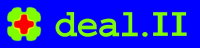 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
17 #ifndef dealii_mesh_worker_dof_info_h
18 #define dealii_mesh_worker_dof_info_h
39 template <
int dim,
class DOFINFO>
75 template <
int dim,
int spacedim = dim,
typename number =
double>
127 template <
class DHCellIterator>
129 reinit(
const DHCellIterator &c);
134 template <
class DHCellIterator,
class DHFaceIterator>
136 reinit(
const DHCellIterator &c,
137 const DHFaceIterator &f,
138 const unsigned int face_no);
143 template <
class DHCellIterator,
class DHFaceIterator>
145 reinit(
const DHCellIterator &c,
146 const DHFaceIterator &f,
147 const unsigned int face_no,
148 const unsigned int subface_no);
154 template <
class DHFaceIterator>
156 set_face(
const DHFaceIterator &f,
const unsigned int face_no);
162 template <
class DHFaceIterator>
165 const unsigned int face_no,
166 const unsigned int subface_no);
193 template <
class DHCellIterator>
222 template <
int dim,
class DOFINFO>
254 template <
class ASSEMBLER>
292 template <
int dim,
int spacedim,
typename number>
301 template <
int dim,
int spacedim,
typename number>
308 std::vector<types::global_dof_index> aux(1);
309 aux[0] = dof_handler.
get_fe().dofs_per_cell;
314 template <
int dim,
int spacedim,
typename number>
315 template <
class DHCellIterator>
319 indices.resize(c->get_fe().dofs_per_cell);
320 if (block_info ==
nullptr || block_info->local().size() == 0)
321 c->get_active_or_mg_dof_indices(indices);
324 indices_org.resize(c->get_fe().dofs_per_cell);
325 c->get_active_or_mg_dof_indices(indices_org);
331 template <
int dim,
int spacedim,
typename number>
332 template <
class DHCellIterator>
337 level_cell = c->is_level_cell();
349 template <
int dim,
int spacedim,
typename number>
350 template <
class DHFaceIterator>
353 const unsigned int face_no)
356 face_number = face_no;
361 template <
int dim,
int spacedim,
typename number>
362 template <
class DHCellIterator,
class DHFaceIterator>
365 const DHFaceIterator &f,
366 const unsigned int face_no)
371 level_cell = c->is_level_cell();
374 set_face(f, face_no);
383 template <
int dim,
int spacedim,
typename number>
384 template <
class DHFaceIterator>
387 const unsigned int face_no,
388 const unsigned int subface_no)
391 face_number = face_no;
392 sub_number = subface_no;
396 template <
int dim,
int spacedim,
typename number>
397 template <
class DHCellIterator,
class DHFaceIterator>
400 const DHFaceIterator &f,
401 const unsigned int face_no,
402 const unsigned int subface_no)
408 level_cell = c->is_level_cell();
411 set_subface(f, face_no, subface_no);
420 template <
int dim,
int spacedim,
typename number>
425 return block_info->local();
426 return aux_local_indices;
431 template <
int dim,
class DOFINFO>
446 template <
int dim,
class DOFINFO>
450 , cell_valid(other.cell_valid)
462 template <
int dim,
class DOFINFO>
472 interior_face_available[i] =
false;
473 exterior_face_available[i] =
false;
479 template <
int dim,
class DOFINFO>
486 interior_face_available[i] =
false;
487 exterior_face_available[i] =
false;
492 template <
int dim,
class DOFINFO>
493 template <
class ASSEMBLER>
500 assembler.assemble(cell);
504 if (interior_face_available[i])
509 if (exterior_face_available[i])
510 assembler.assemble(interior[i], exterior[i]);
512 assembler.assemble(interior[i]);
bool interior_face_available[GeometryInfo< dim >::faces_per_cell]
void set_block_indices()
Set up local block indices.
DoFInfoBox(const DOFINFO &seed)
void reinit(const DHCellIterator &c)
void set_subface(const DHFaceIterator &f, const unsigned int face_no, const unsigned int subface_no)
DOFINFO interior[GeometryInfo< dim >::faces_per_cell]
SmartPointer< const BlockInfo, DoFInfo< dim, spacedim > > block_info
The block structure of the system.
@ valid
Iterator points to a valid object.
DOFINFO exterior[GeometryInfo< dim >::faces_per_cell]
BlockIndices aux_local_indices
const BlockIndices & local_indices() const
#define DEAL_II_NAMESPACE_OPEN
DoFInfoBox & operator=(const DoFInfoBox< dim, DOFINFO > &)
std::vector< types::global_dof_index > indices
TriaIterator< CellAccessor< dim, spacedim > > cell_iterator
void assemble(ASSEMBLER &ass) const
void set_face(const DHFaceIterator &f, const unsigned int face_no)
void reinit(const unsigned int n_blocks, const size_type n_elements_per_block)
void reinit(const BlockIndices &local_sizes)
void get_indices(const DHCellIterator &c)
Fill index vector with active indices.
static const unsigned int invalid_unsigned_int
std::vector< types::global_dof_index > indices_org
Auxiliary vector.
bool exterior_face_available[GeometryInfo< dim >::faces_per_cell]
A small class collecting the different BlockIndices involved in global, multilevel and local computat...
std::vector< std::vector< types::global_dof_index > > indices_by_block
#define DEAL_II_NAMESPACE_CLOSE
const FiniteElement< dim, spacedim > & get_fe(const unsigned int index=0) const
Triangulation< dim, spacedim >::face_iterator face
The current face.
Triangulation< dim, spacedim >::cell_iterator cell
The current cell.