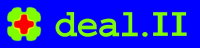 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_grid_refinement_h
17 #define dealii_grid_refinement_h
30 template <
int dim,
int spacedim>
32 template <
typename Number>
88 std::pair<double, double>
92 const double top_fraction_of_cells,
93 const double bottom_fraction_of_cells);
159 template <
int dim,
typename Number,
int spacedim>
163 const Vector<Number> & criteria,
164 const double top_fraction_of_cells,
165 const double bottom_fraction_of_cells,
224 template <
int dim,
typename Number,
int spacedim>
228 const Vector<Number> & criteria,
229 const double top_fraction,
230 const double bottom_fraction,
308 template <
int dim,
typename Number,
int spacedim>
311 const Vector<Number> & criteria,
312 const unsigned int order = 2);
328 template <
int dim,
typename Number,
int spacedim>
331 const Vector<Number> & criteria,
332 const double threshold,
349 template <
int dim,
typename Number,
int spacedim>
352 const Vector<Number> & criteria,
353 const double threshold);
372 #endif // dealii_grid_refinement_h
void refine_and_coarsen_fixed_number(Triangulation< dim, spacedim > &triangulation, const Vector< Number > &criteria, const double top_fraction_of_cells, const double bottom_fraction_of_cells, const unsigned int max_n_cells=std::numeric_limits< unsigned int >::max())
std::pair< double, double > adjust_refine_and_coarsen_number_fraction(const types::global_cell_index current_n_cells, const types::global_cell_index max_n_cells, const double top_fraction_of_cells, const double bottom_fraction_of_cells)
void coarsen(Triangulation< dim, spacedim > &tria, const Vector< Number > &criteria, const double threshold)
void refine_and_coarsen_fixed_fraction(Triangulation< dim, spacedim > &tria, const Vector< Number > &criteria, const double top_fraction, const double bottom_fraction, const unsigned int max_n_cells=std::numeric_limits< unsigned int >::max())
static ::ExceptionBase & ExcNegativeCriteria()
#define DEAL_II_NAMESPACE_OPEN
void refine_and_coarsen_optimize(Triangulation< dim, spacedim > &tria, const Vector< Number > &criteria, const unsigned int order=2)
#define DeclException0(Exception0)
static const unsigned int invalid_unsigned_int
const typename ::parallel::distributed::Triangulation< dim, spacedim > * triangulation
static ::ExceptionBase & ExcInvalidParameterValue()
#define DEAL_II_NAMESPACE_CLOSE
void refine(Triangulation< dim, spacedim > &tria, const Vector< Number > &criteria, const double threshold, const unsigned int max_to_mark=numbers::invalid_unsigned_int)
T max(const T &t, const MPI_Comm &mpi_communicator)