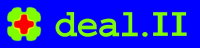 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_lapack_full_matrix_h
17 #define dealii_lapack_full_matrix_h
37 template <
typename number>
39 template <
typename number>
41 template <
typename number>
43 template <
typename number>
59 template <
typename number>
66 using size_type = std::make_unsigned<types::blas_int>::type;
110 template <
typename number2>
120 template <
typename number2>
197 const bool left =
true);
205 template <
typename MatrixType>
307 template <
typename MatrixType>
309 fill(
const MatrixType &src,
314 const number factor = 1.,
345 template <
typename number2>
347 vmult(Vector<number2> &
w,
348 const Vector<number2> &v,
349 const bool adding =
false)
const;
357 const bool adding =
false)
const;
365 template <
typename number2>
367 vmult_add(Vector<number2> &
w,
const Vector<number2> &v)
const;
386 template <
typename number2>
389 const Vector<number2> &v,
390 const bool adding =
false)
const;
398 const bool adding =
false)
const;
406 template <
typename number2>
408 Tvmult_add(Vector<number2> &
w,
const Vector<number2> &v)
const;
434 const bool adding =
false)
const;
443 const bool adding =
false)
const;
462 const bool adding =
false)
const;
471 const bool adding =
false)
const;
492 const bool adding =
false)
const;
511 const bool adding =
false)
const;
520 const bool adding =
false)
const;
540 const bool adding =
false)
const;
549 const bool adding =
false)
const;
697 const bool left_eigenvectors =
false);
720 const number upper_bound,
721 const number abs_accuracy,
755 const number upper_bound,
756 const number abs_accuracy,
890 const unsigned int precision = 3,
891 const bool scientific =
true,
892 const unsigned int width = 0,
893 const char * zero_string =
" ",
894 const double denominator = 1.,
895 const double threshold = 0.)
const;
902 norm(
const char type)
const;
919 mutable std::vector<number>
work;
924 mutable std::vector<types::blas_int>
iwork;
932 std::vector<types::blas_int>
ipiv;
943 std::vector<typename numbers::NumberTraits<number>::real_type>
wr;
949 std::vector<number>
wi;
954 std::vector<number>
vl;
959 std::vector<number>
vr;
965 std::unique_ptr<LAPACKFullMatrix<number>>
svd_u;
971 std::unique_ptr<LAPACKFullMatrix<number>>
svd_vt;
987 template <
typename number>
1011 template <
typename number>
1017 (*this)(i, j) =
value;
1021 template <
typename number>
1025 return static_cast<size_type>(this->n_rows());
1028 template <
typename number>
1032 return static_cast<size_type>(this->n_cols());
1035 template <
typename number>
1036 template <
typename MatrixType>
1040 this->
reinit(M.m(), M.n());
1045 for (
size_type row = 0; row < M.m(); ++row)
1047 const typename MatrixType::const_iterator end_row = M.end(row);
1048 for (
typename MatrixType::const_iterator entry = M.begin(row);
1051 this->el(row, entry->column()) = entry->value();
1059 template <
typename number>
1060 template <
typename MatrixType>
1067 const number factor,
1072 for (
size_type row = src_offset_i; row < M.m(); ++row)
1074 const typename MatrixType::const_iterator end_row = M.end(row);
1075 for (
typename MatrixType::const_iterator entry = M.begin(row);
1082 const size_type dst_i = dst_offset_i + i - src_offset_i;
1083 const size_type dst_j = dst_offset_j + j - src_offset_j;
1084 if (dst_i < this->n_rows() && dst_j < this->n_cols())
1085 (*this)(dst_i, dst_j) = factor * entry->value();
1093 template <
typename number>
1094 template <
typename number2>
1097 const Vector<number2> &,
1101 ExcMessage(
"LAPACKFullMatrix<number>::vmult must be called with a "
1102 "matching Vector<double> vector type."));
1106 template <
typename number>
1107 template <
typename number2>
1110 const Vector<number2> &)
const
1113 ExcMessage(
"LAPACKFullMatrix<number>::vmult_add must be called with a "
1114 "matching Vector<double> vector type."));
1118 template <
typename number>
1119 template <
typename number2>
1122 const Vector<number2> &,
1126 ExcMessage(
"LAPACKFullMatrix<number>::Tvmult must be called with a "
1127 "matching Vector<double> vector type."));
1131 template <
typename number>
1132 template <
typename number2>
1135 const Vector<number2> &)
const
1139 "LAPACKFullMatrix<number>::Tvmult_add must be called with a "
1140 "matching Vector<double> vector type."));
1144 template <
typename number>
1145 inline std::complex<number>
1154 return std::complex<number>(wi[i]);
1156 return std::complex<number>(wr[i], wi[i]);
1160 template <
typename number>
1172 template <
typename number>
1183 template <
typename number>
@ svd
Matrix contains singular value decomposition,.
LAPACKFullMatrix< number > & operator*=(const number factor)
std::make_unsigned< types::blas_int >::type size_type
void copy_from(const MatrixType &)
void vmult(Vector< number2 > &w, const Vector< number2 > &v, const bool adding=false) const
std::unique_ptr< LAPACKFullMatrix< number > > svd_u
void compute_generalized_eigenvalues_symmetric(LAPACKFullMatrix< number > &B, const number lower_bound, const number upper_bound, const number abs_accuracy, Vector< number > &eigenvalues, std::vector< Vector< number >> &eigenvectors, const types::blas_int itype=1)
@ inverse_svd
Matrix is the inverse of a singular value decomposition.
void set(const size_type i, const size_type j, const number value)
std::vector< typename numbers::NumberTraits< number >::real_type > wr
static ::ExceptionBase & ExcInvalidState()
void solve(Vector< number > &v, const bool transposed=false) const
void compute_lu_factorization()
void compute_inverse_svd(const double threshold=0.)
number frobenius_norm() const
const LAPACKFullMatrix< number > & get_svd_vt() const
number reciprocal_condition_number() const
#define AssertIndexRange(index, range)
SymmetricTensor< 2, dim, Number > C(const Tensor< 2, dim, Number > &F)
void grow_or_shrink(const size_type size)
SymmetricTensor< 2, dim, Number > d(const Tensor< 2, dim, Number > &F, const Tensor< 2, dim, Number > &dF_dt)
void add(const number a, const LAPACKFullMatrix< number > &B)
std::vector< number > inv_work
std::unique_ptr< LAPACKFullMatrix< number > > svd_vt
LAPACKSupport::Property property
void fill(const MatrixType &src, const size_type dst_offset_i=0, const size_type dst_offset_j=0, const size_type src_offset_i=0, const size_type src_offset_j=0, const number factor=1., const bool transpose=false)
number singular_value(const size_type i) const
number determinant() const
void transpose(LAPACKFullMatrix< number > &B) const
Tensor< 2, dim, Number > w(const Tensor< 2, dim, Number > &F, const Tensor< 2, dim, Number > &dF_dt)
void Tvmult_add(Vector< number2 > &w, const Vector< number2 > &v) const
void set_property(const LAPACKSupport::Property property)
static ::ExceptionBase & ExcMessage(std::string arg1)
@ eigenvalues
Eigenvalue vector is filled.
typename AlignedVector< Number >::size_type size_type
void reinit(MatrixBlock< MatrixType > &v, const BlockSparsityPattern &p)
void vmult(Vector< number > &, const Vector< number > &) const
const LAPACKFullMatrix< number > & get_svd_u() const
LAPACKFullMatrix(const size_type size=0)
void Tmmult(LAPACKFullMatrix< number > &C, const LAPACKFullMatrix< number > &B, const bool adding=false) const
Iterator lower_bound(Iterator first, Iterator last, const T &val)
#define DEAL_II_NAMESPACE_OPEN
LAPACKFullMatrix< number > & operator/=(const number factor)
@ matrix
Contents is actually a matrix.
number norm(const char type) const
LAPACKSupport::State state
void compute_eigenvalues(const bool right_eigenvectors=false, const bool left_eigenvectors=false)
void Tvmult(Vector< number > &, const Vector< number > &) const
std::complex< number > eigenvalue(const size_type i) const
void rank1_update(const number a, const Vector< number > &v)
void compute_eigenvalues_symmetric(const number lower_bound, const number upper_bound, const number abs_accuracy, Vector< number > &eigenvalues, FullMatrix< number > &eigenvectors)
void reinit(const size_type size)
static ::ExceptionBase & ExcInternalError()
void apply_givens_rotation(const std::array< number, 3 > &csr, const size_type i, const size_type k, const bool left=true)
std::vector< number > work
void vmult_add(Vector< number2 > &w, const Vector< number2 > &v) const
#define Assert(cond, exc)
SmartPointer< const LAPACKFullMatrix< number >, PreconditionLU< number > > matrix
std::vector< types::blas_int > iwork
void TmTmult(LAPACKFullMatrix< number > &C, const LAPACKFullMatrix< number > &B, const bool adding=false) const
void Tvmult(Vector< number2 > &w, const Vector< number2 > &v, const bool adding=false) const
void print_formatted(std::ostream &out, const unsigned int precision=3, const bool scientific=true, const unsigned int width=0, const char *zero_string=" ", const double denominator=1., const double threshold=0.) const
void mTmult(LAPACKFullMatrix< number > &C, const LAPACKFullMatrix< number > &B, const bool adding=false) const
void mmult(LAPACKFullMatrix< number > &C, const LAPACKFullMatrix< number > &B, const bool adding=false) const
void initialize(const LAPACKFullMatrix< number > &)
void remove_row_and_column(const size_type row, const size_type col)
LAPACKFullMatrix< number > & operator=(const LAPACKFullMatrix< number > &)
const TableIndices< N > & size() const
#define DEAL_II_NAMESPACE_CLOSE
static ::ExceptionBase & ExcState(State arg1)
std::vector< types::blas_int > ipiv
void compute_inverse_svd_with_kernel(const unsigned int kernel_size)
number linfty_norm() const
void compute_cholesky_factorization()
void scale_rows(const Vector< number > &V)
std::array< std::pair< Number, Tensor< 1, dim, Number > >, std::integral_constant< int, dim >::value > eigenvectors(const SymmetricTensor< 2, dim, Number > &T, const SymmetricTensorEigenvectorMethod method=SymmetricTensorEigenvectorMethod::ql_implicit_shifts)
SmartPointer< VectorMemory< Vector< number > >, PreconditionLU< number > > mem