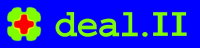 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
25 template <
int dim,
int spacedim>
27 const unsigned int polynomial_degree)
33 template <
int dim,
int spacedim>
37 , support_point_cache(mapping.support_point_cache)
42 template <
int dim,
int spacedim>
49 support_point_cache.reset();
50 clear_signal.disconnect();
55 template <
int dim,
int spacedim>
56 std::unique_ptr<Mapping<dim, spacedim>>
59 return std_cxx14::make_unique<MappingQCache<dim, spacedim>>(*this);
64 template <
int dim,
int spacedim>
73 template <
int dim,
int spacedim>
89 template <
int dim,
int spacedim>
95 &compute_points_on_cell)
98 [&]() ->
void { this->support_point_cache.reset(); });
100 support_point_cache =
101 std::make_shared<std::vector<std::vector<std::vector<Point<spacedim>>>>>(
112 (*support_point_cache)[cell->level()][cell->index()] =
113 compute_points_on_cell(cell);
115 (*support_point_cache)[cell->level()][cell->index()].size(),
116 Utilities::pow(this->get_degree() + 1, dim));
118 std::function<
void(
void *)>(),
127 template <
int dim,
int spacedim>
131 if (support_point_cache.get() !=
nullptr)
132 return sizeof(*this) +
135 return sizeof(*this);
140 template <
int dim,
int spacedim>
141 std::vector<Point<spacedim>>
145 Assert(support_point_cache.get() !=
nullptr,
146 ExcMessage(
"Must call MappingQCache::initialize() before "
147 "using it or after mesh has changed!"));
150 AssertIndexRange(cell->index(), (*support_point_cache)[cell->level()].size());
151 return (*support_point_cache)[cell->level()][cell->index()];
157 #include "mapping_q_cache.inst"
friend class MappingQCache
static unsigned int n_threads()
void initialize(const Triangulation< dim, spacedim > &triangulation, const MappingQGeneric< dim, spacedim > &mapping)
#define AssertIndexRange(index, range)
std::size_t memory_consumption() const
void run(const std::vector< std::vector< Iterator >> &colored_iterators, Worker worker, Copier copier, const ScratchData &sample_scratch_data, const CopyData &sample_copy_data, const unsigned int queue_length=2 *MultithreadInfo::n_threads(), const unsigned int chunk_size=8)
static ::ExceptionBase & ExcMessage(std::string arg1)
#define DEAL_II_NAMESPACE_OPEN
std::enable_if< std::is_fundamental< T >::value, std::size_t >::type memory_consumption(const T &t)
Tensor< 2, dim, Number > l(const Tensor< 2, dim, Number > &F, const Tensor< 2, dim, Number > &dF_dt)
#define AssertDimension(dim1, dim2)
virtual std::vector< Point< spacedim > > compute_mapping_support_points(const typename Triangulation< dim, spacedim >::cell_iterator &cell) const override
virtual std::vector< Point< spacedim > > compute_mapping_support_points(const typename Triangulation< dim, spacedim >::cell_iterator &cell) const
virtual std::unique_ptr< Mapping< dim, spacedim > > clone() const override
unsigned int get_degree() const
#define Assert(cond, exc)
virtual bool preserves_vertex_locations() const override
const typename ::parallel::distributed::Triangulation< dim, spacedim > * triangulation
#define DEAL_II_NAMESPACE_CLOSE