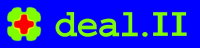 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_solver_control_h
17 #define dealii_solver_control_h
110 <<
"Iterative method reported convergence failure in step " <<
last_step
111 <<
". The residual in the last step was " <<
last_residual <<
".\n\n"
112 <<
"This error message can indicate that you have simply not allowed "
113 <<
"a sufficiently large number of iterations for your iterative solver "
114 <<
"to converge. This often happens when you increase the size of your "
115 <<
"problem. In such cases, the last residual will likely still be very "
116 <<
"small, and you can make the error go away by increasing the allowed "
117 <<
"number of iterations when setting up the SolverControl object that "
118 <<
"determines the maximal number of iterations you allow."
120 <<
"The other situation where this error may occur is when your matrix "
121 <<
"is not invertible (e.g., your matrix has a null-space), or if you "
122 <<
"try to apply the wrong solver to a matrix (e.g., using CG for a "
123 <<
"matrix that is not symmetric or not positive definite). In these "
124 <<
"cases, the residual in the last iteration is likely going to be large."
153 const double tol = 1.
e-10,
193 check(
const unsigned int step,
const double check_value);
268 const std::vector<double> &
478 check(
const unsigned int step,
const double check_value)
override;
555 check(
const unsigned int step,
const double check_value)
override;
612 check(
const unsigned int step,
const double check_value)
override;
unsigned int m_log_frequency
virtual State check(const unsigned int step, const double check_value)
virtual ~SolverControl() override=default
IterationNumberControl(const unsigned int maxiter=100, const double tolerance=1e-12, const bool log_history=false, const bool log_result=true)
double final_reduction() const
virtual State check(const unsigned int step, const double check_value) override
double set_reduction(const double)
void enable_history_data()
const std::vector< double > & get_history_data() const
const double last_residual
ReductionControl & operator=(const SolverControl &c)
static ::ExceptionBase & ExcHistoryDataRequired()
static void declare_parameters(ParameterHandler ¶m)
SymmetricTensor< 2, dim, Number > e(const Tensor< 2, dim, Number > &F)
double last_value() const
virtual ~IterationNumberControl() override=default
unsigned int n_converged_iterations
double average_reduction() const
NoConvergence(const unsigned int last_step, const double last_residual)
void clear_failure_criterion()
unsigned int last_step() const
@ iterate
Continue iteration.
virtual ~ReductionControl() override=default
SolverControl(const unsigned int n=100, const double tol=1.e-10, const bool log_history=false, const bool log_result=true)
unsigned int set_max_steps(const unsigned int)
unsigned int log_frequency(unsigned int)
virtual void print_info(std::ostream &out) const override
std::vector< double > history_data
virtual ~ConsecutiveControl() override=default
void parse_parameters(ParameterHandler ¶m)
void set_failure_criterion(const double rel_failure_residual)
virtual ~NoConvergence() noexcept override=default
#define DEAL_II_NAMESPACE_OPEN
double step_reduction(unsigned int step) const
bool history_data_enabled
ReductionControl(const unsigned int maxiter=100, const double tolerance=1.e-10, const double reduce=1.e-2, const bool log_history=false, const bool log_result=true)
IterationNumberControl & operator=(const SolverControl &c)
void parse_parameters(ParameterHandler ¶m)
unsigned int n_consecutive_iterations
const unsigned int last_step
ConsecutiveControl(const unsigned int maxiter=100, const double tolerance=1.e-10, const unsigned int n_consecutive_iterations=2, const bool log_history=false, const bool log_result=false)
#define DeclException0(Exception0)
@ failure
Stop iteration, goal not reached.
static void declare_parameters(ParameterHandler ¶m)
virtual State check(const unsigned int step, const double check_value) override
@ success
Stop iteration, goal reached.
ConsecutiveControl & operator=(const SolverControl &c)
double relative_failure_residual
unsigned int max_steps() const
#define DEAL_II_NAMESPACE_CLOSE
double set_tolerance(const double)
virtual State check(const unsigned int step, const double check_value) override
double initial_value() const