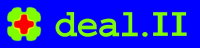 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_thread_management_h
17 # define dealii_thread_management_h
27 # include <condition_variable>
28 # include <functional>
37 # ifdef DEAL_II_WITH_THREADS
39 # ifdef DEAL_II_USE_MT_POSIX
42 # define TBB_SUPPRESS_DEPRECATED_MESSAGES 1
43 # include <tbb/task.h>
44 # undef TBB_SUPPRESS_DEPRECATED_MESSAGES
45 # include <tbb/tbb_stddef.h>
187 condition_variable.notify_one();
197 condition_variable.notify_all();
212 std::unique_lock<std::mutex> lock(mutex, std::adopt_lock);
213 condition_variable.wait(lock);
223 # ifdef DEAL_II_USE_MT_POSIX
249 const char * name =
nullptr,
250 void * arg =
nullptr);
271 # ifndef DEAL_II_USE_MT_POSIX_NO_BARRIERS
366 template <
typename ForwardIterator>
367 std::vector<std::pair<ForwardIterator, ForwardIterator>>
369 const ForwardIterator &
end,
370 const unsigned int n_intervals);
380 std::vector<std::pair<unsigned int, unsigned int>>
382 const unsigned int end,
383 const unsigned int n_intervals);
457 template <
typename ForwardIterator>
458 std::vector<std::pair<ForwardIterator, ForwardIterator>>
460 const ForwardIterator &
end,
461 const unsigned int n_intervals)
463 using IteratorPair = std::pair<ForwardIterator, ForwardIterator>;
469 if (n_intervals == 1)
470 return (std::vector<IteratorPair>(1, IteratorPair(
begin,
end)));
473 const unsigned int n_elements = std::distance(
begin,
end);
474 const unsigned int n_elements_per_interval = n_elements / n_intervals;
475 const unsigned int residual = n_elements % n_intervals;
477 std::vector<IteratorPair> return_values(n_intervals);
479 return_values[0].first =
begin;
480 for (
unsigned int i = 0; i < n_intervals; ++i)
482 if (i != n_intervals - 1)
484 return_values[i].second = return_values[i].first;
490 static_cast<signed int>(n_elements_per_interval));
494 ++return_values[i].second;
496 return_values[i + 1].first = return_values[i].second;
499 return_values[i].second =
end;
501 return return_values;
519 template <
typename RT>
541 value = std::move(v);
555 template <
typename RT>
568 inline reference_type
605 template <
typename RT>
607 call(
const std::function<RT()> &
function,
610 ret_val.
set(
function());
625 # ifdef DEAL_II_WITH_THREADS
637 template <
typename RT>
713 start(
const std::function<RT()> &
function)
716 ret_val = std::make_shared<return_value<RT>>();
760 catch (
const std::exception &exc)
781 template <
typename RT>
782 struct ThreadDescriptor
788 std::shared_ptr<return_value<RT>>
ret_val;
795 start(
const std::function<RT()> &
function)
797 ret_val = std::make_shared<return_value<RT>>();
835 template <
typename RT =
void>
842 Thread(
const std::function<RT()> &
function)
966 template <
typename T>
985 template <
typename T>
988 static std::reference_wrapper<T>
1008 template <
typename RT>
1081 template <
typename FunctionObjectType>
1084 ->
Thread<decltype(function_object())>
1086 using return_type = decltype(function_object());
1098 template <
typename RT,
typename... Args>
1114 template <
typename RT,
typename C,
typename... Args>
1121 return new_thread(std::function<RT()>(std::bind(
1125 # ifndef DEAL_II_CONST_MEMBER_DEDUCTION_BUG
1131 template <
typename RT,
typename C,
typename... Args>
1138 return new_thread(std::function<RT()>(std::bind(
1153 template <
typename RT =
void>
1196 # ifdef DEAL_II_WITH_THREADS
1204 template <
typename RT>
1220 catch (
const std::exception &exc)
1258 template <
typename RT>
1265 std::function<RT()>
function;
1338 friend class ::Threads::Task<RT>;
1343 template <
typename RT>
1345 const std::function<RT()> &
function)
1346 : function(function)
1348 , task_is_done(false)
1352 template <
typename RT>
1358 task =
new (tbb::task::allocate_root()) tbb::empty_task;
1359 task->set_ref_count(2);
1364 tbb::task::spawn(*worker);
1369 template <
typename RT>
1371 : task_is_done(false)
1378 template <
typename RT>
1396 task->destroy(*task);
1400 template <
typename RT>
1413 if (task_is_done ==
true)
1417 task_is_done =
true;
1418 task->wait_for_all();
1423 # else // no threading enabled
1429 template <
typename RT>
1443 call(
function, ret_val);
1479 template <
typename RT =
void>
1490 Task(
const std::function<RT()> &function_object)
1495 std::make_shared<internal::TaskDescriptor<RT>>(function_object);
1496 task_descriptor->queue_task();
1528 task_descriptor->join();
1546 return (task_descriptor !=
1598 return task_descriptor->ret_val.get();
1611 return task_descriptor == t.task_descriptor;
1623 "The current object is not associated with a task that "
1624 "can be joined. It may have been detached, or you "
1625 "may have already joined it in the past.");
1645 template <
typename RT>
1718 template <
typename FunctionObjectType>
1721 ->
Task<decltype(function_object())>
1723 using return_type = decltype(function_object());
1736 template <
typename RT,
typename... Args>
1752 template <
typename RT,
typename C,
typename... Args>
1759 return new_task(std::function<RT()>(std::bind(
1763 # ifndef DEAL_II_CONST_MEMBER_DEDUCTION_BUG
1769 template <
typename RT,
typename C,
typename... Args>
1776 return new_task(std::function<RT()>(std::bind(
1797 template <
typename RT =
void>
1822 return tasks.size();
1835 for (
auto &t : tasks)
#define DeclExceptionMsg(Exception, defaulttext)
unsigned int this_thread_id()
virtual tbb::task * execute() override
reference_type get() const
return_value< RT > ret_val
Task< RT > new_task(RT(C::*fun_ptr)(Args...) const, typename identity< const C >::type &c, typename identity< Args >::type... args)
void handle_unknown_exception()
ThreadGroup & operator+=(const Thread< RT > &t)
TaskEntryPoint(TaskDescriptor< RT > &task_descriptor)
std::shared_ptr< internal::TaskDescriptor< RT > > task_descriptor
bool operator==(const Task &t) const
Thread(const Thread< RT > &t)
void start(const std::function< RT()> &function)
SymmetricTensor< 2, dim, Number > C(const Tensor< 2, dim, Number > &F)
std::condition_variable condition_variable
TaskDescriptor< RT > & task_descriptor
Mutex thread_is_active_mutex
TaskGroup & operator+=(const Task< RT > &t)
internal::return_value< RT >::reference_type return_value()
static void thread_entry_point(const std::function< RT()> &function, std::shared_ptr< return_value< RT >> ret_val)
std::vector< std::pair< unsigned int, unsigned int > > split_interval(const unsigned int begin, const unsigned int end, const unsigned int n_intervals)
void call(const std::function< void()> &function, internal::return_value< void > &)
#define DEAL_II_ENABLE_EXTRA_DIAGNOSTICS
std::lock_guard< std::mutex > ScopedLock
Task(const std::function< RT()> &function_object)
VectorType::value_type * begin(VectorType &V)
#define DEAL_II_NAMESPACE_OPEN
std::list< Thread< RT > > threads
auto apply(F &&fn, Tuple &&t) -> decltype(apply_impl(std::forward< F >(fn), std::forward< Tuple >(t), std_cxx14::make_index_sequence< std::tuple_size< typename std::remove_reference< Tuple >::type >::value >()))
void call(const std::function< RT()> &function, internal::return_value< RT > &ret_val)
VectorType::value_type * end(VectorType &V)
#define DEAL_II_DEPRECATED
std::list< Task< RT > > tasks
bool operator==(const Thread &t) const
internal::return_value< RT >::reference_type return_value()
unsigned int n_existing_threads()
static std::reference_wrapper< T > act(T &t)
Thread(const std::function< RT()> &function)
void handle_std_exception(const std::exception &exc)
void advance(std::tuple< I1, I2 > &t, const unsigned int n)
Thread< RT > new_thread(const std::function< RT()> &function)
const types::global_dof_index * dummy()
static ::ExceptionBase & ExcInternalError()
std::shared_ptr< return_value< RT > > ret_val
#define Assert(cond, exc)
Mutex & operator=(const Mutex &)
static void initialize_multithreading()
TaskDescriptor & operator=(const TaskDescriptor &)=delete
#define AssertNothrow(cond, exc)
pthread_barrier_t barrier
std::vector< std::pair< ForwardIterator, ForwardIterator > > split_range(const ForwardIterator &begin, const ForwardIterator &end, const unsigned int n_intervals)
#define DEAL_II_NAMESPACE_CLOSE
#define AssertThrow(cond, exc)
#define DEAL_II_DISABLE_EXTRA_DIAGNOSTICS
std::shared_ptr< internal::ThreadDescriptor< RT > > thread_descriptor