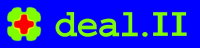 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_mg_matrix_h
17 #define dealii_mg_matrix_h
46 template <
typename VectorType = Vector<
double>>
59 template <
typename MatrixType>
66 template <
typename MatrixType>
125 template <
typename MatrixType,
typename number>
134 const unsigned int col = 0,
204 template <
typename VectorType>
205 template <
typename MatrixType>
222 template <
typename VectorType>
226 matrices.resize(0, 0);
231 template <
typename VectorType>
232 template <
typename MatrixType>
240 template <
typename VectorType>
244 return matrices[
level];
249 template <
typename VectorType>
255 matrices[
level].vmult(dst, src);
260 template <
typename VectorType>
266 matrices[
level].vmult_add(dst, src);
271 template <
typename VectorType>
277 matrices[
level].Tvmult(dst, src);
282 template <
typename VectorType>
288 matrices[
level].Tvmult_add(dst, src);
293 template <
typename VectorType>
297 return matrices.min_level();
302 template <
typename VectorType>
306 return matrices.max_level();
311 template <
typename VectorType>
315 return sizeof(*this) + matrices->memory_consumption();
322 template <
typename MatrixType,
typename number>
324 const unsigned int col,
326 :
matrix(p, typeid(*this).name())
333 template <
typename MatrixType,
typename number>
342 template <
typename MatrixType,
typename number>
345 const unsigned int bcol)
353 template <
typename MatrixType,
typename number>
362 m[
level].block(row, col).vmult(dst, src);
367 template <
typename MatrixType,
typename number>
376 m[
level].block(row, col).vmult_add(dst, src);
381 template <
typename MatrixType,
typename number>
390 m[
level].block(row, col).Tvmult(dst, src);
395 template <
typename MatrixType,
typename number>
404 m[
level].block(row, col).Tvmult_add(dst, src);
virtual unsigned int get_minlevel() const override
virtual void Tvmult(const unsigned int level, Vector< number > &dst, const Vector< number > &src) const
virtual void vmult(const unsigned int level, Vector< number > &dst, const Vector< number > &src) const
unsigned int max_level() const
virtual void vmult_add(const unsigned int level, VectorType &dst, const VectorType &src) const override
virtual void Tvmult_add(const unsigned int level, VectorType &dst, const VectorType &src) const override
unsigned int min_level() const
void set_matrix(MGLevelObject< MatrixType > *M)
SmartPointer< MGLevelObject< MatrixType >, MGMatrixSelect< MatrixType, number > > matrix
const LinearOperator< VectorType > & operator[](unsigned int level) const
MGLevelObject< LinearOperator< VectorType > > matrices
void select_block(const unsigned int row, const unsigned int col)
virtual void Tvmult(const unsigned int level, VectorType &dst, const VectorType &src) const override
void initialize(const MGLevelObject< MatrixType > &M)
#define DEAL_II_NAMESPACE_OPEN
@ matrix
Contents is actually a matrix.
virtual void Tvmult_add(const unsigned int level, Vector< number > &dst, const Vector< number > &src) const
virtual unsigned int get_maxlevel() const override
static ::ExceptionBase & ExcNotInitialized()
virtual void vmult(const unsigned int level, VectorType &dst, const VectorType &src) const override
#define Assert(cond, exc)
virtual void vmult_add(const unsigned int level, Vector< number > &dst, const Vector< number > &src) const
std::size_t memory_consumption() const
#define DEAL_II_NAMESPACE_CLOSE
MGMatrixSelect(const unsigned int row=0, const unsigned int col=0, MGLevelObject< MatrixType > *matrix=0)