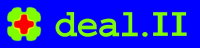 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
17 #ifndef dealii_aligned_vector_h
18 #define dealii_aligned_vector_h
29 #include <boost/version.hpp>
30 #if BOOST_VERSION >= 106400
31 # include <boost/serialization/array_wrapper.hpp>
33 # include <boost/serialization/array.hpp>
35 #include <boost/serialization/split_member.hpp>
38 #include <type_traits>
208 template <
typename ForwardIterator>
233 fill(
const T &element);
318 template <
class Archive>
320 save(Archive &ar,
const unsigned int version)
const;
326 template <
class Archive>
328 load(Archive &ar,
const unsigned int version);
335 template <
class Archive>
337 serialize(Archive &archive,
const unsigned int version);
341 BOOST_SERIALIZATION_SPLIT_MEMBER()
386 template <
typename T>
390 160000 /
sizeof(
T) + 1;
403 const T *
const source_end,
404 T *
const destination)
409 Assert(source_end == source_begin || destination !=
nullptr,
411 const std::size_t size = source_end - source_begin;
424 const std::size_t
end)
const override
438 for (std::size_t i =
begin; i <
end; ++i)
453 template <
typename T>
457 160000 /
sizeof(
T) + 1;
471 T *
const destination)
476 Assert(source_end == source_begin || destination !=
nullptr,
478 const std::size_t size = source_end - source_begin;
491 const std::size_t
end)
const override
505 for (std::size_t i =
begin; i <
end; ++i)
531 template <
typename T,
bool initialize_memory>
535 160000 /
sizeof(
T) + 1;
544 T *
const destination)
559 const unsigned char zero[
sizeof(
T)] = {};
563 if (std::memcmp(
zero,
564 static_cast<const void *
>(&element),
579 const std::size_t
end)
const override
591 begin,
end, std::integral_constant<bool, initialize_memory>());
602 const std::size_t
end,
603 std::integral_constant<bool, false>)
const
605 for (std::size_t i =
begin; i <
end; ++i)
612 const std::size_t
end,
613 std::integral_constant<bool, true>)
const
615 for (std::size_t i =
begin; i <
end; ++i)
633 template <
typename T,
bool initialize_memory>
637 160000 /
sizeof(
T) + 1;
662 const std::size_t
end)
const override
674 begin,
end, std::integral_constant<bool, initialize_memory>());
683 const std::size_t
end,
684 std::integral_constant<bool, false>)
const
686 for (std::size_t i =
begin; i <
end; ++i)
693 const std::size_t
end,
694 std::integral_constant<bool, true>)
const
696 for (std::size_t i =
begin; i <
end; ++i)
709 : data_begin(nullptr)
711 , allocated_end(nullptr)
718 : data_begin(nullptr)
720 , allocated_end(nullptr)
738 : data_begin(nullptr)
740 , allocated_end(nullptr)
743 reserve(vec.data_end - vec.data_begin);
744 data_end = allocated_end;
752 : data_begin(vec.data_begin)
753 , data_end(vec.data_end)
754 , allocated_end(vec.allocated_end)
757 vec.data_end =
nullptr;
758 vec.allocated_end =
nullptr;
768 resize_fast(vec.data_end - vec.data_begin);
781 data_begin = vec.data_begin;
782 data_end = vec.data_end;
783 allocated_end = vec.allocated_end;
785 vec.data_begin =
nullptr;
786 vec.data_end =
nullptr;
787 vec.allocated_end =
nullptr;
804 while (data_end != data_begin + size_in)
808 data_end = data_begin + size_in;
814 size_in - old_size, data_begin + old_size);
829 while (data_end != data_begin + size_in)
833 data_end = data_begin + size_in;
836 if (size_in > old_size)
838 size_in - old_size, data_begin + old_size);
853 while (data_end != data_begin + size_in)
857 data_end = data_begin + size_in;
860 if (size_in > old_size)
863 data_begin + old_size);
872 const size_type old_size = data_end - data_begin;
873 const size_type allocated_size = allocated_end - data_begin;
874 if (size_alloc > allocated_size)
880 if (size_alloc < (2 * allocated_size))
881 new_size = 2 * allocated_size;
883 const size_type size_actual_allocate = new_size *
sizeof(
T);
890 size_actual_allocate);
895 data_end = data_begin + old_size;
896 allocated_end = data_begin + new_size;
897 if (data_end != data_begin)
907 else if (size_alloc == 0)
917 if (data_begin !=
nullptr)
920 while (data_end != data_begin)
925 data_begin =
nullptr;
927 allocated_end =
nullptr;
937 if (data_end == allocated_end)
940 new (data_end++)
T(in_data);
942 *data_end++ = in_data;
952 T *field = data_end - 1;
963 const T *field = data_end - 1;
970 template <
typename ForwardIterator>
974 const unsigned int old_size = size();
1011 std::swap(allocated_end, vec.allocated_end);
1020 return data_end == data_begin;
1029 return data_end - data_begin;
1038 return allocated_end - data_begin;
1048 return data_begin[index];
1058 return data_begin[index];
1063 template <
typename T>
1072 template <
typename T>
1118 template <
class Archive>
1125 ar &boost::serialization::make_array(data_begin, vec_size);
1131 template <
class Archive>
1141 ar &boost::serialization::make_array(data_begin, vec_size);
1142 data_end = data_begin + vec_size;
1153 for (
const T *t = data_begin; t != data_end; ++t)
1155 memory +=
sizeof(
T) * (allocated_end - data_end);
1160 #endif // ifndef DOXYGEN
1172 if (lhs.size() != rhs.size())
bool operator!=(const AlignedVector< T > &lhs, const AlignedVector< T > &rhs)
void serialize(Archive &archive, const unsigned int version)
bool operator==(const AlignedVector< T > &lhs, const AlignedVector< T > &rhs)
virtual void apply_to_subrange(const std::size_t begin, const std::size_t end) const override
size_type capacity() const
void copy_construct_or_assign(const std::size_t begin, const std::size_t end, std::integral_constant< bool, false >) const
#define AssertIndexRange(index, range)
void save(Archive &ar, const unsigned int version) const
void resize(const size_type size_in)
AlignedVectorSet(const std::size_t size, const T &element, T *const destination)
void insert_back(ForwardIterator begin, ForwardIterator end)
const value_type * const_pointer
virtual void apply_to_subrange(const std::size_t begin, const std::size_t end) const override
AlignedVectorDefaultInitialize(const std::size_t size, T *const destination)
static const std::size_t minimum_parallel_grain_size
static const std::size_t minimum_parallel_grain_size
static const std::size_t minimum_parallel_grain_size
static const std::size_t minimum_parallel_grain_size
void load(Archive &ar, const unsigned int version)
AlignedVector & operator=(const AlignedVector< T > &vec)
VectorType::value_type * begin(VectorType &V)
void default_construct_or_assign(const std::size_t begin, const std::size_t end, std::integral_constant< bool, true >) const
#define DEAL_II_NAMESPACE_OPEN
std::enable_if< std::is_fundamental< T >::value, std::size_t >::type memory_consumption(const T &t)
reference operator[](const size_type index)
VectorType::value_type * end(VectorType &V)
void swap(MemorySpaceData< Number, MemorySpace > &, MemorySpaceData< Number, MemorySpace > &)
AlignedVectorMove(T *const source_begin, T *const source_end, T *const destination)
virtual void apply_to_subrange(const std::size_t begin, const std::size_t end) const override
void swap(AlignedVector< T > &vec)
void reserve(const size_type size_alloc)
void default_construct_or_assign(const std::size_t begin, const std::size_t end, std::integral_constant< bool, false >) const
void resize_fast(const size_type size)
const value_type * const_iterator
virtual void apply_to_subrange(const std::size_t begin, const std::size_t end) const override
size_type memory_consumption() const
static ::ExceptionBase & ExcInternalError()
types::global_dof_index size_type
void push_back(const T in_data)
#define Assert(cond, exc)
static const types::blas_int zero
#define DEAL_II_NAMESPACE_CLOSE
void posix_memalign(void **memptr, std::size_t alignment, std::size_t size)
const value_type & const_reference
AlignedVectorCopy(const T *const source_begin, const T *const source_end, T *const destination)
void apply_parallel(const std::size_t begin, const std::size_t end, const std::size_t minimum_parallel_grain_size) const
T max(const T &t, const MPI_Comm &mpi_communicator)
void copy_construct_or_assign(const std::size_t begin, const std::size_t end, std::integral_constant< bool, true >) const