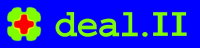 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
17 #ifndef dealii_matrix_free_vector_access_internal_h
18 #define dealii_matrix_free_vector_access_internal_h
41 inline typename VectorType::value_type
55 inline typename VectorType::value_type &
69 inline typename VectorType::value_type &
72 return vec.local_element(entry);
81 inline typename VectorType::value_type
84 return vec.local_element(entry);
94 const unsigned int entry,
95 const typename VectorType::value_type &val)
97 vec.add_local_element(entry, val);
107 const unsigned int entry,
108 const typename VectorType::value_type &val)
121 const typename VectorType::value_type &val)
134 const typename VectorType::value_type &val)
146 const unsigned int entry,
147 const typename VectorType::value_type &val)
149 vec.set_local_element(entry, val);
159 const unsigned int entry,
160 const typename VectorType::value_type &val)
202 "The parallel layout of the given vector is not "
203 "compatible with the parallel partitioning in MatrixFree. "
204 "Use MatrixFree::initialize_dof_vector to get a "
205 "compatible vector."));
216 template <
typename Number,
typename VectorizedArrayType>
219 template <
typename VectorType>
230 template <
typename VectorType>
233 const unsigned int dof_index,
235 VectorizedArrayType *dof_values,
236 std::integral_constant<bool, true>)
const
238 const Number *vec_ptr = vec.begin() + dof_index;
239 for (
unsigned int i = 0; i < dofs_per_cell;
240 ++i, vec_ptr += VectorizedArrayType::size())
241 dof_values[i].load(vec_ptr);
246 template <
typename VectorType>
249 const unsigned int dof_index,
251 VectorizedArrayType *dof_values,
252 std::integral_constant<bool, false>)
const
254 for (
unsigned int i = 0; i < dofs_per_cell; ++i)
255 for (
unsigned int v = 0; v < VectorizedArrayType::size(); ++v)
257 vector_access(vec, dof_index + v + i * VectorizedArrayType::size());
262 template <
typename VectorType>
265 const unsigned int * dof_indices,
267 VectorizedArrayType *dof_values,
268 std::integral_constant<bool, true>)
const
278 template <
typename VectorType>
281 const unsigned int * dof_indices,
283 VectorizedArrayType *dof_values,
284 std::integral_constant<bool, false>)
const
286 for (
unsigned int d = 0;
d < dofs_per_cell; ++
d)
287 for (
unsigned int v = 0; v < VectorizedArrayType::size(); ++v)
295 template <
typename VectorType>
299 const unsigned int constant_offset,
300 VectorizedArrayType &res,
301 std::integral_constant<bool, true>)
const
303 res.gather(vec.begin() + constant_offset, indices);
310 template <
typename VectorType>
314 const unsigned int constant_offset,
315 VectorizedArrayType &res,
316 std::integral_constant<bool, false>)
const
318 for (
unsigned int v = 0; v < VectorizedArrayType::size(); ++v)
324 template <
typename VectorType>
343 template <
typename VectorType>
366 res = VectorizedArrayType();
374 template <
typename Number,
typename VectorizedArrayType>
377 template <
typename VectorType>
386 template <
typename VectorType>
389 const unsigned int dof_index,
391 VectorizedArrayType *dof_values,
392 std::integral_constant<bool, true>)
const
394 Number *vec_ptr = vec.begin() + dof_index;
395 for (
unsigned int i = 0; i < dofs_per_cell;
396 ++i, vec_ptr += VectorizedArrayType::size())
398 VectorizedArrayType tmp;
400 tmp += dof_values[i];
407 template <
typename VectorType>
410 const unsigned int dof_index,
412 VectorizedArrayType *dof_values,
413 std::integral_constant<bool, false>)
const
415 for (
unsigned int i = 0; i < dofs_per_cell; ++i)
416 for (
unsigned int v = 0; v < VectorizedArrayType::size(); ++v)
418 dof_index + v + i * VectorizedArrayType::size(),
424 template <
typename VectorType>
427 const unsigned int * dof_indices,
429 VectorizedArrayType *dof_values,
430 std::integral_constant<bool, true>)
const
433 true, dofs_per_cell, dof_values, dof_indices, vec.begin());
438 template <
typename VectorType>
441 const unsigned int * dof_indices,
443 VectorizedArrayType *dof_values,
444 std::integral_constant<bool, false>)
const
446 for (
unsigned int d = 0;
d < dofs_per_cell; ++
d)
447 for (
unsigned int v = 0; v < VectorizedArrayType::size(); ++v)
455 template <
typename VectorType>
459 const unsigned int constant_offset,
460 VectorizedArrayType &res,
461 std::integral_constant<bool, true>)
const
463 #if DEAL_II_VECTORIZATION_WIDTH_IN_BITS < 512
464 for (
unsigned int v = 0; v < VectorizedArrayType::size(); ++v)
468 VectorizedArrayType tmp;
469 tmp.gather(vec.begin() + constant_offset, indices);
471 tmp.scatter(indices, vec.begin() + constant_offset);
479 template <
typename VectorType>
483 const unsigned int constant_offset,
484 VectorizedArrayType &res,
485 std::integral_constant<bool, false>)
const
487 for (
unsigned int v = 0; v < VectorizedArrayType::size(); ++v)
493 template <
typename VectorType>
512 template <
typename VectorType>
538 template <
typename Number,
typename VectorizedArrayType>
541 template <
typename VectorType>
550 template <
typename VectorType>
553 const unsigned int dof_index,
555 VectorizedArrayType *dof_values,
556 std::integral_constant<bool, true>)
const
558 Number *vec_ptr = vec.begin() + dof_index;
559 for (
unsigned int i = 0; i < dofs_per_cell;
560 ++i, vec_ptr += VectorizedArrayType::size())
561 dof_values[i].store(vec_ptr);
566 template <
typename VectorType>
569 const unsigned int dof_index,
571 VectorizedArrayType *dof_values,
572 std::integral_constant<bool, false>)
const
574 for (
unsigned int i = 0; i < dofs_per_cell; ++i)
575 for (
unsigned int v = 0; v < VectorizedArrayType::size(); ++v)
576 vector_access(vec, dof_index + v + i * VectorizedArrayType::size()) =
582 template <
typename VectorType>
585 const unsigned int * dof_indices,
587 VectorizedArrayType *dof_values,
588 std::integral_constant<bool, true>)
const
591 false, dofs_per_cell, dof_values, dof_indices, vec.begin());
596 template <
typename VectorType,
bool booltype>
599 const unsigned int * dof_indices,
601 VectorizedArrayType *dof_values,
602 std::integral_constant<bool, false>)
const
604 for (
unsigned int i = 0; i < dofs_per_cell; ++i)
605 for (
unsigned int v = 0; v < VectorizedArrayType::size(); ++v)
611 template <
typename VectorType>
615 const unsigned int constant_offset,
616 VectorizedArrayType &res,
617 std::integral_constant<bool, true>)
const
619 res.scatter(indices, vec.begin() + constant_offset);
624 template <
typename VectorType>
628 const unsigned int constant_offset,
629 VectorizedArrayType &res,
630 std::integral_constant<bool, false>)
const
632 for (
unsigned int v = 0; v < VectorizedArrayType::size(); ++v)
638 template <
typename VectorType>
655 template <
typename VectorType>
void process_dof_global(const types::global_dof_index index, VectorType &vec, Number &res) const
void pre_constraints(const Number &, Number &) const
void process_dofs_vectorized_transpose(const unsigned int dofs_per_cell, const unsigned int *dof_indices, VectorType &vec, VectorizedArrayType *dof_values, std::integral_constant< bool, true >) const
void vector_access_add(VectorType &vec, const unsigned int entry, const typename VectorType::value_type &val)
void process_dof_gather(const unsigned int *indices, VectorType &vec, const unsigned int constant_offset, VectorizedArrayType &res, std::integral_constant< bool, true >) const
void process_dofs_vectorized_transpose(const unsigned int dofs_per_cell, const unsigned int *dof_indices, VectorType &vec, VectorizedArrayType *dof_values, std::integral_constant< bool, false >) const
void process_dofs_vectorized(const unsigned int dofs_per_cell, const unsigned int dof_index, VectorType &vec, VectorizedArrayType *dof_values, std::integral_constant< bool, true >) const
void process_dof_gather(const unsigned int *indices, VectorType &vec, const unsigned int constant_offset, VectorizedArrayType &res, std::integral_constant< bool, false >) const
void vector_access_add_global(VectorType &vec, const types::global_dof_index entry, const typename VectorType::value_type &val)
void process_dof_gather(const unsigned int *indices, VectorType &vec, const unsigned int constant_offset, VectorizedArrayType &res, std::integral_constant< bool, true >) const
void process_dof_global(const types::global_dof_index index, VectorType &vec, Number &res) const
void post_constraints(const Number &sum, Number &write_pos) const
void process_dofs_vectorized(const unsigned int dofs_per_cell, const unsigned int dof_index, VectorType &vec, VectorizedArrayType *dof_values, std::integral_constant< bool, true >) const
void pre_constraints(const Number &input, Number &res) const
void vectorized_load_and_transpose(const unsigned int n_entries, const Number *in, const unsigned int *offsets, VectorizedArray< Number, width > *out)
void process_constraint(const unsigned int, const Number, VectorType &, Number &) const
void process_dofs_vectorized_transpose(const unsigned int dofs_per_cell, const unsigned int *dof_indices, const VectorType &vec, VectorizedArrayType *dof_values, std::integral_constant< bool, false >) const
void process_empty(VectorizedArrayType &) const
SymmetricTensor< 2, dim, Number > d(const Tensor< 2, dim, Number > &F, const Tensor< 2, dim, Number > &dF_dt)
void vector_access_set(VectorType &vec, const unsigned int entry, const typename VectorType::value_type &val)
void pre_constraints(const Number &, Number &res) const
std::shared_ptr< const Utilities::MPI::Partitioner > vector_partitioner
void process_dofs_vectorized(const unsigned int dofs_per_cell, const unsigned int dof_index, const VectorType &vec, VectorizedArrayType *dof_values, std::integral_constant< bool, false >) const
static ::ExceptionBase & ExcMessage(std::string arg1)
unsigned int global_dof_index
void process_dof_gather(const unsigned int *indices, VectorType &vec, const unsigned int constant_offset, VectorizedArrayType &res, std::integral_constant< bool, true >) const
void process_dof(const unsigned int index, const VectorType &vec, Number &res) const
#define DEAL_II_NAMESPACE_OPEN
void process_empty(VectorizedArrayType &) const
void post_constraints(const Number &, Number &) const
void process_constraint(const unsigned int index, const Number weight, VectorType &vec, Number &res) const
void process_dof_global(const types::global_dof_index index, const VectorType &vec, Number &res) const
T sum(const T &t, const MPI_Comm &mpi_communicator)
#define AssertDimension(dim1, dim2)
void process_empty(VectorizedArrayType &res) const
void process_dof_gather(const unsigned int *indices, const VectorType &vec, const unsigned int constant_offset, VectorizedArrayType &res, std::integral_constant< bool, false >) const
void post_constraints(const Number &, Number &) const
void process_dofs_vectorized(const unsigned int dofs_per_cell, const unsigned int dof_index, VectorType &vec, VectorizedArrayType *dof_values, std::integral_constant< bool, false >) const
void process_dofs_vectorized(const unsigned int dofs_per_cell, const unsigned int dof_index, VectorType &vec, VectorizedArrayType *dof_values, std::integral_constant< bool, true >) const
#define Assert(cond, exc)
void process_dofs_vectorized(const unsigned int dofs_per_cell, const unsigned int dof_index, VectorType &vec, VectorizedArrayType *dof_values, std::integral_constant< bool, false >) const
void process_dofs_vectorized_transpose(const unsigned int dofs_per_cell, const unsigned int *dof_indices, VectorType &vec, VectorizedArrayType *dof_values, std::integral_constant< bool, false >) const
void process_dofs_vectorized_transpose(const unsigned int dofs_per_cell, const unsigned int *dof_indices, VectorType &vec, VectorizedArrayType *dof_values, std::integral_constant< bool, true >) const
void process_dof_gather(const unsigned int *indices, VectorType &vec, const unsigned int constant_offset, VectorizedArrayType &res, std::integral_constant< bool, false >) const
void process_dofs_vectorized_transpose(const unsigned int dofs_per_cell, const unsigned int *dof_indices, VectorType &vec, VectorizedArrayType *dof_values, std::integral_constant< bool, true >) const
VectorType::value_type vector_access(const VectorType &vec, const unsigned int entry)
#define DEAL_II_NAMESPACE_CLOSE
void process_dof(const unsigned int index, VectorType &vec, Number &res) const
void process_dof(const unsigned int index, VectorType &vec, Number &res) const
void process_constraint(const unsigned int index, const Number weight, const VectorType &vec, Number &res) const
void check_vector_compatibility(const VectorType &vec, const internal::MatrixFreeFunctions::DoFInfo &dof_info)
void vectorized_transpose_and_store(const bool add_into, const unsigned int n_entries, const VectorizedArray< Number, width > *in, const unsigned int *offsets, Number *out)