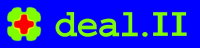 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
32 template <
int dim,
int spacedim,
class... Arguments>
36 const std::string & arguments,
39 std::function<void(Arguments...)> wrapper =
40 [&tria, &generator](Arguments... args) { generator(tria, args...); };
42 bound_function.parse_arguments(arguments);
52 template <
int dim,
int spacedim>
53 typename std::enable_if<dim != spacedim, bool>::type
54 generate_codimension_zero_grid(
const std::string &,
68 generate_codimension_zero_grid(
const std::string & name,
69 const std::string & arguments,
72 if (name ==
"simplex")
73 parse_and_create<dim, dim, const std::vector<Point<dim>> &>(
simplex,
76 else if (name ==
"subdivided_hyper_rectangle")
86 const std::vector<unsigned int> &,
97 const std::vector<std::vector<double>> &,
105 else if (name ==
"plate_with_a_hole")
106 parse_and_create<dim,
120 else if (name ==
"channel_with_cylinder")
121 parse_and_create<dim, dim, double, unsigned int, double, bool>(
124 else if (name ==
"enclosed_hyper_cube")
125 parse_and_create<dim, dim, double, double, double, bool>(
128 else if (name ==
"hyper_ball")
133 else if (name ==
"quarter_hyper_ball")
134 parse_and_create<dim, dim, const Point<dim> &,
double>(
137 else if (name ==
"half_hyper_ball")
138 parse_and_create<dim, dim, const Point<dim> &,
double>(
half_hyper_ball,
142 else if (name ==
"cylinder")
143 parse_and_create<dim, dim, double, double>(
cylinder, arguments, tria);
145 else if (name ==
"truncated_cone")
146 parse_and_create<dim, dim, double, double, double>(
truncated_cone,
150 else if (name ==
"hyper_L")
151 parse_and_create<dim, dim, double, double, bool>(
hyper_L,
155 else if (name ==
"hyper_cube_slit")
160 else if (name ==
"hyper_shell")
161 parse_and_create<dim,
169 else if (name ==
"half_hyper_shell")
170 parse_and_create<dim,
178 else if (name ==
"quarter_hyper_shell")
179 parse_and_create<dim,
187 else if (name ==
"cylinder_shell")
188 parse_and_create<dim,
196 else if (name ==
"hyper_cube_with_cylindrical_hole")
197 parse_and_create<dim, dim, double, double, double, unsigned int, bool>(
200 else if (name ==
"concentric_hyper_shells")
201 parse_and_create<dim,
222 template <
int dim,
int spacedim>
223 typename std::enable_if<dim != spacedim - 1,
bool>::type
224 generate_codimension_one_grid(
const std::string &,
238 generate_codimension_one_grid(
const std::string & name,
239 const std::string & arguments,
242 if (name ==
"hyper_sphere")
243 parse_and_create<dim, dim + 1, const Point<dim + 1> &,
double>(
255 template <
int dim,
int spacedim>
257 generate_special(
const std::string &,
270 generate_special(
const std::string & name,
271 const std::string & arguments,
274 if (name ==
"moebius")
275 parse_and_create<3, 3, unsigned int, unsigned int, double, double>(
277 else if (name ==
"torus")
278 parse_and_create<3, 3, double, double, unsigned int, double>(
torus,
294 generate_special(
const std::string & name,
295 const std::string & arguments,
299 parse_and_create<2, 3, double, double, unsigned int, double>(
torus,
312 template <
int dim,
int spacedim>
315 const std::string & name,
316 const std::string & arguments)
320 if (name ==
"hyper_cube")
321 parse_and_create<dim, spacedim, double, double, bool>(
hyper_cube,
324 else if (name ==
"subdivided_hyper_cube")
325 parse_and_create<dim, spacedim, unsigned int, double, double, bool>(
327 else if (name ==
"hyper_rectangle")
328 parse_and_create<dim,
333 else if (name ==
"cheese")
334 parse_and_create<dim, spacedim, const std::vector<unsigned int> &>(
336 else if (name ==
"general_cell")
337 parse_and_create<dim,
339 const std::vector<Point<spacedim>> &,
341 else if (name ==
"hyper_cross")
342 parse_and_create<dim, spacedim, const std::vector<unsigned int> &,
bool>(
351 else if (generate_codimension_zero_grid(name, arguments, tria))
353 else if (generate_codimension_one_grid(name, arguments, tria))
355 else if (generate_special(name, arguments, tria))
361 ExcMessage(name +
"(" + arguments +
") not implemented"));
365 #include "grid_generator_from_name.inst"
void hyper_cube_with_cylindrical_hole(Triangulation< dim > &triangulation, const double inner_radius=.25, const double outer_radius=.5, const double L=.5, const unsigned int repetitions=1, const bool colorize=false)
void subdivided_hyper_cube(Triangulation< dim, spacedim > &tria, const unsigned int repetitions, const double left=0., const double right=1., const bool colorize=false)
void simplex(Triangulation< dim, dim > &tria, const std::vector< Point< dim >> &vertices)
void enclosed_hyper_cube(Triangulation< dim > &tria, const double left=0., const double right=1., const double thickness=1., const bool colorize=false)
void concentric_hyper_shells(Triangulation< dim > &triangulation, const Point< dim > ¢er, const double inner_radius=0.125, const double outer_radius=0.25, const unsigned int n_shells=1, const double skewness=0.1, const unsigned int n_cells_per_shell=0, const bool colorize=false)
void general_cell(Triangulation< dim, spacedim > &tria, const std::vector< Point< spacedim >> &vertices, const bool colorize=false)
void truncated_cone(Triangulation< dim > &tria, const double radius_0=1.0, const double radius_1=0.5, const double half_length=1.0)
void torus(Triangulation< dim, spacedim > &tria, const double R, const double r, const unsigned int n_cells_toroidal=6, const double phi=2.0 *numbers::PI)
void quarter_hyper_shell(Triangulation< dim > &tria, const Point< dim > ¢er, const double inner_radius, const double outer_radius, const unsigned int n_cells=0, const bool colorize=false)
void channel_with_cylinder(Triangulation< dim > &tria, const double shell_region_width=0.03, const unsigned int n_shells=2, const double skewness=2.0, const bool colorize=false)
void hyper_rectangle(Triangulation< dim, spacedim > &tria, const Point< dim > &p1, const Point< dim > &p2, const bool colorize=false)
void half_hyper_ball(Triangulation< dim > &tria, const Point< dim > ¢er=Point< dim >(), const double radius=1.)
void moebius(Triangulation< 3, 3 > &tria, const unsigned int n_cells, const unsigned int n_rotations, const double R, const double r)
void cylinder(Triangulation< dim > &tria, const double radius=1., const double half_length=1.)
void hyper_L(Triangulation< dim > &tria, const double left=-1., const double right=1., const bool colorize=false)
void cylinder_shell(Triangulation< dim > &tria, const double length, const double inner_radius, const double outer_radius, const unsigned int n_radial_cells=0, const unsigned int n_axial_cells=0)
void quarter_hyper_ball(Triangulation< dim > &tria, const Point< dim > ¢er=Point< dim >(), const double radius=1.)
static ::ExceptionBase & ExcMessage(std::string arg1)
void hyper_shell(Triangulation< dim > &tria, const Point< dim > ¢er, const double inner_radius, const double outer_radius, const unsigned int n_cells=0, bool colorize=false)
void hyper_sphere(Triangulation< spacedim - 1, spacedim > &tria, const Point< spacedim > ¢er=Point< spacedim >(), const double radius=1.)
void hyper_cross(Triangulation< dim, spacedim > &tria, const std::vector< unsigned int > &sizes, const bool colorize_cells=false)
A center cell with stacks of cell protruding from each surface.
void subdivided_hyper_rectangle(Triangulation< dim, spacedim > &tria, const std::vector< unsigned int > &repetitions, const Point< dim > &p1, const Point< dim > &p2, const bool colorize=false)
#define DEAL_II_NAMESPACE_OPEN
void hyper_cube_slit(Triangulation< dim > &tria, const double left=0., const double right=1., const bool colorize=false)
void hyper_ball(Triangulation< dim > &tria, const Point< dim > ¢er=Point< dim >(), const double radius=1., const bool attach_spherical_manifold_on_boundary_cells=false)
void hyper_cube(Triangulation< dim, spacedim > &tria, const double left=0., const double right=1., const bool colorize=false)
void cheese(Triangulation< dim, spacedim > &tria, const std::vector< unsigned int > &holes)
Rectangular domain with rectangular pattern of holes.
void half_hyper_shell(Triangulation< dim > &tria, const Point< dim > ¢er, const double inner_radius, const double outer_radius, const unsigned int n_cells=0, const bool colorize=false)
void plate_with_a_hole(Triangulation< dim > &tria, const double inner_radius=0.4, const double outer_radius=1., const double pad_bottom=2., const double pad_top=2., const double pad_left=1., const double pad_right=1., const Point< dim > center=Point< dim >(), const types::manifold_id polar_manifold_id=0, const types::manifold_id tfi_manifold_id=1, const double L=1., const unsigned int n_slices=2, const bool colorize=false)
Rectangular plate with an (offset) cylindrical hole.
void generate_from_name_and_arguments(Triangulation< dim, spacedim > &tria, const std::string &grid_generator_function_name, const std::string &grid_generator_function_arguments)
#define DEAL_II_NAMESPACE_CLOSE
#define AssertThrow(cond, exc)
static ::ExceptionBase & ExcNoMatch(std::string arg1, std::string arg2)
MutableBind< ReturnType, FunctionArgs... > mutable_bind(ReturnType(*function)(FunctionArgs...), typename identity< FunctionArgs >::type &&... arguments)