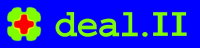 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_mg_constrained_dofs_h
17 #define dealii_mg_constrained_dofs_h
34 template <
int dim,
int spacedim>
68 template <
int dim,
int spacedim>
82 template <
int dim,
int spacedim>
99 template <
int dim,
int spacedim>
103 const std::set<types::boundary_id> &boundary_ids,
138 template <
int dim,
int spacedim>
142 const unsigned int first_vector_component);
252 template <
int dim,
int spacedim>
267 for (
unsigned int l = 0;
l < nlevels; ++
l)
277 for (; cell != endc; ++cell)
281 if (cell->has_periodic_neighbor(f) &&
282 cell->periodic_neighbor(f)->level() == cell->level())
284 if (cell->is_locally_owned_on_level())
287 cell->periodic_neighbor(f)->level_subdomain_id() !=
290 "Periodic neighbor of a locally owned cell must either be owned or ghost."));
294 else if (cell->periodic_neighbor(f)->level_subdomain_id() ==
297 Assert(cell->is_locally_owned_on_level() ==
false,
302 const unsigned int dofs_per_face =
303 cell->face(f)->
get_fe(0).dofs_per_face;
304 std::vector<types::global_dof_index> dofs_1(dofs_per_face);
305 std::vector<types::global_dof_index> dofs_2(dofs_per_face);
307 cell->periodic_neighbor(f)
308 ->face(cell->periodic_neighbor_face_no(f))
309 ->get_mg_dof_indices(
l, dofs_1, 0);
310 cell->face(f)->get_mg_dof_indices(
l, dofs_2, 0);
316 for (
unsigned int i = 0; i < dofs_per_face; ++i)
339 template <
int dim,
int spacedim>
361 template <
int dim,
int spacedim>
365 const std::set<types::boundary_id> &boundary_ids,
382 template <
int dim,
int spacedim>
387 const unsigned int first_vector_component)
400 for (; face != endf; ++face)
401 if (face->at_boundary() && face->boundary_id() == bid)
402 for (
unsigned int d = 0;
d < dim; ++
d)
410 if (std::abs(std::abs(unit_vec * normal_vec) - 1.0) < 1
e-10)
411 comp_mask.
set(
d + first_vector_component,
true);
414 std::abs(unit_vec * normal_vec) < 1
e-10,
416 "We can currently only support no normal flux conditions "
417 "for a specific boundary id if all faces are normal to the "
418 "x, y, or z axis."));
423 "We can currently only support no normal flux conditions "
424 "for a specific boundary id if all faces are facing in the "
425 "same direction, i.e., a boundary normal to the x-axis must "
426 "have a different boundary id than a boundary normal to the "
427 "y- or z-axis and so on. If the mesh here was produced using "
428 "GridGenerator::..., setting colorize=true during mesh generation "
429 "and calling make_no_normal_flux_constraints() for each no normal "
430 "flux boundary will fulfill the condition."));
438 const unsigned int level,
449 constraints_on_level,
486 const unsigned int level,
490 const IndexSet &interface_dofs_on_level =
bool have_boundary_indices() const
typename ActiveSelector::cell_iterator cell_iterator
unsigned int n_selected_components(const unsigned int overall_number_of_components=numbers::invalid_unsigned_int) const
std::vector< std::set< types::global_dof_index > >::size_type size_dof
const AffineConstraints< double > & get_level_constraint_matrix(const unsigned int level) const
std::vector< AffineConstraints< double > > user_constraints
#define AssertIndexRange(index, range)
SymmetricTensor< 2, dim, Number > e(const Tensor< 2, dim, Number > &F)
virtual Tensor< 1, spacedim > normal_vector(const typename Triangulation< dim, spacedim >::face_iterator &face, const Point< spacedim > &p) const
SymmetricTensor< 2, dim, Number > d(const Tensor< 2, dim, Number > &F, const Tensor< 2, dim, Number > &dF_dt)
std::vector< IndexSet > boundary_indices
const AffineConstraints< double > & get_level_constraints(const unsigned int level) const
bool is_boundary_index(const unsigned int level, const types::global_dof_index index) const
cell_iterator begin(const unsigned int level=0) const
static ::ExceptionBase & ExcMessage(std::string arg1)
const Triangulation< dim, spacedim > & get_triangulation() const
#define DEAL_II_NAMESPACE_OPEN
void set(const unsigned int index, const bool value)
Tensor< 2, dim, Number > l(const Tensor< 2, dim, Number > &F, const Tensor< 2, dim, Number > &dF_dt)
bool at_refinement_edge(const unsigned int level, const types::global_dof_index index) const
bool is_element(const size_type index) const
#define DEAL_II_DEPRECATED
void add_user_constraints(const unsigned int level, const AffineConstraints< double > &constraints_on_level)
bool is_interface_matrix_entry(const unsigned int level, const types::global_dof_index i, const types::global_dof_index j) const
std::vector< AffineConstraints< double > > level_constraints
cell_iterator end() const
const AffineConstraints< double > & get_user_constraint_matrix(const unsigned int level) const
static ::ExceptionBase & ExcInternalError()
const IndexSet & get_boundary_indices(const unsigned int level) const
types::global_dof_index size_type
#define Assert(cond, exc)
void initialize(const DoFHandler< dim, spacedim > &dof)
void make_zero_boundary_constraints(const DoFHandler< dim, spacedim > &dof, const std::set< types::boundary_id > &boundary_ids, const ComponentMask &component_mask=ComponentMask())
const IndexSet & get_refinement_edge_indices(unsigned int level) const
const types::subdomain_id artificial_subdomain_id
#define DEAL_II_NAMESPACE_CLOSE
void make_no_normal_flux_constraints(const DoFHandler< dim, spacedim > &dof, const types::boundary_id bid, const unsigned int first_vector_component)
std::vector< IndexSet > refinement_edge_indices
const FiniteElement< dim, spacedim > & get_fe(const unsigned int index=0) const
const Manifold< dim, spacedim > & get_manifold(const types::manifold_id number) const
types::global_dof_index n_dofs() const