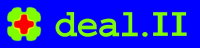 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
18 #ifdef DEAL_II_WITH_SLEPC
24 # include <petscversion.h>
26 # include <slepcversion.h>
37 , mpi_communicator(mpi_communicator)
38 , reason(EPS_CONVERGED_ITERATING)
46 ierr = EPSSetTolerances(
eps,
48 this->solver_control.max_steps());
65 const PetscErrorCode ierr = EPSDestroy(&
eps);
76 const PetscErrorCode ierr = EPSSetOperators(
eps,
A,
nullptr);
85 const PetscErrorCode ierr = EPSSetOperators(
eps,
A, B);
95 PetscErrorCode ierr = EPSSetST(
eps, transformation.
st);
98 # if DEAL_II_SLEPC_VERSION_GTE(3, 8, 0)
109 ierr = EPSSetTarget(
eps, sinv->additional_data.shift_parameter);
121 const PetscErrorCode ierr = EPSSetTarget(
eps, this_target);
129 const PetscErrorCode ierr = EPSSetWhichEigenpairs(
eps, eps_which);
136 const PetscErrorCode ierr = EPSSetProblemType(
eps, eps_problem);
144 PetscErrorCode ierr =
145 EPSSetDimensions(
eps, n_eigenpairs, PETSC_DECIDE, PETSC_DECIDE);
149 ierr = EPSSetFromOptions(
eps);
158 ierr = EPSSetConvergenceTest(
eps, EPS_CONV_ABS);
168 ierr = EPSSolve(
eps);
172 ierr = EPSGetConverged(
eps,
reinterpret_cast<PetscInt *
>(n_converged));
175 PetscInt n_iterations = 0;
176 PetscReal residual_norm = 0;
182 ierr = EPSGetIterationNumber(
eps, &n_iterations);
186 for (
unsigned int i = 0; i < *n_converged; i++)
188 double residual_norm_i = 0.0;
207 # if DEAL_II_SLEPC_VERSION_GTE(3, 6, 0)
208 ierr = EPSComputeError(
eps, i, EPS_ERROR_ABSOLUTE, &residual_norm_i);
210 ierr = EPSComputeResidualNorm(
eps, i, &residual_norm_i);
214 residual_norm =
std::max(residual_norm, residual_norm_i);
240 const PetscErrorCode ierr =
248 double & real_eigenvalues,
249 double & imag_eigenvalues,
253 # ifndef PETSC_USE_COMPLEX
255 const PetscErrorCode ierr = EPSGetEigenpair(
eps,
266 "Your PETSc/SLEPc installation was configured with scalar-type complex "
267 "but this function is not defined for complex types."));
271 (void)real_eigenvalues;
272 (void)imag_eigenvalues;
273 (void)real_eigenvectors;
274 (void)imag_eigenvectors;
283 case ::SolverControl::iterate:
284 reason = EPS_CONVERGED_ITERATING;
287 case ::SolverControl::success:
288 reason =
static_cast<EPSConvergedReason
>(1);
291 case ::SolverControl::failure:
293 reason = EPS_DIVERGED_ITS;
295 reason = EPS_DIVERGED_BREAKDOWN;
332 , additional_data(data)
334 const PetscErrorCode ierr =
335 EPSSetType(
eps,
const_cast<char *
>(EPSKRYLOVSCHUR));
341 const bool delayed_reorthogonalization)
342 : delayed_reorthogonalization(delayed_reorthogonalization)
351 PetscErrorCode ierr = EPSSetType(
eps,
const_cast<char *
>(EPSARNOLDI));
358 ierr = EPSArnoldiSetDelayed(
eps, PETSC_TRUE);
375 PetscErrorCode ierr = EPSSetType(
eps,
const_cast<char *
>(EPSLANCZOS));
387 , additional_data(data)
389 PetscErrorCode ierr = EPSSetType(
eps,
const_cast<char *
>(EPSPOWER));
395 bool double_expansion)
396 : double_expansion(double_expansion)
406 PetscErrorCode ierr = EPSSetType(
eps,
const_cast<char *
>(EPSGD));
411 ierr = EPSGDSetDoubleExpansion(
eps, PETSC_TRUE);
421 , additional_data(data)
423 const PetscErrorCode ierr = EPSSetType(
eps,
const_cast<char *
>(EPSJD));
432 , additional_data(data)
436 # if PETSC_HAVE_BLASLAPACK
437 const PetscErrorCode ierr = EPSSetType(
eps,
const_cast<char *
>(EPSLAPACK));
443 "Your PETSc/SLEPc installation was not configured with BLAS/LAPACK "
444 "but this is needed to use the LAPACK solver."));
451 #endif // DEAL_II_WITH_SLEPC
virtual State check(const unsigned int step, const double check_value)
void set_matrices(const PETScWrappers::MatrixBase &A)
SolverLanczos(SolverControl &cn, const MPI_Comm &mpi_communicator=PETSC_COMM_SELF, const AdditionalData &data=AdditionalData())
SolverGeneralizedDavidson(SolverControl &cn, const MPI_Comm &mpi_communicator=PETSC_COMM_SELF, const AdditionalData &data=AdditionalData())
static int convergence_test(EPS eps, PetscScalar real_eigenvalue, PetscScalar imag_eigenvalue, PetscReal residual_norm, PetscReal *estimated_error, void *solver_control)
SolverControl & control() const
static ::ExceptionBase & ExcNotImplemented()
static ::ExceptionBase & ExcSLEPcError(int arg1)
void set_transformation(SLEPcWrappers::TransformationBase &this_transformation)
void get_solver_state(const SolverControl::State state)
const AdditionalData additional_data
SolverControl & solver_control
AdditionalData(bool double_expansion=false)
const AdditionalData additional_data
unsigned int last_step() const
SolverKrylovSchur(SolverControl &cn, const MPI_Comm &mpi_communicator=PETSC_COMM_SELF, const AdditionalData &data=AdditionalData())
const AdditionalData additional_data
void solve(const PETScWrappers::MatrixBase &A, std::vector< PetscScalar > &eigenvalues, std::vector< OutputVector > &eigenvectors, const unsigned int n_eigenpairs=1)
bool delayed_reorthogonalization
static ::ExceptionBase & ExcMessage(std::string arg1)
@ eigenvalues
Eigenvalue vector is filled.
#define DEAL_II_NAMESPACE_OPEN
SolverArnoldi(SolverControl &cn, const MPI_Comm &mpi_communicator=PETSC_COMM_SELF, const AdditionalData &data=AdditionalData())
AdditionalData(const EPSLanczosReorthogType r=EPS_LANCZOS_REORTHOG_FULL)
void set_which_eigenpairs(EPSWhich set_which)
EPSConvergedReason reason
#define Assert(cond, exc)
void get_eigenpair(const unsigned int index, PetscScalar &eigenvalues, PETScWrappers::VectorBase &eigenvectors)
const MPI_Comm mpi_communicator
void set_target_eigenvalue(const PetscScalar &this_target)
#define AssertNothrow(cond, exc)
unsigned int max_steps() const
SolverJacobiDavidson(SolverControl &cn, const MPI_Comm &mpi_communicator=PETSC_COMM_SELF, const AdditionalData &data=AdditionalData())
SolverLAPACK(SolverControl &cn, const MPI_Comm &mpi_communicator=PETSC_COMM_SELF, const AdditionalData &data=AdditionalData())
#define DEAL_II_NAMESPACE_CLOSE
void set_problem_type(EPSProblemType set_problem)
SolverPower(SolverControl &cn, const MPI_Comm &mpi_communicator=PETSC_COMM_SELF, const AdditionalData &data=AdditionalData())
#define AssertThrow(cond, exc)
AdditionalData(const bool delayed_reorthogonalization=false)
T max(const T &t, const MPI_Comm &mpi_communicator)
EPSLanczosReorthogType reorthog
SolverBase(SolverControl &cn, const MPI_Comm &mpi_communicator)
std::array< std::pair< Number, Tensor< 1, dim, Number > >, std::integral_constant< int, dim >::value > eigenvectors(const SymmetricTensor< 2, dim, Number > &T, const SymmetricTensorEigenvectorMethod method=SymmetricTensorEigenvectorMethod::ql_implicit_shifts)