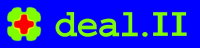 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_transformations_h
17 #define dealii_transformations_h
30 namespace Transformations
60 template <
typename Number>
93 template <
typename Number>
119 namespace Contravariant
139 template <
int dim,
typename Number>
158 template <
int dim,
typename Number>
178 template <
int dim,
typename Number>
197 template <
int dim,
typename Number>
217 template <
int dim,
typename Number>
242 template <
int dim,
typename Number>
261 template <
int dim,
typename Number>
280 template <
int dim,
typename Number>
299 template <
int dim,
typename Number>
318 template <
int dim,
typename Number>
366 template <
int dim,
typename Number>
385 template <
int dim,
typename Number>
405 template <
int dim,
typename Number>
424 template <
int dim,
typename Number>
444 template <
int dim,
typename Number>
469 template <
int dim,
typename Number>
488 template <
int dim,
typename Number>
507 template <
int dim,
typename Number>
526 template <
int dim,
typename Number>
545 template <
int dim,
typename Number>
582 template <
int dim,
typename Number>
602 template <
int dim,
typename Number>
623 template <
int dim,
typename Number>
644 template <
int dim,
typename Number>
666 template <
int dim,
typename Number>
693 template <
int dim,
typename Number>
713 template <
int dim,
typename Number>
733 template <
int dim,
typename Number>
754 template <
int dim,
typename Number>
775 template <
int dim,
typename Number>
810 template <
int dim,
typename Number>
829 template <
int dim,
typename Number>
834 return contract<1, 0>(
F,
V);
839 template <
int dim,
typename Number>
844 return contract<1, 0>(
F, contract<1, 1>(
T,
F));
849 template <
int dim,
typename Number>
850 inline ::SymmetricTensor<2, dim, Number>
851 transformation_contraction(const ::SymmetricTensor<2, dim, Number> &
T,
855 for (
unsigned int i = 0; i < dim; ++i)
856 for (
unsigned int J = 0; J < dim; ++J)
858 for (
unsigned int I_ = 0; I_ < dim; ++I_)
859 tmp_1[i][J] +=
F[i][I_] *
T[I_][J];
862 for (
unsigned int i = 0; i < dim; ++i)
863 for (
unsigned int j = i; j < dim; ++j)
864 for (
unsigned int J = 0; J < dim; ++J)
865 out[i][j] +=
F[j][J] * tmp_1[i][J];
872 template <
int dim,
typename Number>
896 return contract<1, 1>(
897 F, contract<1, 1>(
F, contract<2, 1>(contract<2, 1>(H,
F),
F)));
902 template <
int dim,
typename Number>
903 inline ::SymmetricTensor<4, dim, Number>
904 transformation_contraction(const ::SymmetricTensor<4, dim, Number> &H,
925 for (
unsigned int I_ = 0; I_ < dim; ++I_)
926 for (
unsigned int j = 0; j < dim; ++j)
927 for (
unsigned int K = 0; K < dim; ++K)
928 for (
unsigned int L = 0;
L < dim; ++
L)
929 for (
unsigned int J = 0; J < dim; ++J)
930 tmp[I_][j][K][
L] +=
F[j][J] * H[I_][J][K][
L];
933 tmp = contract<1, 0>(
F, contract<3, 1>(tmp,
F));
937 for (
unsigned int i = 0; i < dim; ++i)
938 for (
unsigned int j = i; j < dim; ++j)
939 for (
unsigned int k = 0; k < dim; ++k)
940 for (
unsigned int l = k;
l < dim; ++
l)
941 for (
unsigned int K = 0; K < dim; ++K)
942 out[i][j][k][
l] +=
F[k][K] * tmp[i][j][K][
l];
951 template <
typename Number>
962 template <
typename Number>
966 const Number &
angle)
969 ExcMessage(
"The supplied axial vector is not a unit vector."));
972 const Number t = 1. - c;
973 const double rotation[3][3] = {{t * axis[0] * axis[0] + c,
974 t * axis[0] * axis[1] - s * axis[2],
975 t * axis[0] * axis[2] + s * axis[1]},
976 {t * axis[0] * axis[1] + s * axis[2],
977 t * axis[1] * axis[1] + c,
978 t * axis[1] * axis[2] - s * axis[0]},
979 {t * axis[0] * axis[2] - s * axis[1],
980 t * axis[1] * axis[2] + s * axis[0],
981 t * axis[2] * axis[2] + c}};
987 template <
int dim,
typename Number>
993 return internal::Physics::transformation_contraction(
V,
F);
998 template <
int dim,
typename Number>
1004 return internal::Physics::transformation_contraction(
T,
F);
1009 template <
int dim,
typename Number>
1015 return internal::Physics::transformation_contraction(
T,
F);
1020 template <
int dim,
typename Number>
1026 return internal::Physics::transformation_contraction(H,
F);
1031 template <
int dim,
typename Number>
1037 return internal::Physics::transformation_contraction(H,
F);
1042 template <
int dim,
typename Number>
1048 return internal::Physics::transformation_contraction(v,
invert(
F));
1053 template <
int dim,
typename Number>
1059 return internal::Physics::transformation_contraction(t,
invert(
F));
1064 template <
int dim,
typename Number>
1070 return internal::Physics::transformation_contraction(t,
invert(
F));
1075 template <
int dim,
typename Number>
1081 return internal::Physics::transformation_contraction(h,
invert(
F));
1086 template <
int dim,
typename Number>
1092 return internal::Physics::transformation_contraction(h,
invert(
F));
1097 template <
int dim,
typename Number>
1108 template <
int dim,
typename Number>
1119 template <
int dim,
typename Number>
1130 template <
int dim,
typename Number>
1136 return internal::Physics::transformation_contraction(H,
transpose(
invert(
F)));
1141 template <
int dim,
typename Number>
1147 return internal::Physics::transformation_contraction(H,
transpose(
invert(
F)));
1152 template <
int dim,
typename Number>
1157 return internal::Physics::transformation_contraction(v,
transpose(
F));
1162 template <
int dim,
typename Number>
1167 return internal::Physics::transformation_contraction(t,
transpose(
F));
1172 template <
int dim,
typename Number>
1178 return internal::Physics::transformation_contraction(t,
transpose(
F));
1183 template <
int dim,
typename Number>
1188 return internal::Physics::transformation_contraction(h,
transpose(
F));
1193 template <
int dim,
typename Number>
1199 return internal::Physics::transformation_contraction(h,
transpose(
F));
1204 template <
int dim,
typename Number>
1214 template <
int dim,
typename Number>
1224 template <
int dim,
typename Number>
1235 template <
int dim,
typename Number>
1245 template <
int dim,
typename Number>
1256 template <
int dim,
typename Number>
1266 template <
int dim,
typename Number>
1276 template <
int dim,
typename Number>
1287 template <
int dim,
typename Number>
1297 template <
int dim,
typename Number>
1308 template <
int dim,
typename Number>
Tensor< 2, dim, Number > F(const Tensor< 2, dim, Number > &Grad_u)
constexpr Tensor< 2, dim, Number > cofactor(const Tensor< 2, dim, Number > &t)
constexpr SymmetricTensor< 2, dim, Number > invert(const SymmetricTensor< 2, dim, Number > &t)
SymmetricTensor< 2, dim, Number > e(const Tensor< 2, dim, Number > &F)
inline ::VectorizedArray< Number, width > cos(const ::VectorizedArray< Number, width > &x)
numbers::NumberTraits< double >::real_type norm() const
static ::ExceptionBase & ExcMessage(std::string arg1)
#define DEAL_II_NAMESPACE_OPEN
Tensor< 2, dim, Number > l(const Tensor< 2, dim, Number > &F, const Tensor< 2, dim, Number > &dF_dt)
#define Assert(cond, exc)
constexpr Number determinant(const SymmetricTensor< 2, dim, Number > &t)
#define DEAL_II_NAMESPACE_CLOSE
inline ::VectorizedArray< Number, width > sin(const ::VectorizedArray< Number, width > &x)