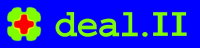 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_tensor_accessors_h
17 #define dealii_tensor_accessors_h
77 template <
int index,
int rank,
typename T>
79 template <
int position,
int rank>
81 template <
int no_contr,
int rank_1,
int rank_2,
int dim>
83 template <
int rank_1,
int rank_2,
int dim>
104 template <
typename T>
110 template <
typename T>
116 template <
typename T, std::
size_t N>
122 template <
typename T, std::
size_t N>
136 template <
int deref_steps,
typename T>
144 template <
typename T>
190 template <
int index,
int rank,
typename T>
194 static_assert(0 <= index && index < rank,
195 "The specified index must lie within the range [0,rank)");
224 template <
int rank,
typename T,
typename ArrayType>
273 template <
int no_contr,
281 contract(T1 &result,
const T2 &left,
const T3 &right)
283 static_assert(rank_1 >= no_contr,
284 "The rank of the left tensor must be "
285 "equal or greater than the number of "
287 static_assert(rank_2 >= no_contr,
288 "The rank of the right tensor must be "
289 "equal or greater than the number of "
293 template contract<T1, T2, T3>(result, left, right);
327 template <
int rank_1,
335 contract3(
const T2 &left,
const T3 &middle,
const T4 &right)
338 template contract3<T1, T2, T3, T4>(left, middle, right);
348 template <
int rank,
typename S>
350 template <
typename T>
352 template <
int no_contr,
int dim>
364 template <
typename T>
370 template <
int rank,
typename S>
376 template <
int index,
int rank,
typename T>
411 template <
int index,
int rank,
typename T>
445 template <
int rank,
typename T>
468 template <
typename T>
494 template <
typename T>
522 template <
int rank,
typename S>
545 return s_.apply(j)[
i_];
557 template <
typename S>
573 return s_.apply(j)[
i_];
589 template <
int position,
int rank>
592 template <
typename T,
typename ArrayType>
598 ArrayType>(t[indices[position]], indices);
607 template <
typename T,
typename ArrayType>
631 template <
int no_contr,
int rank_1,
int rank_2,
int dim>
635 template <
typename T1,
typename T2,
typename T3>
637 contract(T1 &result,
const T2 &left,
const T3 &right)
639 for (
unsigned int i = 0; i < dim; ++i)
661 template <
int no_contr,
int rank_2,
int dim>
665 template <
typename T1,
typename T2,
typename T3>
667 contract(T1 &result,
const T2 &left,
const T3 &right)
669 for (
unsigned int i = 0; i < dim; ++i)
691 template <
int no_contr,
int dim>
695 template <
typename T1,
typename T2,
typename T3>
697 contract(T1 &result,
const T2 &left,
const T3 &right)
707 template <
int no_contr,
int dim>
711 template <
typename T1,
typename T2,
typename T3>
725 Contract2<no_contr - 1, dim>::template contract2<T1>(left[0],
727 for (
unsigned int i = 1; i < dim; ++i)
743 template <
typename T1,
typename T2,
typename T3>
766 template <
int rank_1,
int rank_2,
int dim>
770 template <
typename T1,
typename T2,
typename T3,
typename T4>
772 contract3(
const T2 &left,
const T3 &middle,
const T4 &right)
777 for (
unsigned int i = 0; i < dim; ++i)
779 left[i], middle[i], right);
796 template <
int rank_2,
int dim>
800 template <
typename T1,
typename T2,
typename T3,
typename T4>
802 contract3(
const T2 &left,
const T3 &middle,
const T4 &right)
807 for (
unsigned int i = 0; i < dim; ++i)
823 template <
typename T1,
typename T2,
typename T3,
typename T4>
825 contract3(
const T2 &left,
const T3 &middle,
const T4 &right)
827 return left * middle * right;
constexpr ReferenceType< return_type >::type apply(unsigned int j) const
constexpr void contract(T1 &result, const T2 &left, const T3 &right)
const typename T::value_type value_type
ReferenceType< T >::type t_
constexpr StoreIndex(S s, int i)
typename T::value_type value_type
ReferenceType< T >::type t_
StoreIndex< rank - 1, StoreIndex< rank, S > > value_type
constexpr value_type operator[](unsigned int j) const
static constexpr const T & value(const T &t)
constexpr value_type operator[](unsigned int j) const
constexpr static void contract(T1 &result, const T2 &left, const T3 &right)
#define DEAL_II_ALWAYS_INLINE
#define DEAL_II_CONSTEXPR
typename ValueType< typename S::return_type >::value_type return_type
constexpr return_type & operator[](unsigned int j) const
constexpr ReturnType< rank, T >::value_type & extract(T &t, const ArrayType &indices)
typename ReturnType< deref_steps - 1, typename ValueType< T >::value_type >::value_type value_type
typename ValueType< T >::value_type return_type
constexpr static T1 contract2(const T2 &left, const T3 &right)
ReorderedIndexView< index - 1, rank - 1, typename ValueType< T >::value_type > value_type
constexpr value_type operator[](unsigned int j) const
constexpr static void contract(T1 &result, const T2 &left, const T3 &right)
ReferenceType< T >::type t_
#define DEAL_II_NAMESPACE_OPEN
constexpr StoreIndex(S s, int i)
constexpr ReferenceType< return_type >::type apply(unsigned int j) const
constexpr ReorderedIndexView(typename ReferenceType< T >::type t)
constexpr static T1 contract3(const T2 &left, const T3 &middle, const T4 &right)
constexpr static T1 contract2(const T2 &left, const T3 &right)
ReferenceType< T >::type t_
typename ValueType< typename S::return_type >::value_type return_type
constexpr ReorderedIndexView(typename ReferenceType< T >::type t)
constexpr internal::ReorderedIndexView< index, rank, T > reordered_index_view(T &t)
constexpr Identity(typename ReferenceType< T >::type t)
constexpr ReorderedIndexView(typename ReferenceType< T >::type t)
#define DEAL_II_NAMESPACE_CLOSE
constexpr T1 contract3(const T2 &left, const T3 &middle, const T4 &right)
typename ReferenceType< typename ValueType< T >::value_type >::type value_type
constexpr value_type operator[](unsigned int j) const
constexpr static T1 contract3(const T2 &left, const T3 &middle, const T4 &right)
constexpr static void contract(T1 &result, const T2 &left, const T3 &right)
constexpr static T1 contract3(const T2 &left, const T3 &middle, const T4 &right)