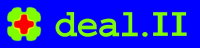 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
17 #ifndef dealii_point_value_history_h
18 #define dealii_point_value_history_h
54 namespace PointValueHistoryImplementation
67 const std::vector<types::global_dof_index> &new_sol_indices);
239 const unsigned int n_independent_variables = 0);
317 const std::vector<std::string> &component_names);
336 template <
class VectorType>
358 template <
class VectorType>
370 template <
class VectorType>
390 template <
class VectorType>
437 const std::vector<
Point<dim>> &postprocessor_locations =
535 status(std::ostream &out);
553 "A call has been made to push_back_independent() when "
554 "no independent values were requested.");
561 "This error is thrown to indicate that the data sets appear to be out of "
562 "sync. The class requires that the number of dataset keys is the same as "
563 "the number of independent values sets and mesh linked value sets. The "
564 "number of each of these is allowed to differ by one to allow new values "
565 "to be added with out restricting the order the user choses to do so. "
566 "Special cases of no FHandler and no independent values should not "
567 "trigger this error.");
575 "A method which requires access to a @p DoFHandler to be meaningful has "
576 "been called when have_dof_handler is false (most likely due to default "
577 "constructor being called). Only independent variables may be logged with "
585 "The triangulation has been refined or coarsened in some way. This "
586 "suggests that the internal DoF indices stored by the current "
587 "object are no longer meaningful.");
615 std::map<std::string, std::vector<std::vector<double>>>
data_store;
632 std::vector<internal::PointValueHistoryImplementation::PointGeometryData<dim>>
#define DeclExceptionMsg(Exception, defaulttext)
PointValueHistory(const unsigned int n_independent_variables=0)
static ::ExceptionBase & ExcNoIndependent()
std::vector< std::vector< double > > independent_values
std::vector< double > dataset_key
void add_points(const std::vector< Point< dim >> &locations)
std::vector< types::global_dof_index > solution_indices
std::vector< Point< dim > > support_point_locations
std::vector< std::string > indep_names
void add_field_name(const std::string &vector_name, const ComponentMask &component_mask=ComponentMask())
void get_support_locations(std::vector< std::vector< Point< dim >>> &locations)
static ::ExceptionBase & ExcDoFHandlerRequired()
void evaluate_field(const std::string &name, const VectorType &solution)
void start_new_dataset(const double key)
SmartPointer< const DoFHandler< dim >, PointValueHistory< dim > > dof_handler
static ::ExceptionBase & ExcDoFHandlerChanged()
#define DEAL_II_NAMESPACE_OPEN
PointValueHistory & operator=(const PointValueHistory &point_value_history)
void add_component_names(const std::string &vector_name, const std::vector< std::string > &component_names)
void add_independent_names(const std::vector< std::string > &independent_names)
boost::signals2::connection tria_listener
void evaluate_field_at_requested_location(const std::string &name, const VectorType &solution)
Vector< double > mark_support_locations()
#define DEAL_II_DEPRECATED
void get_postprocessor_locations(const Quadrature< dim > &quadrature, std::vector< Point< dim >> &locations)
static ::ExceptionBase & ExcDataLostSync()
void write_gnuplot(const std::string &base_name, const std::vector< Point< dim >> &postprocessor_locations=std::vector< Point< dim >>())
std::map< std::string, std::vector< std::vector< double > > > data_store
bool triangulation_changed
std::map< std::string, ComponentMask > component_mask
void add_point(const Point< dim > &location)
void tria_change_listener()
void status(std::ostream &out)
void get_points(std::vector< std::vector< Point< dim >>> &locations)
std::map< std::string, std::vector< std::string > > component_names_map
PointGeometryData(const Point< dim > &new_requested_location, const std::vector< Point< dim >> &new_locations, const std::vector< types::global_dof_index > &new_sol_indices)
Only a constructor needed for this class (a struct really)
bool deep_check(const bool strict)
#define DEAL_II_NAMESPACE_CLOSE
Point< dim > requested_location
void push_back_independent(const std::vector< double > &independent_values)
std::vector< internal::PointValueHistoryImplementation::PointGeometryData< dim > > point_geometry_data