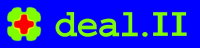 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
17 #ifndef dealii_mesh_worker_local_results_h
18 #define dealii_mesh_worker_local_results_h
225 template <
typename number>
268 value(
const unsigned int i);
274 value(
const unsigned int i)
const;
280 vector(
const unsigned int i);
286 vector(
const unsigned int i)
const;
295 matrix(
const unsigned int i,
const bool external =
false);
304 matrix(
const unsigned int i,
const bool external =
false)
const;
359 template <
typename MatrixType>
371 template <
typename MatrixType>
391 template <
class StreamType>
405 std::vector<number>
J;
411 std::vector<BlockVector<number>>
R;
417 std::vector<MatrixBlock<FullMatrix<number>>>
M1;
425 std::vector<MatrixBlock<FullMatrix<number>>>
M2;
435 template <
typename number>
443 template <
typename number>
451 template <
typename number>
452 template <
typename MatrixType>
458 M1.resize(matrices.
size());
460 M2.resize(matrices.
size());
461 for (
unsigned int i = 0; i < matrices.
size(); ++i)
463 const unsigned int row = matrices.
block(i).
row;
477 template <
typename number>
478 template <
typename MatrixType>
484 M1.resize(matrices.
size());
486 M2.resize(matrices.
size());
487 for (
unsigned int i = 0; i < matrices.
size(); ++i)
490 const unsigned int row = o[o.
min_level()].row;
491 const unsigned int col = o[o.
min_level()].column;
504 template <
typename number>
512 for (
unsigned int i = 0; i < n; ++i)
525 template <
typename number>
528 const unsigned int nv)
530 quadrature_data.reinit(np, nv);
534 template <
typename number>
542 template <
typename number>
550 template <
typename number>
558 template <
typename number>
562 return quadrature_data.n_rows();
566 template <
typename number>
570 return quadrature_data.n_cols();
574 template <
typename number>
583 template <
typename number>
592 template <
typename number>
606 template <
typename number>
609 const unsigned int i)
611 return quadrature_data(k, i);
615 template <
typename number>
619 return quadrature_data;
623 template <
typename number>
632 template <
typename number>
641 template <
typename number>
655 template <
typename number>
658 const unsigned int i)
const
660 return quadrature_data(k, i);
664 template <
typename number>
665 template <
class StreamType>
669 os <<
"J: " << J.size() << std::endl;
670 os <<
"R: " << R.size() << std::endl;
671 for (
unsigned int i = 0; i < R.size(); ++i)
673 os <<
" " << R[i].n_blocks() <<
" -";
674 for (
unsigned int j = 0; j < R[i].n_blocks(); ++j)
675 os <<
' ' << R[i].block(j).size();
678 os <<
"M: " << M1.size() <<
" face " << M2.size() << std::endl;
679 for (
unsigned int i = 0; i < M1.size(); ++i)
681 os <<
" " << M1[i].row <<
"," << M1[i].column <<
" "
682 << M1[i].matrix.m() <<
'x' << M1[i].matrix.n();
684 os <<
" face " << M2[i].row <<
"," << M2[i].column <<
" "
685 << M2[i].matrix.m() <<
'x' << M2[i].matrix.n();
std::vector< BlockVector< number > > R
number & value(const unsigned int i)
void initialize_vectors(const unsigned int n)
std::vector< MatrixBlock< FullMatrix< number > > > M2
unsigned int n_matrices() const
unsigned int min_level() const
unsigned int size() const
Number of stored data objects.
void print_debug(StreamType &os) const
#define AssertIndexRange(index, range)
void initialize_quadrature(const unsigned int np, const unsigned int nv)
void initialize_matrices(const unsigned int n, bool both)
unsigned int n_values() const
std::vector< MatrixBlock< FullMatrix< number > > > M1
unsigned int n_vectors() const
const value_type & block(size_type i) const
#define DEAL_II_NAMESPACE_OPEN
BlockVector< number > & vector(const unsigned int i)
number & quadrature_value(const unsigned int k, const unsigned int i)
Table< 2, number > quadrature_data
MatrixBlock< FullMatrix< number > > & matrix(const unsigned int i, const bool external=false)
unsigned int n_quadrature_values() const
Table< 2, number > & quadrature_values()
void reinit(const BlockIndices &local_sizes)
void initialize_numbers(const unsigned int n)
std::size_t memory_consumption() const
unsigned int size() const
#define DEAL_II_NAMESPACE_CLOSE
unsigned int n_quadrature_points() const
const value_type & block(size_type i) const