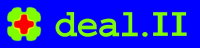 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_distributed_cell_data_transfer_h
17 #define dealii_distributed_cell_data_transfer_h
27 #include <boost/range/iterator_range.hpp>
127 template <
int dim,
int spacedim = dim,
typename VectorType = Vector<
double>>
160 const typename VectorType::value_type
value =
161 input_vector[parent->child(0)->active_cell_index()];
163 for (
unsigned int child_index = 1; child_index < parent->n_children();
167 input_vector[parent->child(child_index)->active_cell_index()],
169 "Values on cells that will be coarsened are not equal!"));
181 cell_iterator & parent,
184 typename VectorType::value_type
sum = 0;
186 for (
unsigned int child_index = 0; child_index < parent->n_children();
189 input_vector[parent->child(child_index)->active_cell_index()];
204 return sum(parent, input_vector) / parent->n_children();
227 const std::function<std::vector<value_type>(
228 const typename ::Triangulation<dim, spacedim>::cell_iterator
232 preserve<dim, spacedim, value_type>,
234 const typename ::Triangulation<dim, spacedim>::cell_iterator
238 check_equality<dim, spacedim, value_type>);
284 const std::vector<const VectorType *> &all_in);
316 unpack(std::vector<VectorType *> &all_out);
348 const std::function<std::vector<value_type>(
359 const std::vector<value_type> &children_values)>
403 const boost::iterator_range<std::vector<char>::const_iterator>
405 std::vector<VectorType *> &all_out);
static VectorType::value_type check_equality(const typename parallel::distributed::Triangulation< dim, spacedim >::cell_iterator &parent, const VectorType &input_vector)
const std::function< value_type(const typename Triangulation< dim, spacedim >::cell_iterator &parent, const std::vector< value_type > &children_values)> coarsening_strategy
SmartPointer< const parallel::distributed::Triangulation< dim, spacedim >, CellDataTransfer< dim, spacedim, VectorType > > triangulation
void deserialize(VectorType &out)
void unpack(VectorType &out)
CellDataTransfer(const parallel::distributed::Triangulation< dim, spacedim > &triangulation, const bool transfer_variable_size_data=false, const std::function< std::vector< value_type >(const typename ::Triangulation< dim, spacedim >::cell_iterator &parent, const value_type parent_value)> refinement_strategy=&::AdaptationStrategies::Refinement::preserve< dim, spacedim, value_type >, const std::function< value_type(const typename ::Triangulation< dim, spacedim >::cell_iterator &parent, const std::vector< value_type > &children_values)> coarsening_strategy=&::AdaptationStrategies::Coarsening::check_equality< dim, spacedim, value_type >)
const bool transfer_variable_size_data
std::vector< const VectorType * > input_vectors
typename std::vector< unsigned int > ::value_type value_type
void prepare_for_coarsening_and_refinement(const VectorType &in)
void unpack_callback(const typename parallel::distributed::Triangulation< dim, spacedim >::cell_iterator &cell, const typename parallel::distributed::Triangulation< dim, spacedim >::CellStatus status, const boost::iterator_range< std::vector< char >::const_iterator > &data_range, std::vector< VectorType * > &all_out)
void register_data_attach()
static ::ExceptionBase & ExcMessage(std::string arg1)
std::vector< char > pack_callback(const typename parallel::distributed::Triangulation< dim, spacedim >::cell_iterator &cell, const typename parallel::distributed::Triangulation< dim, spacedim >::CellStatus status)
#define DEAL_II_NAMESPACE_OPEN
#define DEAL_II_DEPRECATED
T sum(const T &t, const MPI_Comm &mpi_communicator)
void prepare_for_serialization(const VectorType &in)
static VectorType::value_type sum(const typename parallel::distributed::Triangulation< dim, spacedim >::cell_iterator &parent, const VectorType &input_vector)
#define Assert(cond, exc)
const std::function< std::vector< value_type > const typename Triangulation< dim, spacedim >::cell_iterator &parent, const value_type parent_value)> refinement_strategy
#define DEAL_II_NAMESPACE_CLOSE
static VectorType::value_type mean(const typename parallel::distributed::Triangulation< dim, spacedim >::cell_iterator &parent, const VectorType &input_vector)