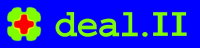 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_table_handler_h
17 #define dealii_table_handler_h
25 #include <boost/serialization/map.hpp>
26 #include <boost/serialization/split_member.hpp>
27 #include <boost/serialization/string.hpp>
28 #include <boost/serialization/vector.hpp>
29 #include <boost/variant.hpp>
98 cache_string(
bool scientific,
unsigned int precision)
const;
120 template <
class Archive>
122 save(Archive &ar,
const unsigned int version)
const;
128 template <
class Archive>
130 load(Archive &ar,
const unsigned int version);
137 template <
class Archive>
139 serialize(Archive &archive,
const unsigned int version);
143 BOOST_SERIALIZATION_SPLIT_MEMBER()
151 boost::variant<int, unsigned int, std::uint64_t, double, std::string>;
163 friend class ::TableHandler;
411 template <
typename T>
452 const std::string &superkey);
479 set_precision(
const std::string &key,
const unsigned int precision);
494 set_tex_caption(
const std::string &key,
const std::string &tex_caption);
515 const std::string &tex_supercaption);
524 set_tex_format(
const std::string &key,
const std::string &format =
"c");
549 write_tex(std::ostream &file,
const bool with_header =
true)
const;
570 template <
class Archive>
572 serialize(Archive &ar,
const unsigned int version);
584 <<
"Column <" << arg1 <<
"> does not exist.");
591 <<
"Supercolumn <" << arg1 <<
"> does not exist.");
598 <<
"Column or supercolumn <" << arg1 <<
"> does not exist.");
608 <<
"Column <" << arg1 <<
"> has " << arg2
609 <<
" rows, but Column <" << arg3 <<
"> has " << arg4
617 <<
"<" << arg1 <<
"> is not a tex column format. Use "
618 <<
"'l', 'c', or 'r' to indicate left, centered, or "
619 <<
"right aligned text.");
649 template <
class Archive>
651 save(Archive &ar,
const unsigned int version)
const;
657 template <
class Archive>
659 load(Archive &ar,
const unsigned int version);
666 template <
class Archive>
668 serialize(Archive &archive,
const unsigned int version);
672 BOOST_SERIALIZATION_SPLIT_MEMBER()
766 mutable std::map<std::string, Column>
columns;
805 template <
typename T>
811 template <
typename T>
823 return boost::get<T>(
value);
829 "This TableEntry object does not store a datum of type T"));
836 template <
class Archive>
843 if (
const int *p = boost::get<int>(&
value))
848 else if (
const unsigned int *p = boost::get<unsigned int>(&
value))
853 else if (
const double *p = boost::get<double>(&
value))
858 else if (
const std::string *p = boost::get<std::string>(&
value))
863 else if (
const std::uint64_t *p = boost::get<std::uint64_t>(&
value))
874 template <
class Archive>
935 template <
typename T>
947 unsigned int max_col_length = 0;
948 for (
const auto &column :
columns)
951 static_cast<unsigned int>(column.second.entries.size()));
953 while (
columns[key].entries.size() + 1 < max_col_length)
960 static_cast<unsigned int>(
976 template <
class Archive>
985 template <
class Archive>
989 ar &entries &tex_caption &tex_format &precision &scientific &flag &max_length;
994 template <
class Archive>
void set_tex_supercaption(const std::string &superkey, const std::string &tex_supercaption)
void add_value(const std::string &key, const T value)
static ::ExceptionBase & ExcSuperColumnNotExistent(std::string arg1)
void set_tex_caption(const std::string &key, const std::string &tex_caption)
void serialize(Archive &archive, const unsigned int version)
void set_scientific(const std::string &key, const bool scientific)
void set_tex_table_label(const std::string &table_label)
void set_column_order(const std::vector< std::string > &new_order)
void pad_column_below(const unsigned int length)
void set_tex_table_caption(const std::string &table_caption)
static ::ExceptionBase & ExcColumnOrSuperColumnNotExistent(std::string arg1)
void cache_string(bool scientific, unsigned int precision) const
std::string tex_table_caption
const std::string & get_cached_string() const
static ::ExceptionBase & ExcWrongNumberOfDataEntries(std::string arg1, int arg2, std::string arg3, int arg4)
TableEntry get_default_constructed_copy() const
#define DEAL_II_ENABLE_EXTRA_DIAGNOSTICS
std::vector< internal::TableEntry > entries
void save(Archive &ar, const unsigned int version) const
std::string tex_table_label
static ::ExceptionBase & ExcMessage(std::string arg1)
static ::ExceptionBase & ExcUndefinedTexFormat(std::string arg1)
#define DEAL_II_NAMESPACE_OPEN
unsigned int n_rows() const
void load(Archive &ar, const unsigned int version)
#define DeclException1(Exception1, type1, outsequence)
double get_numeric_value() const
std::map< std::string, std::vector< std::string > > supercolumns
boost::variant< int, unsigned int, std::uint64_t, double, std::string > value_type
#define DeclException4(Exception4, type1, type2, type3, type4, outsequence)
void set_auto_fill_mode(const bool state)
@ table_with_separate_column_description
static ::ExceptionBase & ExcInternalError()
void save(Archive &ar, const unsigned int version) const
#define Assert(cond, exc)
std::map< std::string, std::string > tex_supercaptions
std::map< std::string, Column > columns
@ simple_table_with_separate_column_description
void add_column_to_supercolumn(const std::string &key, const std::string &superkey)
void set_tex_format(const std::string &key, const std::string &format="c")
void declare_column(const std::string &key)
static ::ExceptionBase & ExcColumnNotExistent(std::string arg1)
#define DEAL_II_NAMESPACE_CLOSE
void load(Archive &ar, const unsigned int version)
void get_selected_columns(std::vector< std::string > &sel_columns) const
void write_tex(std::ostream &file, const bool with_header=true) const
void serialize(Archive &ar, const unsigned int version)
T max(const T &t, const MPI_Comm &mpi_communicator)
void write_text(std::ostream &out, const TextOutputFormat format=table_with_headers) const
#define DEAL_II_DISABLE_EXTRA_DIAGNOSTICS
std::vector< std::string > column_order
void set_precision(const std::string &key, const unsigned int precision)
void serialize(Archive &archive, const unsigned int version)