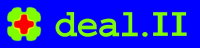 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_tria_levels_h
17 #define dealii_tria_levels_h
27 #include <boost/serialization/utility.hpp>
36 namespace TriangulationImplementation
178 const unsigned int dimension,
179 const unsigned int space_dimension);
200 template <
class Archive>
202 serialize(Archive &ar,
const unsigned int version);
210 <<
"The containers have sizes " << arg1 <<
" and " << arg2
211 <<
", which is not as expected.");
246 const unsigned int dimension,
247 const unsigned int space_dimension);
257 template <
class Archive>
259 serialize(Archive &ar,
const unsigned int version);
267 <<
"The containers have sizes " << arg1 <<
" and " << arg2
268 <<
", which is not as expected.");
274 template <
class Archive>
278 ar &refine_flags &coarsen_flags;
286 ar &level_subdomain_ids;
294 template <
class Archive>
std::vector< std::uint8_t > refine_flags
void reserve_space(const unsigned int total_cells, const unsigned int dimension, const unsigned int space_dimension)
std::vector< bool > direction_flags
std::vector< std::uint8_t > refine_flags
std::vector< std::pair< int, int > > neighbors
std::vector< types::subdomain_id > level_subdomain_ids
std::vector< bool > coarsen_flags
std::vector< std::pair< int, int > > neighbors
std::vector< bool > coarsen_flags
#define DEAL_II_NAMESPACE_OPEN
std::vector< int > parents
std::size_t memory_consumption() const
std::vector< int > parents
void monitor_memory(const unsigned int true_dimension) const
TriaObjects< TriaObject< dim > > cells
std::vector< types::subdomain_id > level_subdomain_ids
std::vector< unsigned int > active_cell_indices
std::vector< unsigned int > active_cell_indices
std::vector< bool > direction_flags
std::vector< types::subdomain_id > subdomain_ids
void serialize(Archive &ar, const unsigned int version)
#define DEAL_II_NAMESPACE_CLOSE
std::vector< types::subdomain_id > subdomain_ids
#define DeclException2(Exception2, type1, type2, outsequence)
static ::ExceptionBase & ExcMemoryInexact(int arg1, int arg2)