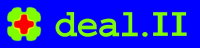 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_grid_construction_utilities_h
17 #define dealii_grid_construction_utilities_h
66 template <
int structdim>
135 template <
class Archive>
137 serialize(Archive &ar,
const unsigned int version);
139 static_assert(structdim > 0,
140 "The class CellData can only be used for structdim>0.");
230 template <
int structdim>
231 template <
class Archive>
287 template <
class Archive>
289 serialize(Archive &ar,
const unsigned int );
322 std::array<types::manifold_id, GeometryInfo<dim>::lines_per_cell>
346 template <
int dim,
int spacedim>
352 template <
class Archive>
354 serialize(Archive &ar,
const unsigned int );
442 template <
int dim,
int spacedim = dim>
445 const ::Triangulation<dim, spacedim> &tria,
482 template <
int dim,
int spacedim = dim>
486 & serial_grid_generator,
489 const unsigned int)> &serial_grid_partitioner,
491 const int group_size = 1,
502 template <
class Archive>
508 ar &level_subdomain_id;
511 ar &manifold_line_ids;
513 ar &manifold_quad_ids;
518 template <
int dim,
int spacedim>
519 template <
class Archive>
525 ar &coarse_cell_vertices;
526 ar &coarse_cell_index_to_coarse_cell_id;
538 if (this->
id != other.
id)
558 template <
int dim,
int spacedim>
567 if (this->coarse_cell_index_to_coarse_cell_id !=
572 if (this->settings != other.
settings)
void serialize(Archive &ar, const unsigned int)
std::vector< std::vector< CellData< dim > > > cell_infos
std::vector< CellData< 2 > > boundary_quads
void serialize(Archive &ar, const unsigned int version)
bool operator==(const CellData< dim > &other) const
unsigned int vertices[GeometryInfo< structdim >::vertices_per_cell]
bool operator==(const Description< dim, spacedim > &other) const
std::vector< types::coarse_cell_id > coarse_cell_index_to_coarse_cell_id
std::vector< Point< spacedim > > coarse_cell_vertices
Description< dim, spacedim > create_description_from_triangulation(const ::Triangulation< dim, spacedim > &tria, const MPI_Comm comm, const TriangulationDescription::Settings settings=TriangulationDescription::Settings::default_setting, const unsigned int my_rank_in=numbers::invalid_unsigned_int)
#define DEAL_II_NAMESPACE_OPEN
types::boundary_id boundary_id
types::manifold_id manifold_id
void serialize(Archive &ar, const unsigned int)
types::subdomain_id level_subdomain_id
Triangulation< dim, spacedim >::MeshSmoothing smoothing
types::subdomain_id subdomain_id
unsigned int subdomain_id
std::vector< std::pair< unsigned int, types::boundary_id > > boundary_ids
bool check_consistency(const unsigned int dim) const
static const unsigned int invalid_unsigned_int
std::array< unsigned int, 4 > binary_type
Description< dim, spacedim > create_description_from_triangulation_in_groups(const std::function< void(::Triangulation< dim, spacedim > &)> &serial_grid_generator, const std::function< void(::Triangulation< dim, spacedim > &, const MPI_Comm, const unsigned int)> &serial_grid_partitioner, const MPI_Comm comm, const int group_size=1, const typename Triangulation< dim, spacedim >::MeshSmoothing smoothing=::Triangulation< dim, spacedim >::none, const TriangulationDescription::Settings setting=TriangulationDescription::Settings::default_setting)
std::vector< CellData< 1 > > boundary_lines
types::material_id material_id
#define DEAL_II_NAMESPACE_CLOSE
std::array< types::manifold_id, dim==1 ? 1 :GeometryInfo< 3 >::quads_per_cell > manifold_quad_ids
std::vector<::CellData< dim > > coarse_cells
bool operator==(const CellData< structdim > &other) const
@ construct_multigrid_hierarchy
std::array< types::manifold_id, GeometryInfo< dim >::lines_per_cell > manifold_line_ids
types::manifold_id manifold_id