHandlerType::default_fe_index)
const;
738 template <
int,
class,
bool>
744 template <
int dim,
int spacedim>
746 template <
int dim,
int spacedim>
749 friend struct ::internal::DoFHandlerImplementation::Policy::
751 friend struct ::internal::DoFHandlerImplementation::Implementation;
752 friend struct ::internal::hp::DoFHandlerImplementation::Implementation;
753 friend struct ::internal::DoFCellAccessorImplementation::Implementation;
754 friend struct ::internal::DoFAccessorImplementation::Implementation;
768 template <
template <
int,
int>
class DoFHandlerType,
770 bool level_dof_access>
771 class DoFAccessor<0, DoFHandlerType<1, spacedim>, level_dof_access>
840 const DoFHandlerType<1, spacedim> *
dof_handler = 0);
854 template <
int structdim2,
int dim2,
int spacedim2>
861 template <
int dim2,
class DoFHandlerType2,
bool level_dof_access2>
884 const DoFHandlerType<1, spacedim> &
890 template <
bool level_dof_access2>
893 const DoFAccessor<0, DoFHandlerType<1, spacedim>, level_dof_access2> &a);
915 child(
const unsigned int c)
const;
923 typename ::internal::DoFHandlerImplementation::
924 Iterators<DoFHandlerType<1, spacedim>, level_dof_access>::line_iterator
925 line(
const unsigned int i)
const;
933 typename ::internal::DoFHandlerImplementation::
934 Iterators<DoFHandlerType<1, spacedim>, level_dof_access>::quad_iterator
935 quad(
const unsigned int i)
const;
981 std::vector<types::global_dof_index> &dof_indices,
982 const unsigned int fe_index = AccessorData::default_fe_index)
const;
993 std::vector<types::global_dof_index> &dof_indices,
994 const unsigned int fe_index = AccessorData::default_fe_index)
const;
1015 const unsigned int vertex,
1016 const unsigned int i,
1017 const unsigned int fe_index = AccessorData::default_fe_index)
const;
1035 const unsigned int fe_index = AccessorData::default_fe_index)
const;
1085 DoFHandlerType<1, spacedim>::space_dimension> &
1086 get_fe(
const unsigned int fe_index)
const;
1098 "This accessor object has not been "
1099 "associated with any DoFHandler object.");
1142 template <
int dim2,
class DoFHandlerType2,
bool level_dof_access2>
1150 template <
int dim2,
class DoFHandlerType2,
bool level_dof_access2>
1179 const unsigned int i,
1181 const unsigned int fe_index = AccessorData::default_fe_index)
const;
1202 const unsigned int vertex,
1203 const unsigned int i,
1205 const unsigned int fe_index = AccessorData::default_fe_index)
const;
1220 friend struct ::internal::DoFHandlerImplementation::Policy::
1222 friend struct ::internal::DoFHandlerImplementation::Implementation;
1223 friend struct ::internal::hp::DoFHandlerImplementation::Implementation;
1224 friend struct ::internal::DoFCellAccessorImplementation::Implementation;
1253 template <
int structdim,
int dim,
int spacedim = dim>
1271 const int level = -1,
1272 const int index = -1,
1288 template <
typename OtherAccessor>
1299 const unsigned int fe_index =
1320 template <
typename DoFHandlerType,
bool level_dof_access>
1329 static const unsigned int dim = DoFHandlerType::dimension;
1334 static const unsigned int spacedim = DoFHandlerType::space_dimension;
1373 DoFHandlerType::space_dimension> *tria,
1390 template <
int structdim2,
int dim2,
int spacedim2>
1397 template <
int dim2,
class DoFHandlerType2,
bool level_dof_access2>
1443 neighbor(
const unsigned int i)
const;
1467 child(
const unsigned int i)
const;
1476 face(
const unsigned int i)
const;
1493 const unsigned int subface_no)
const;
1503 const unsigned int subface_no)
const;
1530 template <
class InputVector,
typename number>
1548 template <
class InputVector,
typename ForwardIterator>
1551 ForwardIterator local_values_begin,
1552 ForwardIterator local_values_end)
const;
1571 template <
class InputVector,
typename ForwardIterator>
1575 const InputVector & values,
1576 ForwardIterator local_values_begin,
1577 ForwardIterator local_values_end)
const;
1600 template <
class OutputVector,
typename number>
1603 OutputVector & values)
const;
1636 template <
class InputVector,
typename number>
1639 const InputVector &values,
1641 const unsigned int fe_index = DoFHandlerType::default_fe_index)
const;
1695 template <
class OutputVector,
typename number>
1699 OutputVector & values,
1700 const unsigned int fe_index = DoFHandlerType::default_fe_index)
const;
1710 template <
typename number,
typename OutputVector>
1713 OutputVector & global_destination)
const;
1723 template <
typename ForwardIterator,
typename OutputVector>
1726 ForwardIterator local_source_end,
1727 OutputVector & global_destination)
const;
1739 template <
typename ForwardIterator,
typename OutputVector>
1743 ForwardIterator local_source_begin,
1744 ForwardIterator local_source_end,
1745 OutputVector & global_destination)
const;
1753 template <
typename number,
typename OutputMatrix>
1756 OutputMatrix &global_destination)
const;
1762 template <
typename number,
typename OutputMatrix,
typename OutputVector>
1766 OutputMatrix & global_matrix,
1767 OutputVector & global_vector)
const;
1795 std::vector<types::global_dof_index> &dof_indices)
const;
1833 get_dof_indices(std::vector<types::global_dof_index> &dof_indices)
const;
1867 DoFHandlerType::space_dimension> &
1935 set_dof_indices(
const std::vector<types::global_dof_index> &dof_indices);
1975 DoFHandlerType::space_dimension> &
2036 template <
int dim,
int spacedim>
2038 friend struct ::internal::DoFCellAccessorImplementation::Implementation;
2042 template <
int sd,
typename DoFHandlerType,
bool level_dof_access>
2046 return level_dof_access;
2051 template <
int structdim,
int dim,
int spacedim>
2052 template <
typename OtherAccessor>
2054 const OtherAccessor &)
2057 ExcMessage(
"You are attempting an illegal conversion between "
2058 "iterator/accessor types. The constructor you call "
2059 "only exists to make certain template constructs "
2060 "easier to write as dimension independent code but "
2061 "the conversion is not valid in the current context."));
2069 #include "dof_accessor.templates.h"
void set_vertex_dof_index(const unsigned int vertex, const unsigned int i, const types::global_dof_index index, const unsigned int fe_index=DoFHandlerType::default_fe_index) const
unsigned int active_fe_index() const
#define DeclExceptionMsg(Exception, defaulttext)
void get_interpolated_dof_values(const InputVector &values, Vector< number > &interpolated_values, const unsigned int fe_index=DoFHandlerType::default_fe_index) const
void copy_from(const DoFAccessor< structdim, DoFHandlerType, level_dof_access2 > &a)
typename ::internal::DoFHandlerImplementation::Iterators< DoFHandlerType, level_dof_access >::quad_iterator quad(const unsigned int i) const
void set_mg_dof_indices(const std::vector< types::global_dof_index > &dof_indices)
const FiniteElement< DoFHandlerType::dimension, DoFHandlerType::space_dimension > & get_future_fe() const
typename TriaAccessorBase< structdim, dim, spacedim >::AccessorData AccessorData
void distribute_local_to_global(const Vector< number > &local_source, OutputVector &global_destination) const
static bool is_level_cell()
TriaIterator< DoFCellAccessor< DoFHandlerType, level_dof_access > > parent() const
TriaIterator< DoFAccessor< structdim, DoFHandlerType, level_dof_access > > child(const unsigned int c) const
void get_mg_dof_indices(std::vector< types::global_dof_index > &dof_indices) const
std::array< face_iterator, GeometryInfo< DoFHandlerType::dimension >::faces_per_cell > face_iterators() const
bool future_fe_index_set() const
void set_dof_index(const unsigned int i, const types::global_dof_index index, const unsigned int fe_index=DoFHandler< dim, spacedim >::default_fe_index) const
TriaIterator< DoFCellAccessor< DoFHandlerType, level_dof_access > > periodic_neighbor(const unsigned int i) const
unsigned int future_fe_index() const
TriaIterator< DoFAccessor< DoFHandlerType::dimension - 1, DoFHandlerType, level_dof_access > > face_iterator
static const unsigned int dim
static const unsigned int spacedim
typename ::internal::DoFAccessorImplementation::Inheritance< structdim, dimension, space_dimension >::BaseClass BaseClass
bool operator==(const DoFAccessor< dim2, DoFHandlerType2, level_dof_access2 > &) const
DoFCellAccessor< DoFHandlerType, level_dof_access > & operator=(const DoFCellAccessor< DoFHandlerType, level_dof_access > &da)=delete
Point< spacedim > & vertex(const unsigned int i) const
const FiniteElement< DoFHandlerType::dimension, DoFHandlerType::space_dimension > & get_fe() const
void set_mg_dof_indices(const int level, const std::vector< types::global_dof_index > &dof_indices, const unsigned int fe_index=DoFHandlerType::default_fe_index)
static const unsigned int dimension
void set_dof_handler(DoFHandlerType *dh)
typename InvalidAccessor< structdim, dim, spacedim >::AccessorData AccessorData
static ::ExceptionBase & ExcNotActive()
DoFHandlerType AccessorData
void get_mg_dof_indices(const int level, std::vector< types::global_dof_index > &dof_indices, const unsigned int fe_index=DoFHandlerType::default_fe_index) const
static ::ExceptionBase & ExcMessage(std::string arg1)
types::global_dof_index dof_index(const unsigned int i, const unsigned int fe_index=DoFHandlerType::default_fe_index) const
#define DEAL_II_NAMESPACE_OPEN
static ::ExceptionBase & ExcMatrixDoesNotMatch()
void set_mg_vertex_dof_index(const int level, const unsigned int vertex, const unsigned int i, const types::global_dof_index index, const unsigned int fe_index=DoFHandlerType::default_fe_index) const
void set_dof_values_by_interpolation(const Vector< number > &local_values, OutputVector &values, const unsigned int fe_index=DoFHandlerType::default_fe_index) const
void get_dof_indices(std::vector< types::global_dof_index > &dof_indices, const unsigned int fe_index=DoFHandlerType::default_fe_index) const
void get_active_or_mg_dof_indices(std::vector< types::global_dof_index > &dof_indices) const
void set_active_fe_index(const unsigned int i) const
TriaIterator< DoFCellAccessor< DoFHandlerType, level_dof_access > > neighbor_child_on_subface(const unsigned int face_no, const unsigned int subface_no) const
std::set< unsigned int > get_active_fe_indices() const
void get_dof_indices(std::vector< types::global_dof_index > &dof_indices) const
TriaIterator< DoFCellAccessor< DoFHandlerType, level_dof_access > > neighbor_or_periodic_neighbor(const unsigned int i) const
DoFInvalidAccessor(const Triangulation< dim, spacedim > *parent=0, const int level=-1, const int index=-1, const AccessorData *local_data=0)
types::global_dof_index vertex_dof_index(const unsigned int vertex, const unsigned int i, const unsigned int fe_index=DoFHandlerType::default_fe_index) const
void get_dof_values(const InputVector &values, Vector< number > &local_values) const
void set_dof_values(const Vector< number > &local_values, OutputVector &values) const
void set_dof_index(const unsigned int i, const types::global_dof_index index, const unsigned int fe_index=DoFHandlerType::default_fe_index) const
types::global_dof_index mg_vertex_dof_index(const int level, const unsigned int vertex, const unsigned int i, const unsigned int fe_index=DoFHandlerType::default_fe_index) const
static ::ExceptionBase & ExcVectorNotEmpty()
#define Assert(cond, exc)
void set_dof_indices(const std::vector< types::global_dof_index > &dof_indices)
bool operator!=(const DoFAccessor< dim2, DoFHandlerType2, level_dof_access2 > &) const
static ::ExceptionBase & ExcVectorDoesNotMatch()
static ::ExceptionBase & ExcInvalidObject()
unsigned int n_active_fe_indices() const
#define DeclException0(Exception0)
face_iterator face(const unsigned int i) const
unsigned int nth_active_fe_index(const unsigned int n) const
static const unsigned int space_dimension
DoFHandlerType< 1, spacedim > AccessorData
types::global_dof_index mg_dof_index(const int level, const unsigned int i) const
TriaIterator< DoFCellAccessor< DoFHandlerType, level_dof_access > > neighbor(const unsigned int i) const
unsigned int vertex_index(const unsigned int i) const
DoFCellAccessor(const Triangulation< DoFHandlerType::dimension, DoFHandlerType::space_dimension > *tria, const int level, const int index, const AccessorData *local_data)
void set_mg_dof_index(const int level, const unsigned int i, const types::global_dof_index index) const
const DoFHandlerType & get_dof_handler() const
TriaIterator< DoFCellAccessor< DoFHandlerType, level_dof_access > > periodic_neighbor_child_on_subface(const unsigned int face_no, const unsigned int subface_no) const
void clear_future_fe_index() const
#define DEAL_II_NAMESPACE_CLOSE
typename ::internal::DoFHandlerImplementation::Iterators< DoFHandlerType, level_dof_access >::line_iterator line(const unsigned int i) const
DoFAccessor< structdim, DoFHandlerType, level_dof_access > & operator=(const DoFAccessor< structdim, DoFHandlerType, level_dof_access > &da)=delete
typename TriaAccessorBase< structdim, dim, spacedim >::AccessorData AccessorData
DoFHandlerType< 1, spacedim > * dof_handler
static ::ExceptionBase & ExcCantCompareIterators()
void set_future_fe_index(const unsigned int i) const
void update_cell_dof_indices_cache() const
DoFHandlerType * dof_handler
TriaIterator< DoFCellAccessor< DoFHandlerType, level_dof_access > > child(const unsigned int i) const
bool fe_index_is_active(const unsigned int fe_index) const
const FiniteElement< DoFHandlerType::dimension, DoFHandlerType::space_dimension > & get_fe(const unsigned int fe_index) const