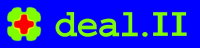 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_grid_in_h
17 #define dealii_grid_in_h
34 template <
int dim,
int space_dim>
306 template <
int dim,
int spacedim = dim>
450 const bool apply_all_indicators_to_manifolds =
false);
493 const bool apply_all_indicators_to_manifolds =
false);
574 const bool remove_duplicates =
true,
575 const double tol = 1
e-12,
576 const bool ignore_unsupported_element_types =
true);
603 <<
"The section type <" << arg1 <<
"> in an UNV "
604 <<
"input file is not implemented.");
611 <<
"The element type <" << arg1 <<
"> in an UNV "
612 <<
"input file is not implemented.");
619 <<
"The identifier <" << arg1 <<
"> as name of a "
620 <<
"part in an UCD input file is unknown or the "
621 <<
"respective input routine is not implemented."
622 <<
"(Maybe the space dimension of triangulation and "
623 <<
"input file do not match?");
635 <<
"While creating cell " << arg1
636 <<
", you are referencing a vertex with index " << arg2
637 <<
" but no vertex with this index has been described in the input file.");
646 <<
"While creating cell " << arg1 <<
" (which is numbered as " << arg2
647 <<
" in the input file), you are referencing a vertex with index " << arg3
648 <<
" but no vertex with this index has been described in the input file.");
658 <<
"The string <" << arg1
659 <<
"> is not recognized at the present"
660 <<
" position of a DB Mesh file.");
668 <<
"The specified dimension " << arg1
669 <<
" is not the same as that of the triangulation to be created.");
673 <<
"The string <" << arg1
674 <<
"> is not recognized at the present"
675 <<
" position of a Gmsh Mesh file.");
679 <<
"The Element Identifier <" << arg1 <<
"> is not "
680 <<
"supported in the deal.II library when "
681 <<
"reading meshes in " << dim <<
" dimensions.\n"
682 <<
"Supported elements are: \n"
684 <<
"1 Line (2 nodes, 1 edge).\n"
685 <<
"3 Quadrilateral (4 nodes, 4 edges).\n"
686 <<
"5 Hexahedron (8 nodes, 12 edges, 6 faces) when in 3d.\n"
687 <<
"15 Point (1 node, ignored when read)");
750 std::vector<unsigned int> &tecplot2deal,
751 unsigned int & n_vars,
752 unsigned int & n_vertices,
754 std::vector<unsigned int> &IJK,
static std::string default_suffix(const Format format)
static void debug_output_grid(const std::vector< CellData< dim >> &cells, const std::vector< Point< spacedim >> &vertices, std::ostream &out)
void read(std::istream &in, Format format=Default)
static ::ExceptionBase & ExcGmshUnsupportedGeometry(int arg1)
static ::ExceptionBase & ExcInvalidGMSHInput(std::string arg1)
void read_ucd(std::istream &in, const bool apply_all_indicators_to_manifolds=false)
static ::ExceptionBase & ExcInvalidVertexIndex(int arg1, int arg2)
@ tecplot
Use read_tecplot()
void read_xda(std::istream &in)
void read_abaqus(std::istream &in, const bool apply_all_indicators_to_manifolds=false)
void attach_triangulation(Triangulation< dim, spacedim > &tria)
static Format parse_format(const std::string &format_name)
static ::ExceptionBase & ExcUnknownSectionType(int arg1)
static ::ExceptionBase & ExcInvalidDBMeshFormat()
@ abaqus
Use read_abaqus()
static ::ExceptionBase & ExcGmshNoCellInformation()
void read_dbmesh(std::istream &in)
SymmetricTensor< 2, dim, Number > e(const Tensor< 2, dim, Number > &F)
static ::ExceptionBase & ExcInvalidVertexIndexGmsh(int arg1, int arg2, int arg3)
void read_unv(std::istream &in)
static ::ExceptionBase & ExcDBMESHWrongDimension(int arg1)
@ dbmesh
Use read_dbmesh()
#define DEAL_II_NAMESPACE_OPEN
void read_vtu(std::istream &in)
#define DeclException3(Exception3, type1, type2, type3, outsequence)
#define DEAL_II_DEPRECATED
#define DeclException1(Exception1, type1, outsequence)
static std::string get_format_names()
static void parse_tecplot_header(std::string &header, std::vector< unsigned int > &tecplot2deal, unsigned int &n_vars, unsigned int &n_vertices, unsigned int &n_cells, std::vector< unsigned int > &IJK, bool &structured, bool &blocked)
void read_assimp(const std::string &filename, const unsigned int mesh_index=numbers::invalid_unsigned_int, const bool remove_duplicates=true, const double tol=1e-12, const bool ignore_unsupported_element_types=true)
SmartPointer< Triangulation< dim, spacedim >, GridIn< dim, spacedim > > tria
static ::ExceptionBase & ExcInvalidDBMESHInput(std::string arg1)
static void skip_comment_lines(std::istream &in, const char comment_start)
static ::ExceptionBase & ExcNoTriangulationSelected()
void read_vtk(std::istream &in)
#define DeclException0(Exception0)
static const unsigned int invalid_unsigned_int
static void skip_empty_lines(std::istream &in)
@ Default
Use GridIn::default_format stored in this object.
@ netcdf
Use read_netcdf()
void read_msh(std::istream &in)
static ::ExceptionBase & ExcUnknownIdentifier(std::string arg1)
unsigned int n_cells(const internal::TriangulationImplementation::NumberCache< 1 > &c)
static ::ExceptionBase & ExcUnknownElementType(int arg1)
#define DEAL_II_NAMESPACE_CLOSE
@ assimp
Use read_assimp()
#define DeclException2(Exception2, type1, type2, outsequence)
void read_netcdf(const std::string &filename)
void read_tecplot(std::istream &in)