Loading [MathJax]/extensions/TeX/AMSsymbols.js
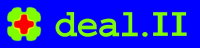 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_cell_id_h
17 #define dealii_cell_id_h
28 #ifdef DEAL_II_WITH_P4EST
145 template <
int dim,
int spacedim>
184 template <
class Archive>
186 serialize(Archive &ar,
const unsigned int version);
216 #ifdef DEAL_II_WITH_P4EST
217 std::array<std::uint8_t, internal::p4est::functions<2>::max_level>
223 friend std::istream &
225 friend std::ostream &
234 inline std::ostream &
243 os <<
static_cast<unsigned char>(
'0' + cid.
child_indices[i]);
252 template <
class Archive>
264 inline std::istream &
313 return !(*
this == other);
324 unsigned int idx = 0;
friend std::istream & operator>>(std::istream &is, CellId &cid)
bool operator!=(const CellId &other) const
friend std::ostream & operator<<(std::ostream &os, const CellId &cid)
bool operator<(const CellId &other) const
bool is_ancestor_of(const CellId &other) const
std::string to_string() const
types::coarse_cell_id coarse_cell_id
static ::ExceptionBase & ExcMessage(std::string arg1)
std::array< std::uint8_t, internal::p4est::functions< 2 >::max_level > child_indices
#define DEAL_II_NAMESPACE_OPEN
types::coarse_cell_id get_coarse_cell_id() const
unsigned int n_child_indices
void serialize(Archive &ar, const unsigned int version)
bool is_parent_of(const CellId &other) const
const types::global_dof_index * dummy()
#define Assert(cond, exc)
OutputOperator< VectorType > & operator<<(OutputOperator< VectorType > &out, unsigned int step)
bool operator==(const CellId &other) const
std::array< unsigned int, 4 > binary_type
#define DEAL_II_NAMESPACE_CLOSE
std::istream & operator>>(std::istream &in, Point< dim, Number > &p)
Triangulation< dim, spacedim >::cell_iterator to_cell(const Triangulation< dim, spacedim > &tria) const
binary_type to_binary() const