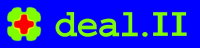 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_solver_h
17 #define dealii_solver_h
27 #include <boost/signals2.hpp>
33 template <
typename number>
332 template <
class VectorType = Vector<
double>>
393 boost::signals2::connection
396 const double check_value,
432 template <
typename Iterator>
458 boost::signals2::signal<
460 const double check_value,
475 template <
class VectorType = Vector<
double>>
482 template <
class VectorType>
498 template <
class VectorType>
499 template <
typename Iterator>
505 ExcMessage(
"You can't combine iterator states if no state is given."));
511 for (; p !=
end; ++p)
512 state = this->
operator()(state, *p);
518 template <
class VectorType>
528 connect([&solver_control](
const unsigned int iteration,
529 const double check_value,
531 return solver_control.
check(iteration, check_value);
537 template <
class VectorType>
540 memory(static_vector_memory)
546 connect([&solver_control](
const unsigned int iteration,
547 const double check_value,
549 return solver_control.
check(iteration, check_value);
555 template <
class VectorType>
556 inline boost::signals2::connection
559 const double check_value,
563 return iteration_status.connect(slot);
virtual State check(const unsigned int step, const double check_value)
GrowingVectorMemory< VectorType > static_vector_memory
@ iterate
Continue iteration.
MatrixTableIterators::Iterator< TransposeTable< T >, Constness, MatrixTableIterators::Storage::column_major > Iterator
static ::ExceptionBase & ExcMessage(std::string arg1)
VectorType::value_type * begin(VectorType &V)
#define DEAL_II_NAMESPACE_OPEN
VectorMemory< VectorType > & memory
VectorType::value_type * end(VectorType &V)
#define DEAL_II_DEPRECATED
boost::signals2::signal< SolverControl::State(const unsigned int iteration, const double check_value, const VectorType ¤t_iterate), StateCombiner > iteration_status
#define Assert(cond, exc)
boost::signals2::connection connect(const std::function< SolverControl::State(const unsigned int iteration, const double check_value, const VectorType ¤t_iterate)> &slot)
@ failure
Stop iteration, goal not reached.
@ success
Stop iteration, goal reached.
SolverBase(SolverControl &solver_control, VectorMemory< VectorType > &vector_memory)
#define DEAL_II_NAMESPACE_CLOSE
SolverControl::State operator()(const SolverControl::State state1, const SolverControl::State state2) const