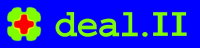 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_fe_tools_H
17 #define dealii_fe_tools_H
44 template <
typename number>
48 template <
int dim,
int spacedim>
50 template <
int dim,
int spacedim>
54 template <
typename number>
90 template <
int dim,
int spacedim = dim>
97 virtual std::unique_ptr<FiniteElement<dim, spacedim>>
98 get(
const unsigned int degree)
const = 0;
105 virtual std::unique_ptr<FiniteElement<dim, spacedim>>
132 virtual std::unique_ptr<FiniteElement<FE::dimension, FE::space_dimension>>
133 get(
const unsigned int degree)
const override;
139 virtual std::unique_ptr<FiniteElement<FE::dimension, FE::space_dimension>>
158 template <
int dim,
int spacedim>
161 std::vector<unsigned int> & renumbering,
162 std::vector<std::vector<unsigned int>> &start_indices);
179 template <
int dim,
int spacedim>
182 std::vector<types::global_dof_index> &renumbering,
183 std::vector<types::global_dof_index> &block_data,
184 bool return_start_indices =
true);
199 template <
int dim,
typename number,
int spacedim>
216 template <
int dim,
typename number,
int spacedim>
233 template <
int dim,
typename number,
int spacedim>
242 template <
int dim,
typename number,
int spacedim>
316 template <
int dim,
int spacedim>
358 template <
int dim,
typename number,
int spacedim>
363 const bool isotropic_only =
false,
364 const double threshold = 1.
e-12);
387 template <
int dim,
typename number,
int spacedim>
392 const unsigned int face_coarse,
393 const unsigned int face_fine,
394 const double threshold = 1.
e-12);
427 template <
int dim,
typename number,
int spacedim>
432 const bool isotropic_only =
false);
519 template <
int dim,
int spacedim>
534 template <
int dim,
int spacedim>
584 template <
int dim,
int spacedim>
591 const unsigned int face,
611 template <
int dim,
int spacedim,
typename number>
616 std::vector<number> & dof_values);
656 template <
int,
int>
class DoFHandlerType1,
657 template <
int,
int>
class DoFHandlerType2,
661 interpolate(
const DoFHandlerType1<dim, spacedim> &dof1,
663 const DoFHandlerType2<dim, spacedim> &dof2,
684 template <
int,
int>
class DoFHandlerType1,
685 template <
int,
int>
class DoFHandlerType2,
690 const DoFHandlerType1<dim, spacedim> & dof1,
692 const DoFHandlerType2<dim, spacedim> & dof2,
712 template <
int,
int>
class DoFHandlerType,
720 OutVector & u1_interpolated);
734 template <
int dim,
class InVector,
class OutVector,
int spacedim>
742 OutVector & u1_interpolated);
753 template <
int dim,
class InVector,
class OutVector,
int spacedim>
758 OutVector & z1_difference);
772 template <
int dim,
class InVector,
class OutVector,
int spacedim>
780 OutVector & z1_difference);
792 template <
int dim,
class InVector,
class OutVector,
int spacedim>
859 template <
int dim,
class InVector,
class OutVector,
int spacedim>
878 template <
int dim,
class InVector,
class OutVector,
int spacedim>
903 std::vector<unsigned int>
916 std::vector<unsigned int> &h2l);
928 std::vector<unsigned int> & h2l);
945 std::vector<unsigned int>
955 std::vector<unsigned int> & l2h);
1039 namespace Compositing
1057 template <
int dim,
int spacedim>
1061 const std::vector<unsigned int> & multiplicities,
1062 const bool do_tensor_product =
true);
1069 template <
int dim,
int spacedim>
1072 const std::initializer_list<
1079 template <
int dim,
int spacedim>
1082 const unsigned int N1,
1084 const unsigned int N2 = 0,
1086 const unsigned int N3 = 0,
1088 const unsigned int N4 = 0,
1090 const unsigned int N5 = 0);
1104 template <
int dim,
int spacedim>
1108 const std::vector<unsigned int> & multiplicities);
1115 template <
int dim,
int spacedim>
1118 const std::initializer_list<
1136 template <
int dim,
int spacedim>
1140 const unsigned int N1,
1142 const unsigned int N2 = 0,
1144 const unsigned int N3 = 0,
1146 const unsigned int N4 = 0,
1148 const unsigned int N5 = 0);
1167 template <
int dim,
int spacedim>
1168 std::vector<ComponentMask>
1171 const std::vector<unsigned int> & multiplicities,
1172 const bool do_tensor_product =
true);
1179 template <
int dim,
int spacedim>
1180 std::vector<ComponentMask>
1182 const std::initializer_list<
1204 template <
int dim,
int spacedim>
1205 std::vector<ComponentMask>
1208 const unsigned int N1,
1210 const unsigned int N2 = 0,
1212 const unsigned int N3 = 0,
1214 const unsigned int N4 = 0,
1216 const unsigned int N5 = 0,
1217 const bool do_tensor_product =
true);
1235 template <
int dim,
int spacedim>
1238 std::vector<std::pair<std::pair<unsigned int, unsigned int>,
1239 unsigned int>> &system_to_base_table,
1240 std::vector<std::pair<unsigned int, unsigned int>>
1241 & system_to_component_table,
1242 std::vector<std::pair<std::pair<unsigned int, unsigned int>,
1243 unsigned int>> &component_to_base_table,
1245 const bool do_tensor_product =
true);
1262 template <
int dim,
int spacedim>
1265 std::vector<std::pair<std::pair<unsigned int, unsigned int>,
1266 unsigned int>> &face_system_to_base_table,
1267 std::vector<std::pair<unsigned int, unsigned int>>
1268 & face_system_to_component_table,
1270 const bool do_tensor_product =
true);
1308 template <
int dim,
int spacedim = dim>
1309 std::unique_ptr<FiniteElement<dim, spacedim>>
1363 template <
int dim,
int spacedim>
1379 <<
"Can't re-generate a finite element from the string '"
1396 <<
"The dimension " << arg1
1397 <<
" in the finite element string must match "
1398 <<
"the space dimension " << arg2 <<
".");
1428 "You are using continuous elements on a grid with "
1429 "hanging nodes but without providing hanging node "
1430 "constraints. Use the respective function with "
1431 "additional AffineConstraints argument(s), instead.");
1448 <<
"This is a " << arg1 <<
"x" << arg2 <<
" matrix, "
1449 <<
"but should be a " << arg3 <<
"x" << arg4 <<
" matrix.");
1458 <<
"Least squares fit leaves a gap of " << arg1);
1468 << arg1 <<
" must be greater than " << arg2);
1477 std::unique_ptr<FiniteElement<FE::dimension, FE::space_dimension>>
1480 return std_cxx14::make_unique<FE>(degree);
1483 namespace Compositing
1485 template <
int dim,
int spacedim>
1488 const std::initializer_list<
1492 std::vector<const FiniteElement<dim, spacedim> *> fes;
1493 std::vector<unsigned int> multiplicities;
1496 [&fes, &multiplicities](
1497 const std::pair<std::unique_ptr<FiniteElement<dim, spacedim>>,
1498 unsigned int> &fe_system) {
1499 fes.push_back(fe_system.first.get());
1500 multiplicities.push_back(fe_system.second);
1503 for (
const auto &p : fe_systems)
1511 template <
int dim,
int spacedim>
1514 const std::initializer_list<
1518 std::vector<const FiniteElement<dim, spacedim> *> fes;
1519 std::vector<unsigned int> multiplicities;
1522 [&fes, &multiplicities](
1523 const std::pair<std::unique_ptr<FiniteElement<dim, spacedim>>,
1524 unsigned int> &fe_system) {
1525 fes.push_back(fe_system.first.get());
1526 multiplicities.push_back(fe_system.second);
1529 for (
const auto &p : fe_systems)
1537 template <
int dim,
int spacedim>
1538 std::vector<ComponentMask>
1540 const std::initializer_list<
1544 std::vector<const FiniteElement<dim, spacedim> *> fes;
1545 std::vector<unsigned int> multiplicities;
1548 [&fes, &multiplicities](
1549 const std::pair<std::unique_ptr<FiniteElement<dim, spacedim>>,
1550 unsigned int> &fe_system) {
1551 fes.push_back(fe_system.first.get());
1552 multiplicities.push_back(fe_system.second);
1555 for (
const auto &p : fe_systems)
#define DeclExceptionMsg(Exception, defaulttext)
typename ActiveSelector::active_cell_iterator active_cell_iterator
static ::ExceptionBase & ExcInvalidFEName(std::string arg1)
static ::ExceptionBase & ExcLeastSquaresError(double arg1)
SymmetricTensor< 2, dim, Number > e(const Tensor< 2, dim, Number > &F)
static ::ExceptionBase & ExcNotGreaterThan(int arg1, int arg2)
constexpr ReturnType< rank, T >::value_type & extract(T &t, const ArrayType &indices)
static ::ExceptionBase & ExcHangingNodesNotAllowed()
static ::ExceptionBase & ExcTriangulationMismatch()
#define DEAL_II_NAMESPACE_OPEN
@ matrix
Contents is actually a matrix.
#define DEAL_II_DEPRECATED
#define DeclException1(Exception1, type1, outsequence)
static ::ExceptionBase & ExcInvalidFEDimension(char arg1, int arg2)
static ::ExceptionBase & ExcMatrixDimensionMismatch(int arg1, int arg2, int arg3, int arg4)
static ::ExceptionBase & ExcFENotPrimitive()
#define DeclException4(Exception4, type1, type2, type3, type4, outsequence)
#define DeclException0(Exception0)
static ::ExceptionBase & ExcInvalidFE()
#define DEAL_II_NAMESPACE_CLOSE
#define DeclException2(Exception2, type1, type2, outsequence)
static ::ExceptionBase & ExcGridNotRefinedAtLeastOnce()