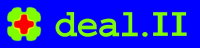 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_sparsity_pattern_h
17 #define dealii_sparsity_pattern_h
30 #include <boost/version.hpp>
31 #if BOOST_VERSION >= 106400
32 # include <boost/serialization/array_wrapper.hpp>
34 # include <boost/serialization/array.hpp>
36 #include <boost/serialization/split_member.hpp>
50 template <
typename number>
52 template <
typename number>
54 template <
typename number>
56 template <
typename number>
71 namespace SparsityPatternTools
91 template <
typename value>
100 template <
typename value>
223 "The instance of this class was initialized"
224 " without SparsityPattern object, which"
225 " means that it is a dummy accessor that can"
226 " not do any operations.");
411 const std::vector<unsigned int> &row_lengths);
623 std::pair<size_type, size_type>
639 print(std::ostream &out)
const;
671 template <
class Archive>
673 save(Archive &ar,
const unsigned int version)
const;
679 template <
class Archive>
681 load(Archive &ar,
const unsigned int version);
688 template <
class Archive>
690 serialize(Archive &archive,
const unsigned int version);
694 BOOST_SERIALIZATION_SPLIT_MEMBER()
710 "The operation you attempted is only allowed after the SparsityPattern "
711 "has been set up and compress() was called.");
719 <<
"Upon entering a new entry to row " << arg1
720 <<
": there was no free entry any more. " << std::endl
721 <<
"(Maximum number of entries for this row: " << arg2
722 <<
"; maybe the matrix is already compressed?)");
730 "The operation you attempted changes the structure of the SparsityPattern "
731 "and is not possible after compress() has been called.");
817 template <
typename number>
819 template <
typename number>
821 template <
typename number>
823 template <
typename number>
935 const unsigned int max_per_row);
948 const std::vector<unsigned int> &row_lengths);
968 const std::vector<unsigned int> &row_lengths);
993 const unsigned int max_per_row,
1111 template <
typename ForwardIterator>
1115 const ForwardIterator
begin,
1116 const ForwardIterator
end);
1139 template <
typename number>
1149 template <
typename ForwardIterator>
1152 ForwardIterator
begin,
1153 ForwardIterator
end,
1154 const bool indices_are_sorted =
false);
1260 template <
class Archive>
1262 save(Archive &ar,
const unsigned int version)
const;
1268 template <
class Archive>
1270 load(Archive &ar,
const unsigned int version);
1277 template <
class Archive>
1279 serialize(Archive &archive,
const unsigned int version);
1283 BOOST_SERIALIZATION_SPLIT_MEMBER()
1298 <<
"The iterators denote a range of " << arg1
1299 <<
" elements, but the given number of rows was " << arg2);
1305 <<
"The number of partitions you gave is " << arg1
1306 <<
", but must be greater than zero.");
1315 template <
typename number>
1317 template <
typename number>
1319 template <
typename number>
1321 template <
typename number>
1343 const std::size_t i)
1344 : container(sparsity_pattern)
1351 : container(sparsity_pattern)
1352 , linear_index(container->rowstart[container->rows])
1358 : container(nullptr)
1365 Accessor::is_valid_entry()
const
1367 Assert(container !=
nullptr, DummyAccessor());
1368 return (linear_index < container->rowstart[container->rows] &&
1375 Accessor::row()
const
1379 const std::size_t *insert_point =
1380 std::upper_bound(container->rowstart.get(),
1381 container->rowstart.get() + container->rows + 1,
1383 return insert_point - container->rowstart.get() - 1;
1389 Accessor::column()
const
1393 return (container->colnums[linear_index]);
1399 Accessor::index()
const
1403 return linear_index - container->rowstart[row()];
1413 return linear_index;
1421 Assert(container !=
nullptr, DummyAccessor());
1422 Assert(other.container !=
nullptr, DummyAccessor());
1423 return (container == other.container && linear_index == other.linear_index);
1431 Assert(container !=
nullptr, DummyAccessor());
1432 Assert(other.container !=
nullptr, DummyAccessor());
1435 return linear_index < other.linear_index;
1443 Assert(container !=
nullptr, DummyAccessor());
1444 Assert(linear_index < container->rowstart[container->rows],
1451 const std::size_t linear_index)
1482 return {
nullptr, 0};
1550 const unsigned int index)
const
1574 template <
class Archive>
1579 ar &boost::serialization::base_object<const Subscriptor>(*
this);
1589 template <
class Archive>
1594 ar &boost::serialization::base_object<Subscriptor>(*
this);
1607 template <
class Archive>
1612 ar &boost::serialization::base_object<const SparsityPatternBase>(*
this);
1618 template <
class Archive>
1623 ar &boost::serialization::base_object<SparsityPatternBase>(*
this);
1663 namespace SparsityPatternTools
1678 template <
typename value>
1687 template <
typename value>
1698 template <
typename ForwardIterator>
1702 const ForwardIterator
begin,
1703 const ForwardIterator
end)
1713 std::vector<unsigned int> row_lengths;
1714 row_lengths.reserve(
n_rows);
1715 for (ForwardIterator i =
begin; i !=
end; ++i)
1716 row_lengths.push_back(std::distance(i->begin(), i->end()) +
1717 (is_square ? 1 : 0));
1726 using inner_iterator =
1727 typename std::iterator_traits<ForwardIterator>::value_type::const_iterator;
1728 for (ForwardIterator i =
begin; i !=
end; ++i, ++row)
1731 const inner_iterator end_of_row = i->end();
1732 for (inner_iterator j = i->begin(); j != end_of_row; ++j)
1738 if ((col != row) || !is_square)
unsigned int row_length(const size_type row) const
#define DeclExceptionMsg(Exception, defaulttext)
void serialize(Archive &archive, const unsigned int version)
unsigned int max_row_length
bool stores_only_added_elements() const
bool operator==(const Accessor &) const
void save(Archive &ar, const unsigned int version) const
std::size_t memory_consumption() const
bool operator<(const SynchronousIterators< Iterators > &a, const SynchronousIterators< Iterators > &b)
void print_svg(std::ostream &out) const
bool operator==(const AlignedVector< T > &lhs, const AlignedVector< T > &rhs)
MatrixTableIterators::Accessor< TransposeTable< T >, Constness, MatrixTableIterators::Storage::column_major > Accessor
void block_read(std::istream &in)
static ::ExceptionBase & ExcInvalidNumberOfPartitions(int arg1)
void add(const size_type i, const size_type j)
static ::ExceptionBase & DummyAccessor()
void load(Archive &ar, const unsigned int version)
SparsityPatternIterators::Iterator const_iterator
~SparsityPatternBase() override=default
#define AssertIndexRange(index, range)
SparsityPatternIterators::Iterator iterator
size_type max_entries_per_row() const
std::unique_ptr< size_type[]> colnums
void serialize(Archive &archive, const unsigned int version)
bool operator==(const SparsityPattern &) const
static ::ExceptionBase & ExcInvalidIterator()
std::size_t n_nonzero_elements() const
size_type global_index() const
bool store_diagonal_first_in_row
static ::ExceptionBase & ExcMatrixIsCompressed()
std::size_t memory_consumption() const
size_type get_column_index_from_iterator(const size_type i)
size_type operator()(const size_type i, const size_type j) const
static ::ExceptionBase & ExcNotCompressed()
MatrixTableIterators::Iterator< TransposeTable< T >, Constness, MatrixTableIterators::Storage::column_major > Iterator
static const size_type invalid_entry
bool operator<(const Accessor &) const
bool exists(const size_type i, const size_type j) const
~SparsityPattern() override=default
Iterator(const SparsityPatternBase *sp, const std::size_t linear_index)
unsigned int global_dof_index
VectorType::value_type * begin(VectorType &V)
#define DEAL_II_NAMESPACE_OPEN
const SparsityPatternBase * container
void reinit(const size_type m, const size_type n, const unsigned int max_per_row)
@ matrix
Contents is actually a matrix.
VectorType::value_type * end(VectorType &V)
#define DeclException1(Exception1, type1, outsequence)
size_type column_number(const size_type row, const unsigned int index) const
void block_write(std::ostream &out) const
void load(Archive &ar, const unsigned int version)
void print(std::ostream &out) const
bool operator==(const SparsityPatternBase &) const
void advance(std::tuple< I1, I2 > &t, const unsigned int n)
std::pair< size_type, size_type > matrix_position(const std::size_t global_index) const
static ::ExceptionBase & ExcInternalError()
#define Assert(cond, exc)
void print_gnuplot(std::ostream &out) const
types::global_dof_index size_type
const types::global_dof_index invalid_size_type
SparsityPattern & operator=(const SparsityPattern &)
types::global_dof_index size_type
size_type bandwidth() const
static ::ExceptionBase & ExcIteratorRange(int arg1, int arg2)
size_type row_position(const size_type i, const size_type j) const
bool is_compressed() const
void add_entries(const size_type row, ForwardIterator begin, ForwardIterator end, const bool indices_are_sorted=false)
virtual void reinit(const size_type m, const size_type n, const ArrayView< const unsigned int > &row_lengths) override
std::unique_ptr< std::size_t[]> rowstart
#define DEAL_II_NAMESPACE_CLOSE
size_type get_column_index_from_iterator(const std::pair< const size_type, value > &i)
void copy_from(const size_type n_rows, const size_type n_cols, const ForwardIterator begin, const ForwardIterator end)
void save(Archive &ar, const unsigned int version) const
TrilinosWrappers::types::int_type global_index(const Epetra_BlockMap &map, const ::types::global_dof_index i)
#define DeclException2(Exception2, type1, type2, outsequence)
SparsityPatternBase::size_type size_type
types::global_dof_index size_type
static ::ExceptionBase & ExcNotEnoughSpace(int arg1, int arg2)
static ::ExceptionBase & ExcIteratorPastEnd()
bool is_valid_entry() const