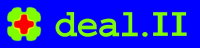 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_sparse_direct_h
17 #define dealii_sparse_direct_h
30 #ifdef DEAL_II_WITH_UMFPACK
42 #ifdef SuiteSparse_long
148 template <
class Matrix>
155 template <
class Matrix>
259 solve(
Vector<std::complex<double>> &rhs_and_solution,
282 template <
class Matrix>
291 template <
class Matrix>
294 Vector<std::complex<double>> &rhs_and_solution,
300 template <
class Matrix>
309 template <
class Matrix>
312 BlockVector<std::complex<double>> &rhs_and_solution,
328 <<
"UMFPACK routine " << arg1 <<
" returned error status " << arg2 <<
"."
330 << (
"A complete list of error codes can be found in the file "
331 "<bundled/umfpack/UMFPACK/Include/umfpack.h>."
333 "That said, the two most common errors that can happen are "
334 "that your matrix cannot be factorized because it is "
335 "rank deficient, and that UMFPACK runs out of memory "
336 "because your problem is too large."
338 "The first of these cases most often happens if you "
339 "forget terms in your bilinear form necessary to ensure "
340 "that the matrix has full rank, or if your equation has a "
341 "spatially variable coefficient (or nonlinearity) that is "
342 "supposed to be strictly positive but, for whatever "
343 "reasons, is negative or zero. In either case, you probably "
344 "want to check your assembly procedure. Similarly, a "
345 "matrix can be rank deficient if you forgot to apply the "
346 "appropriate boundary conditions. For example, the "
347 "Laplace equation for a problem where only Neumann boundary "
348 "conditions are posed (or where you forget to apply Dirichlet "
349 "boundary conditions) has exactly one eigenvalue equal to zero "
350 "and its rank is therefore deficient by one. Finally, the matrix "
351 "may be rank deficient because you are using a quadrature "
352 "formula with too few quadrature points."
354 "The other common situation is that you run out of memory. "
355 "On a typical laptop or desktop, it should easily be possible "
356 "to solve problems with 100,000 unknowns in 2d. If you are "
357 "solving problems with many more unknowns than that, in "
358 "particular if you are in 3d, then you may be running out "
359 "of memory and you will need to consider iterative "
360 "solvers instead of the direct solver employed by "
395 template <
typename number>
399 template <
typename number>
403 template <
typename number>
418 std::vector<types::suitesparse_index>
Ap;
419 std::vector<types::suitesparse_index>
Ai;
420 std::vector<double>
Ax;
421 std::vector<double>
Az;
431 #endif // dealii_sparse_direct_h
void initialize(const SparsityPattern &sparsity_pattern)
~SparseDirectUMFPACK() override
void sort_arrays(const SparseMatrixEZ< number > &)
void * numeric_decomposition
std::vector< types::suitesparse_index > Ai
void factorize(const Matrix &matrix)
unsigned int global_dof_index
static ::ExceptionBase & ExcUMFPACKError(std::string arg1, int arg2)
#define DEAL_II_NAMESPACE_OPEN
@ matrix
Contents is actually a matrix.
void vmult(Vector< double > &dst, const Vector< double > &src) const
void Tvmult(Vector< double > &dst, const Vector< double > &src) const
std::vector< types::suitesparse_index > Ap
void * symbolic_decomposition
void solve(Vector< double > &rhs_and_solution, const bool transpose=false) const
long int suitesparse_index
std::vector< double > control
#define DEAL_II_NAMESPACE_CLOSE
#define DeclException2(Exception2, type1, type2, outsequence)