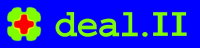 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_matrix_tools_h
17 #define dealii_matrix_tools_h
31 #ifdef DEAL_II_WITH_PETSC
32 # include <petscsys.h>
44 template <
typename number>
46 template <
typename number>
48 template <
typename number>
51 template <
typename number>
53 template <
typename Number>
56 template <
int dim,
int spacedim>
58 template <
int dim,
int spacedim>
66 class MappingCollection;
72 # ifdef DEAL_II_WITH_PETSC
85 # ifdef DEAL_II_WITH_TRILINOS
254 template <
int dim,
int spacedim,
typename number>
268 template <
int dim,
int spacedim,
typename number>
296 template <
int dim,
int spacedim,
typename number>
312 template <
int dim,
int spacedim,
typename number>
326 template <
int dim,
int spacedim,
typename number>
339 template <
int dim,
int spacedim,
typename number>
351 template <
int dim,
int spacedim,
typename number>
366 template <
int dim,
int spacedim,
typename number>
403 template <
int dim,
int spacedim,
typename number>
411 & boundary_functions,
413 std::vector<types::global_dof_index> & dof_to_boundary_mapping,
415 std::vector<unsigned int> component_mapping = {});
422 template <
int dim,
int spacedim,
typename number>
429 & boundary_functions,
431 std::vector<types::global_dof_index> & dof_to_boundary_mapping,
433 std::vector<unsigned int> component_mapping = {});
438 template <
int dim,
int spacedim,
typename number>
446 & boundary_functions,
448 std::vector<types::global_dof_index> & dof_to_boundary_mapping,
450 std::vector<unsigned int> component_mapping = {});
455 template <
int dim,
int spacedim,
typename number>
462 & boundary_functions,
464 std::vector<types::global_dof_index> & dof_to_boundary_mapping,
466 std::vector<unsigned int> component_mapping = {});
487 template <
int dim,
int spacedim>
501 template <
int dim,
int spacedim>
528 template <
int dim,
int spacedim>
544 template <
int dim,
int spacedim>
559 template <
int dim,
int spacedim>
573 template <
int dim,
int spacedim>
586 template <
int dim,
int spacedim>
602 template <
int dim,
int spacedim>
617 "You are providing either a right hand side function or a "
618 "coefficient with a number of vector components that is "
619 "inconsistent with the rest of the arguments. If you do "
620 "provide a coefficient or right hand side function, then "
621 "it either needs to have as many components as the finite "
622 "element in use, or only a single vector component. In "
623 "the latter case, the same value will be taken for "
624 "each vector component of the finite element.");
834 template <
typename number>
837 const std::map<types::global_dof_index, number> &boundary_values,
841 const bool eliminate_columns =
true);
848 template <
typename number>
851 const std::map<types::global_dof_index, number> &boundary_values,
855 const bool eliminate_columns =
true);
857 #ifdef DEAL_II_WITH_PETSC
895 const std::map<types::global_dof_index, PetscScalar> &boundary_values,
899 const bool eliminate_columns =
true);
906 const std::map<types::global_dof_index, PetscScalar> &boundary_values,
910 const bool eliminate_columns =
true);
914 #ifdef DEAL_II_WITH_TRILINOS
950 const std::map<types::global_dof_index, TrilinosScalar> &boundary_values,
954 const bool eliminate_columns =
true);
962 const std::map<types::global_dof_index, TrilinosScalar> &boundary_values,
966 const bool eliminate_columns =
true);
987 template <
typename number>
990 const std::map<types::global_dof_index, number> &boundary_values,
991 const std::vector<types::global_dof_index> & local_dof_indices,
994 const bool eliminate_columns);
1000 "You are providing a matrix whose subdivision into "
1001 "blocks in either row or column direction does not use "
1002 "the same blocks sizes as the solution vector or "
1003 "right hand side vectors, respectively.");
#define DeclExceptionMsg(Exception, defaulttext)
void create_laplace_matrix(const Mapping< dim, spacedim > &mapping, const DoFHandler< dim, spacedim > &dof, const Quadrature< dim > &q, SparseMatrix< double > &matrix, const Function< spacedim > *const a=nullptr, const AffineConstraints< double > &constraints=AffineConstraints< double >())
Abstract base class for mapping classes.
void create_boundary_mass_matrix(const Mapping< dim, spacedim > &mapping, const DoFHandler< dim, spacedim > &dof, const Quadrature< dim - 1 > &q, SparseMatrix< number > &matrix, const std::map< types::boundary_id, const Function< spacedim, number > * > &boundary_functions, Vector< number > &rhs_vector, std::vector< types::global_dof_index > &dof_to_boundary_mapping, const Function< spacedim, number > *const weight=0, std::vector< unsigned int > component_mapping={})
#define DEAL_II_NAMESPACE_OPEN
void create_mass_matrix(const Mapping< dim, spacedim > &mapping, const DoFHandler< dim, spacedim > &dof, const Quadrature< dim > &q, SparseMatrix< number > &matrix, const Function< spacedim, number > *const a=nullptr, const AffineConstraints< number > &constraints=AffineConstraints< number >())
@ matrix
Contents is actually a matrix.
static ::ExceptionBase & ExcBlocksDontMatch()
static ::ExceptionBase & ExcComponentMismatch()
#define DEAL_II_NAMESPACE_CLOSE