Loading [MathJax]/extensions/TeX/newcommand.js
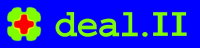 |
Reference documentation for deal.II version 9.2.0
|
\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}
\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}
\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}
\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}
Go to the documentation of this file.
16 #ifndef dealii_mg_level_object_h
17 #define dealii_mg_level_object_h
49 template <
class Object>
73 const unsigned int maxlevel = 0);
100 resize(
const unsigned int new_minlevel,
const unsigned int new_maxlevel);
144 template <
typename ActionFunctionObjectType>
146 apply(ActionFunctionObjectType action);
170 template <
class Object>
172 const unsigned int max)
179 template <
class Object>
182 Assert((i >= minlevel) && (i < minlevel + objects.size()),
184 return *objects[i - minlevel];
188 template <
class Object>
191 Assert((i >= minlevel) && (i < minlevel + objects.size()),
193 return *objects[i - minlevel];
197 template <
class Object>
200 const unsigned int new_maxlevel)
209 minlevel = new_minlevel;
210 for (
unsigned int i = 0; i < new_maxlevel - new_minlevel + 1; ++i)
211 objects.push_back(std::make_shared<Object>());
215 template <
class Object>
219 typename std::vector<std::shared_ptr<Object>>::iterator v;
220 for (v = objects.begin(); v != objects.end(); ++v)
226 template <
class Object>
230 typename std::vector<std::shared_ptr<Object>>::iterator v;
231 for (v = objects.begin(); v != objects.end(); ++v)
236 template <
class Object>
244 template <
class Object>
248 return minlevel + objects.size() - 1;
251 template <
class Object>
252 template <
typename ActionFunctionObjectType>
256 for (
unsigned int lvl = min_level(); lvl <= max_level(); ++lvl)
258 action(lvl, (*
this)[lvl]);
263 template <
class Object>
267 std::size_t result =
sizeof(*this);
268 using Iter =
typename std::vector<std::shared_ptr<Object>>::const_iterator;
269 const Iter
end = objects.end();
270 for (Iter o = objects.begin(); o !=
end; ++o)
271 result += (*o)->memory_consumption();
MGLevelObject< Object > & operator=(const double d)
unsigned int max_level() const
unsigned int min_level() const
VectorizedArray< Number, width > max(const ::VectorizedArray< Number, width > &x, const ::VectorizedArray< Number, width > &y)
VectorizedArray< Number, width > min(const ::VectorizedArray< Number, width > &x, const ::VectorizedArray< Number, width > &y)
std::vector< std::shared_ptr< Object > > objects
MGLevelObject(const unsigned int minlevel=0, const unsigned int maxlevel=0)
SymmetricTensor< 2, dim, Number > d(const Tensor< 2, dim, Number > &F, const Tensor< 2, dim, Number > &dF_dt)
Object & operator[](const unsigned int level)
static ::ExceptionBase & ExcIndexRange(int arg1, int arg2, int arg3)
#define DEAL_II_NAMESPACE_OPEN
VectorType::value_type * end(VectorType &V)
static ::ExceptionBase & ExcInternalError()
void resize(const unsigned int new_minlevel, const unsigned int new_maxlevel)
#define Assert(cond, exc)
std::size_t memory_consumption() const
T min(const T &t, const MPI_Comm &mpi_communicator)
#define DEAL_II_NAMESPACE_CLOSE
void apply(ActionFunctionObjectType action)
T max(const T &t, const MPI_Comm &mpi_communicator)