Loading [MathJax]/extensions/TeX/newcommand.js
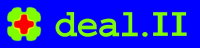 |
Reference documentation for deal.II version 9.2.0
|
\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}
\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}
\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}
\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}
Go to the documentation of this file.
16 #ifndef dealii_chunk_sparsity_pattern_h
17 #define dealii_chunk_sparsity_pattern_h
329 const std::vector<size_type> &row_lengths,
352 const std::vector<size_type> &row_lengths,
399 const std::vector<size_type> &row_lengths,
501 template <
typename ForwardIterator>
505 const ForwardIterator
begin,
506 const ForwardIterator
end,
514 template <
typename SparsityPatternType>
525 template <
typename number>
546 template <
typename Sparsity>
550 const Sparsity &sparsity_pattern_for_chunks,
552 const bool optimize_diagonal =
true);
732 print(std::ostream &out)
const;
766 <<
"The provided number is invalid here: " << arg1);
773 <<
"The given index " << arg1 <<
" should be less than "
781 <<
"Upon entering a new entry to row " << arg1
782 <<
": there was no free entry any more. " << std::endl
783 <<
"(Maximum number of entries for this row: " << arg2
784 <<
"; maybe the matrix is already compressed?)");
791 "The operation you attempted is only allowed after the SparsityPattern "
792 "has been set up and compress() was called.");
799 "The operation you attempted changes the structure of the SparsityPattern "
800 "and is not possible after compress() has been called.");
811 <<
"The iterators denote a range of " << arg1
812 <<
" elements, but the given number of rows was " << arg2);
822 <<
"The number of partitions you gave is " << arg1
823 <<
", but must be greater than zero.");
830 <<
"The array has size " << arg1 <<
" but should have size "
873 : sparsity_pattern(sparsity_pattern)
874 , reduced_accessor(row == sparsity_pattern->n_rows() ?
875 *sparsity_pattern->sparsity_pattern.
end() :
876 *sparsity_pattern->sparsity_pattern.
begin(
877 row / sparsity_pattern->get_chunk_size()))
878 , chunk_row(row == sparsity_pattern->n_rows() ?
880 row % sparsity_pattern->get_chunk_size())
887 : sparsity_pattern(sparsity_pattern)
888 , reduced_accessor(*sparsity_pattern->sparsity_pattern.
end())
896 Accessor::is_valid_entry()
const
898 return reduced_accessor.is_valid_entry() &&
901 sparsity_pattern->n_rows() &&
902 sparsity_pattern->get_chunk_size() * reduced_accessor.column() +
904 sparsity_pattern->n_cols();
910 Accessor::row()
const
914 return sparsity_pattern->get_chunk_size() * reduced_accessor.row() +
921 Accessor::column()
const
925 return sparsity_pattern->get_chunk_size() * reduced_accessor.column() +
932 Accessor::reduced_index()
const
936 return reduced_accessor.linear_index;
947 return (reduced_accessor == other.reduced_accessor &&
948 chunk_row == other.chunk_row && chunk_col == other.chunk_col);
958 if (chunk_row != other.chunk_row)
960 if (reduced_accessor.linear_index ==
961 reduced_accessor.container->n_nonzero_elements())
963 if (other.reduced_accessor.linear_index ==
964 reduced_accessor.container->n_nonzero_elements())
967 const auto global_row = sparsity_pattern->get_chunk_size() *
968 reduced_accessor.row() +
970 other_global_row = sparsity_pattern->get_chunk_size() *
971 other.reduced_accessor.row() +
973 if (global_row < other_global_row)
975 else if (global_row > other_global_row)
980 reduced_accessor.linear_index < other.reduced_accessor.linear_index ||
981 (reduced_accessor.linear_index == other.reduced_accessor.linear_index &&
982 chunk_col < other.chunk_col));
989 const auto chunk_size = sparsity_pattern->get_chunk_size();
993 sparsity_pattern->n_rows() &&
994 reduced_accessor.column() *
chunk_size + chunk_col <
995 sparsity_pattern->n_cols(),
999 reduced_accessor.advance();
1007 reduced_accessor.column() *
chunk_size + chunk_col ==
1008 sparsity_pattern->n_cols())
1010 const auto reduced_row = reduced_accessor.row();
1012 if (reduced_accessor.linear_index + 1 ==
1013 reduced_accessor.container->rowstart[reduced_row + 1])
1022 sparsity_pattern->n_rows()))
1025 reduced_accessor.advance();
1030 reduced_accessor.linear_index =
1031 reduced_accessor.container->rowstart[reduced_row];
1036 reduced_accessor.advance();
1046 : accessor(sparsity_pattern, row)
1077 inline const Accessor *Iterator::operator->()
const
1086 return (accessor == other.accessor);
1094 return !(accessor == other.accessor);
1101 return accessor < other.accessor;
1136 return {
this, r + 1};
1172 template <
typename ForwardIterator>
1176 const ForwardIterator
begin,
1177 const ForwardIterator
end,
1188 std::vector<size_type> row_lengths;
1189 row_lengths.reserve(
n_rows);
1190 for (ForwardIterator i =
begin; i !=
end; ++i)
1191 row_lengths.push_back(std::distance(i->begin(), i->end()) +
1192 (is_square ? 1 : 0));
1197 using inner_iterator =
1198 typename std::iterator_traits<ForwardIterator>::value_type::const_iterator;
1199 for (ForwardIterator i =
begin; i !=
end; ++i, ++row)
1201 const inner_iterator end_of_row = i->end();
1202 for (inner_iterator j = i->begin(); j != end_of_row; ++j)
#define DeclExceptionMsg(Exception, defaulttext)
bool operator!=(const AlignedVector< T > &lhs, const AlignedVector< T > &rhs)
SynchronousIterators< Iterators > operator++(SynchronousIterators< Iterators > &a)
bool operator<(const SynchronousIterators< Iterators > &a, const SynchronousIterators< Iterators > &b)
static ::ExceptionBase & ExcNotEnoughSpace(size_type arg1, size_type arg2)
bool operator==(const AlignedVector< T > &lhs, const AlignedVector< T > &rhs)
void reinit(const size_type m, const size_type n, const size_type max_per_row, const size_type chunk_size)
MatrixTableIterators::Accessor< TransposeTable< T >, Constness, MatrixTableIterators::Storage::column_major > Accessor
static ::ExceptionBase & ExcMatrixIsCompressed()
bool operator!=(const Iterator &) const
#define AssertIndexRange(index, range)
bool operator<(const Accessor &) const
static const size_type invalid_entry
bool operator==(const Accessor &) const
std::size_t memory_consumption() const
~ChunkSparsityPattern() override=default
static ::ExceptionBase & ExcInvalidIterator()
size_type get_chunk_size() const
ChunkSparsityPattern & operator=(const ChunkSparsityPattern &)
static ::ExceptionBase & ExcInvalidNumber(size_type arg1)
static ::ExceptionBase & ExcEmptyObject()
bool operator==(const Iterator &) const
bool stores_only_added_elements() const
static ::ExceptionBase & ExcIteratorRange(size_type arg1, size_type arg2)
const Accessor & operator*() const
size_type get_column_index_from_iterator(const size_type i)
MatrixTableIterators::Iterator< TransposeTable< T >, Constness, MatrixTableIterators::Storage::column_major > Iterator
size_type bandwidth() const
static ::ExceptionBase & ExcInvalidNumberOfPartitions(size_type arg1)
static const size_type invalid_entry
bool operator<(const Iterator &) const
unsigned int global_dof_index
VectorType::value_type * begin(VectorType &V)
const ChunkSparsityPattern * sparsity_pattern
void create_from(const size_type m, const size_type n, const Sparsity &sparsity_pattern_for_chunks, const size_type chunk_size, const bool optimize_diagonal=true)
static ::ExceptionBase & ExcInvalidIndex(size_type arg1, size_type arg2)
#define DEAL_II_NAMESPACE_OPEN
void block_read(std::istream &in)
SparsityPattern sparsity_pattern
@ matrix
Contents is actually a matrix.
SparsityPatternIterators::Accessor reduced_accessor
VectorType::value_type * end(VectorType &V)
#define DeclException1(Exception1, type1, outsequence)
void block_write(std::ostream &out) const
void print(std::ostream &out) const
size_type max_entries_per_row() const
static ::ExceptionBase & ExcInvalidArraySize(size_type arg1, size_type arg2)
Iterator(const ChunkSparsityPattern *sp, const size_type row)
void copy_from(const size_type n_rows, const size_type n_cols, const ForwardIterator begin, const ForwardIterator end, const size_type chunk_size)
std::size_t reduced_index() const
void advance(std::tuple< I1, I2 > &t, const unsigned int n)
static ::ExceptionBase & ExcInternalError()
types::global_dof_index size_type
#define Assert(cond, exc)
size_type n_nonzero_elements() const
#define DeclException0(Exception0)
bool is_valid_entry() const
bool exists(const size_type i, const size_type j) const
size_type row_length(const size_type row) const
const Accessor * operator->() const
void add(const size_type i, const size_type j)
#define DEAL_II_NAMESPACE_CLOSE
static ::ExceptionBase & ExcNotCompressed()
static ::ExceptionBase & ExcMETISNotInstalled()
#define DeclException2(Exception2, type1, type2, outsequence)
bool is_compressed() const
types::global_dof_index size_type
Accessor(const ChunkSparsityPattern *matrix, const size_type row)
static ::ExceptionBase & ExcIteratorPastEnd()
std::enable_if< std::is_floating_point< T >::value &&std::is_floating_point< U >::value, typename ProductType< std::complex< T >, std::complex< U > >::type >::type operator*(const std::complex< T > &left, const std::complex< U > &right)
void print_gnuplot(std::ostream &out) const