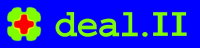 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_differentiation_sd_symengine_number_types_h
17 #define dealii_differentiation_sd_symengine_number_types_h
21 #ifdef DEAL_II_WITH_SYMENGINE
24 # include <symengine/basic.h>
25 # include <symengine/dict.h>
26 # include <symengine/symengine_exception.h>
27 # include <symengine/symengine_rcp.h>
30 # include <symengine/expression.h>
31 # include <symengine/integer.h>
32 # include <symengine/logic.h>
33 # include <symengine/number.h>
34 # include <symengine/rational.h>
37 # include <symengine/add.h>
38 # include <symengine/functions.h>
39 # include <symengine/mul.h>
40 # include <symengine/pow.h>
43 # include <symengine/eval.h>
44 # include <symengine/eval_arb.h>
45 # include <symengine/eval_double.h>
54 # include <boost/serialization/split_member.hpp>
56 # include <symengine/derivative.h>
61 # include <type_traits>
83 <<
"The string '" << arg1
84 <<
"' could not be parsed successfully. Are you sure that (1) it "
85 <<
"consists of legitimate operations and syntax, and (2) you've "
86 <<
"previously declared all symbolic variables that are present "
87 <<
"in the expression?");
208 template <
typename NumberType,
209 typename =
typename std::enable_if<
220 template <
typename NumberType,
221 typename =
typename std::enable_if<
236 template <
typename NumberType,
237 typename =
typename std::enable_if<
239 Expression(
const NumberType &numerator,
const NumberType &denominator);
307 Expression(
const std::vector<std::pair<Expression, Expression>>
308 & condition_expression,
330 Expression(
const std::vector<std::pair<Expression, Expression>>
331 &condition_expression);
355 const bool parse_as_expression =
false);
378 explicit Expression(
const SymEngine::Expression &rhs);
388 Expression(
const SymEngine::RCP<const SymEngine::Basic> &rhs);
403 Expression(SymEngine::RCP<const SymEngine::Basic> &&rhs);
432 print(std::ostream &stream)
const;
440 save(std::ostream &stream)
const;
453 load(std::istream &stream);
462 template <
class Archive>
464 save(Archive &archive,
const unsigned int version)
const;
478 template <
class Archive>
480 load(Archive &archive,
const unsigned int version);
496 template <
class Archive>
498 serialize(Archive &archive,
const unsigned int version);
502 BOOST_SERIALIZATION_SPLIT_MEMBER()
515 const SymEngine::Expression &
522 const SymEngine::Basic &
529 const SymEngine::RCP<const SymEngine::Basic> &
606 template <
typename NumberType>
627 template <
typename NumberType>
637 template <
typename NumberType>
647 template <
typename NumberType>
657 template <
typename NumberType>
681 const SymEngine::RCP<const SymEngine::Symbol> &symbol)
const;
688 differentiate(
const SymEngine::RCP<const SymEngine::Basic> &symbol)
const;
729 substitute(
const SymEngine::map_basic_basic &substitution_values)
const;
752 template <
typename NumberType>
763 template <
typename ReturnType>
779 template <
typename ReturnType>
782 const SymEngine::map_basic_basic &substitution_values)
const;
841 template <
typename ResultType>
842 explicit operator ResultType()
const;
848 explicit operator const SymEngine::Expression &()
const;
854 operator const SymEngine::RCP<const SymEngine::Basic> &()
const;
862 SymEngine::Expression &
1086 template <
typename NumberType,
1087 typename =
typename std::enable_if<
1103 template <
typename NumberType,
1104 typename =
typename std::enable_if<
1120 template <
typename NumberType,
1121 typename =
typename std::enable_if<
1137 template <
typename NumberType,
1138 typename =
typename std::enable_if<
1154 template <
typename NumberType,
1155 typename =
typename std::enable_if<
1170 template <
typename NumberType,
1171 typename =
typename std::enable_if<
1186 template <
typename NumberType,
1187 typename =
typename std::enable_if<
1203 template <
typename NumberType,
1204 typename =
typename std::enable_if<
1228 template <
typename NumberType,
typename>
1234 template <
typename NumberType,
typename>
1235 Expression::Expression(
const std::complex<NumberType> &
value)
1240 template <
typename NumberType,
typename>
1241 Expression::Expression(
const NumberType &numerator,
1242 const NumberType &denominator)
1244 SymEngine::Rational::from_two_ints(*SymEngine::integer(numerator),
1245 *SymEngine::integer(denominator)))
1249 template <
class Archive>
1251 Expression::save(Archive &ar,
const unsigned int )
const
1253 std::stringstream sstream;
1255 const std::string expr = sstream.str();
1260 template <
class Archive>
1262 Expression::load(Archive &ar,
const unsigned int )
1270 template <
typename NumberType>
1273 const NumberType &
value)
const
1275 Assert(SymEngine::is_a<SymEngine::Symbol>(symbol.get_value()),
1277 "Substitution with a number that does not represent a symbol."));
1280 sub_vals[symbol] = Expression(
value);
1285 template <
typename ReturnType>
1290 return static_cast<ReturnType
>(
substitute(substitution_values));
1294 template <
typename ReturnType>
1297 const SymEngine::map_basic_basic &substitution_values)
const
1299 return static_cast<ReturnType
>(
substitute(substitution_values));
1303 template <
typename NumberType>
1305 Expression::operator=(
const NumberType &rhs)
1307 *
this = Expression(rhs);
1312 template <
typename NumberType>
1314 Expression::operator+=(
const NumberType &rhs)
1316 *
this = Expression(SymEngine::add(get_RCP(), Expression(rhs).get_RCP()));
1321 template <
typename NumberType>
1323 Expression::operator-=(
const NumberType &rhs)
1325 *
this = Expression(SymEngine::sub(get_RCP(), Expression(rhs).get_RCP()));
1330 template <
typename NumberType>
1332 Expression::operator*=(
const NumberType &rhs)
1334 *
this = Expression(SymEngine::mul(get_RCP(), Expression(rhs).get_RCP()));
1339 template <
typename NumberType>
1341 Expression::operator/=(
const NumberType &rhs)
1343 *
this = Expression(SymEngine::div(get_RCP(), Expression(rhs).get_RCP()));
1348 template <
typename ResultType>
1349 Expression::operator ResultType()
const
1351 return static_cast<ResultType
>(get_expression());
1363 template <
int rank_,
int dim,
typename Number>
1399 value(
const std::complex<T> &t)
1404 template <
typename T,
int dim>
1411 template <
typename T,
int dim>
1427 if (SymEngine::is_a_Number(
value.get_value()))
1429 const SymEngine::RCP<const SymEngine::Number> number_rcp =
1430 SymEngine::rcp_static_cast<const SymEngine::Number>(
value.get_RCP());
1431 return number_rcp->is_zero();
1459 #endif // DEAL_II_WITH_SYMENGINE
1461 #endif // dealii_differentiation_sd_symengine_number_types_h
Expression operator&&(const Expression &lhs, const Expression &rhs)
Expression operator!(const Expression &expression)
void save(std::ostream &stream) const
Expression & parse(const std::string &expression)
Expression & operator/=(const Expression &rhs)
Expression operator==(const Expression &lhs, const Expression &rhs)
std::istream & operator>>(std::istream &stream, Expression &expression)
const SymEngine::Expression & get_expression() const
std::ostream & print(std::ostream &stream) const
static constexpr const T & value(const T &t)
Expression operator|(const Expression &lhs, const Expression &rhs)
Expression operator+(Expression lhs, const Expression &rhs)
Expression substitute(const types::substitution_map &substitution_values) const
std::vector< SD::Expression > symbol_vector
std::ostream & operator<<(std::ostream &stream, const Expression &expression)
Expression & operator*=(const Expression &rhs)
std::map< SD::Expression, SD::Expression, internal::ExpressionKeyLess > substitution_map
constexpr bool values_are_equal(const Number1 &value_1, const Number2 &value_2)
static ::ExceptionBase & ExcSymEngineParserError(std::string arg1)
const SymEngine::Basic & get_value() const
SymEngine::Expression expression
void load(std::istream &stream)
Expression operator>(const Expression &lhs, const Expression &rhs)
const SymEngine::RCP< const SymEngine::Basic > & get_RCP() const
void serialize(Archive &archive, const unsigned int version)
Expression operator/(Expression lhs, const Expression &rhs)
static ::ExceptionBase & ExcMessage(std::string arg1)
Expression operator<(const Expression &lhs, const Expression &rhs)
Expression operator||(const Expression &lhs, const Expression &rhs)
#define DEAL_II_NAMESPACE_OPEN
Expression & operator+=(const Expression &rhs)
Expression operator!=(const Expression &lhs, const Expression &rhs)
#define DeclException1(Exception1, type1, outsequence)
Expression operator<=(const Expression &lhs, const Expression &rhs)
Expression & operator=(const Expression &rhs)
Expression substitute(const Expression &expression, const types::substitution_map &substitution_map)
Expression & operator-=(const Expression &rhs)
Expression operator^(const Expression &lhs, const Expression &rhs)
Expression operator-() const
Expression operator>=(const Expression &lhs, const Expression &rhs)
#define Assert(cond, exc)
ReturnType substitute_and_evaluate(const types::substitution_map &substitution_values) const
Expression operator-(Expression lhs, const Expression &rhs)
virtual ~Expression()=default
Expression operator&(const Expression &lhs, const Expression &rhs)
Expression differentiate(const Expression &symbol) const
#define DEAL_II_NAMESPACE_CLOSE
ValueType substitute_and_evaluate(const Expression &expression, const types::substitution_map &substitution_map)
constexpr bool value_is_zero(const Number &value)
bool value_is_less_than(const Number1 &value_1, const Number2 &value_2)
Expression operator*(Expression lhs, const Expression &rhs)