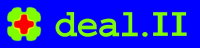 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_q_collection_h
17 #define dealii_q_collection_h
71 template <
class... QTypes>
72 explicit QCollection(
const QTypes &... quadrature_objects);
156 template <
class... QTypes>
160 "Not all of the input arguments of this function "
161 "are derived from Quadrature<dim>!");
166 const auto q_pointers = {
168 for (
const auto p : q_pointers)
178 return quadratures.size();
187 Assert(quadratures.size() > 0,
188 ExcMessage(
"You can't call this function for an empty collection"));
191 for (
unsigned int i = 0; i < quadratures.size(); ++i)
192 if (quadratures[i]->size() > m)
193 m = quadratures[i]->size();
205 return *quadratures[index];
214 const unsigned int n_quadratures = size();
215 if (n_quadratures != q_collection.
size())
218 for (
unsigned int i = 0; i < n_quadratures; ++i)
219 if (!(*quadratures[i] == q_collection[i]))
230 quadratures.push_back(std::make_shared<
const Quadrature<dim>>(quadrature));
247 quadratures.push_back(
std::vector< std::shared_ptr< const Quadrature< dim > > > quadratures
std::size_t memory_consumption() const
const Quadrature< dim > & operator[](const unsigned int index) const
#define AssertIndexRange(index, range)
unsigned int size() const
unsigned int max_n_quadrature_points() const
static ::ExceptionBase & ExcMessage(std::string arg1)
#define DEAL_II_NAMESPACE_OPEN
std::enable_if< std::is_fundamental< T >::value, std::size_t >::type memory_consumption(const T &t)
#define Assert(cond, exc)
#define DeclException0(Exception0)
static ::ExceptionBase & ExcNoQuadrature()
bool operator==(const QCollection< dim > &q_collection) const
#define DEAL_II_NAMESPACE_CLOSE
void push_back(const Quadrature< dim > &new_quadrature)