Loading [MathJax]/extensions/TeX/AMSsymbols.js
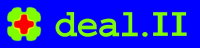 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_block_vector_h
17 #define dealii_block_vector_h
37 # ifdef DEAL_II_WITH_TRILINOS
70 template <
typename Number>
135 template <typename OtherNumber>
138 #ifdef DEAL_II_WITH_TRILINOS
150 BlockVector(
const std::vector<size_type> &block_sizes);
167 template <
typename InputIterator>
168 BlockVector(
const std::vector<size_type> &block_sizes,
169 const InputIterator
first,
170 const InputIterator
end);
225 template <
class Number2>
235 #ifdef DEAL_II_WITH_TRILINOS
259 const bool omit_zeroing_entries =
false);
278 reinit(
const std::vector<size_type> &block_sizes,
279 const bool omit_zeroing_entries =
false);
292 const bool omit_zeroing_entries =
false);
307 template <
typename Number2>
310 const bool omit_zeroing_entries =
false);
316 template <
class BlockVector2>
318 scale(
const BlockVector2 &v);
338 print(std::ostream & out,
339 const unsigned int precision = 3,
340 const bool scientific =
true,
341 const bool across =
true)
const;
384 template <
typename Number>
385 template <
typename InputIterator>
387 const InputIterator
first,
388 const InputIterator
end)
394 reinit(block_sizes,
true);
395 InputIterator start =
first;
398 InputIterator
end = start;
403 Assert(start ==
end, ExcIteratorRangeDoesNotMatchVectorSize());
408 template <
typename Number>
414 BaseClass::operator=(s);
420 template <
typename Number>
425 BaseClass::operator=(v);
431 template <
typename Number>
435 BaseClass::operator=(v);
441 template <
typename Number>
442 template <
typename Number2>
447 BaseClass::operator=(v);
451 template <
typename Number>
456 this->components[i].
compress(operation);
461 template <
typename Number>
470 template <
typename Number>
471 template <
class BlockVector2>
489 template <
typename Number>
499 namespace LinearOperatorImplementation
508 template <
typename number>
512 template <
typename Matrix>
516 bool omit_zeroing_entries)
518 v.
reinit(
matrix.get_row_indices(), omit_zeroing_entries);
521 template <
typename Matrix>
525 bool omit_zeroing_entries)
527 v.
reinit(
matrix.get_column_indices(), omit_zeroing_entries);
540 template <
typename Number>
static void reinit_domain_vector(const Matrix &matrix, BlockVector< number > &v, bool omit_zeroing_entries)
BlockVector & operator=(const value_type s)
typename BlockType::value_type value_type
typename BaseClass::BlockType BlockType
static ::ExceptionBase & ExcIteratorRangeDoesNotMatchVectorSize()
void scale(const BlockVector2 &v)
typename BaseClass::const_pointer const_pointer
typename BaseClass::real_type real_type
typename BlockType::reference reference
typename BlockType::const_reference const_reference
std::enable_if< IsBlockVector< VectorType >::value, unsigned int >::type n_blocks(const VectorType &vector)
bool has_ghost_elements() const
void block_read(std::istream &in)
#define AssertIsFinite(number)
VectorType::value_type * begin(VectorType &V)
void reinit(MatrixBlock< MatrixType > &v, const BlockSparsityPattern &p)
#define DEAL_II_NAMESPACE_OPEN
unsigned int n_blocks() const
void swap(BlockVector< Number > &v)
SymmetricTensor< 2, dim, Number > b(const Tensor< 2, dim, Number > &F)
typename BaseClass::iterator iterator
@ matrix
Contents is actually a matrix.
VectorType::value_type * end(VectorType &V)
void swap(BlockIndices &u, BlockIndices &v)
Vector< Number > BlockType
const value_type * const_pointer
types::global_dof_index size_type
BlockVector(const unsigned int n_blocks=0, const size_type block_size=0)
typename BlockType::real_type real_type
void block_write(std::ostream &out) const
::internal::BlockVectorIterators::Iterator< BlockVectorBase, true > const_iterator
BlockIndices block_indices
void advance(std::tuple< I1, I2 > &t, const unsigned int n)
void reinit(const unsigned int n_blocks, const size_type block_size=0, const bool omit_zeroing_entries=false)
types::global_dof_index size_type
::internal::BlockVectorIterators::Iterator< BlockVectorBase, false > iterator
#define Assert(cond, exc)
typename BaseClass::size_type size_type
typename BaseClass::reference reference
static void reinit_range_vector(const Matrix &matrix, BlockVector< number > &v, bool omit_zeroing_entries)
#define DeclException0(Exception0)
typename BaseClass::const_reference const_reference
typename BaseClass::const_iterator const_iterator
void print(std::ostream &out, const unsigned int precision=3, const bool scientific=true, const bool across=true) const
#define DEAL_II_NAMESPACE_CLOSE
void compress(::VectorOperation::values operation=::VectorOperation::unknown)
std::string compress(const std::string &input)
typename BaseClass::pointer pointer
~BlockVector() override=default
void copy(const T *begin, const T *end, U *dest)
typename BaseClass::value_type value_type