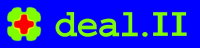 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_error_estimator_h
17 #define dealii_error_estimator_h
261 template <
int dim,
int spacedim = dim>
340 template <
typename InputVector,
typename DoFHandlerType>
344 const DoFHandlerType & dof,
349 const InputVector & solution,
350 Vector<float> & error,
362 template <
typename InputVector,
typename DoFHandlerType>
365 const DoFHandlerType & dof,
370 const InputVector & solution,
371 Vector<float> & error,
392 template <
typename InputVector,
typename DoFHandlerType>
396 const DoFHandlerType & dof,
401 const std::vector<const InputVector *> &solutions,
402 std::vector<Vector<float> *> & errors,
414 template <
typename InputVector,
typename DoFHandlerType>
417 const DoFHandlerType & dof,
422 const std::vector<const InputVector *> &solutions,
423 std::vector<Vector<float> *> & errors,
436 template <
typename InputVector,
typename DoFHandlerType>
440 const DoFHandlerType & dof,
445 const InputVector & solution,
446 Vector<float> & error,
459 template <
typename InputVector,
typename DoFHandlerType>
462 const DoFHandlerType & dof,
467 const InputVector & solution,
468 Vector<float> & error,
481 template <
typename InputVector,
typename DoFHandlerType>
485 const DoFHandlerType & dof,
490 const std::vector<const InputVector *> &solutions,
491 std::vector<Vector<float> *> & errors,
504 template <
typename InputVector,
typename DoFHandlerType>
507 const DoFHandlerType & dof,
512 const std::vector<const InputVector *> &solutions,
513 std::vector<Vector<float> *> & errors,
525 "You provided a ComponentMask argument that is invalid. "
526 "Component masks need to be either default constructed "
527 "(in which case they indicate that every component is "
528 "selected) or need to have a length equal to the number "
529 "of vector components of the finite element in use "
530 "by the DoFHandler object. In the latter case, at "
531 "least one component needs to be selected.");
537 "If you do specify the argument for a (possibly "
538 "spatially variable) coefficient function for this function, "
539 "then it needs to refer to a coefficient that is either "
540 "scalar (has one vector component) or has as many vector "
541 "components as there are in the finite element used by "
542 "the DoFHandler argument.");
550 <<
"You provided a function map that for boundary indicator "
551 << arg1 <<
" specifies a function with " << arg2
552 <<
" vector components. However, the finite "
553 "element in use has "
555 <<
" components, and these two numbers need to match.");
562 <<
"The number of input vectors, " << arg1
563 <<
" needs to be equal to the number of output vectors, "
565 <<
". This is not the case in your call of this function.");
570 "You need to specify at least one solution vector as "
587 template <
int spacedim>
628 template <
typename InputVector,
typename DoFHandlerType>
632 const DoFHandlerType & dof,
637 const InputVector & solution,
638 Vector<float> & error,
650 template <
typename InputVector,
typename DoFHandlerType>
653 const DoFHandlerType &dof,
658 const InputVector & solution,
659 Vector<float> & error,
680 template <
typename InputVector,
typename DoFHandlerType>
684 const DoFHandlerType & dof,
689 const std::vector<const InputVector *> &solutions,
690 std::vector<Vector<float> *> & errors,
702 template <
typename InputVector,
typename DoFHandlerType>
705 const DoFHandlerType &dof,
710 const std::vector<const InputVector *> &solutions,
711 std::vector<Vector<float> *> & errors,
724 template <
typename InputVector,
typename DoFHandlerType>
728 const DoFHandlerType & dof,
733 const InputVector & solution,
734 Vector<float> & error,
747 template <
typename InputVector,
typename DoFHandlerType>
750 const DoFHandlerType & dof,
755 const InputVector & solution,
756 Vector<float> & error,
769 template <
typename InputVector,
typename DoFHandlerType>
773 const DoFHandlerType & dof,
778 const std::vector<const InputVector *> &solutions,
779 std::vector<Vector<float> *> & errors,
792 template <
typename InputVector,
typename DoFHandlerType>
795 const DoFHandlerType & dof,
800 const std::vector<const InputVector *> &solutions,
801 std::vector<Vector<float> *> & errors,
813 "You provided a ComponentMask argument that is invalid. "
814 "Component masks need to be either default constructed "
815 "(in which case they indicate that every component is "
816 "selected) or need to have a length equal to the number "
817 "of vector components of the finite element in use "
818 "by the DoFHandler object. In the latter case, at "
819 "least one component needs to be selected.");
825 "If you do specify the argument for a (possibly "
826 "spatially variable) coefficient function for this function, "
827 "then it needs to refer to a coefficient that is either "
828 "scalar (has one vector component) or has as many vector "
829 "components as there are in the finite element used by "
830 "the DoFHandler argument.");
838 <<
"You provided a function map that for boundary indicator "
839 << arg1 <<
" specifies a function with " << arg2
840 <<
" vector components. However, the finite "
841 "element in use has "
843 <<
" components, and these two numbers need to match.");
850 <<
"The number of input vectors, " << arg1
851 <<
" needs to be equal to the number of output vectors, "
853 <<
". This is not the case in your call of this function.");
858 "You need to specify at least one solution vector as "
#define DeclExceptionMsg(Exception, defaulttext)
static ::ExceptionBase & ExcNoSolutions()
static ::ExceptionBase & ExcInvalidComponentMask()
@ cell_diameter_over_24
Kelly error estimator with the factor .
@ face_diameter_over_twice_max_degree
static ::ExceptionBase & ExcInvalidBoundaryFunction(types::boundary_id arg1, int arg2, int arg3)
@ cell_diameter
Kelly error estimator with the factor .
Abstract base class for mapping classes.
#define DEAL_II_NAMESPACE_OPEN
static void estimate(const Mapping< dim, spacedim > &mapping, const DoFHandlerType &dof, const Quadrature< dim - 1 > &quadrature, const std::map< types::boundary_id, const Function< spacedim, typename InputVector::value_type > * > &neumann_bc, const InputVector &solution, Vector< float > &error, const ComponentMask &component_mask=ComponentMask(), const Function< spacedim > *coefficients=nullptr, const unsigned int n_threads=numbers::invalid_unsigned_int, const types::subdomain_id subdomain_id=numbers::invalid_subdomain_id, const types::material_id material_id=numbers::invalid_material_id, const Strategy strategy=cell_diameter_over_24)
#define DeclException3(Exception3, type1, type2, type3, outsequence)
static ::ExceptionBase & ExcInvalidCoefficient()
unsigned int subdomain_id
const types::material_id invalid_material_id
static ::ExceptionBase & ExcIncompatibleNumberOfElements(int arg1, int arg2)
static const unsigned int invalid_unsigned_int
const types::subdomain_id invalid_subdomain_id
#define DEAL_II_NAMESPACE_CLOSE
@ face_diameter_over_twice_max_degree
#define DeclException2(Exception2, type1, type2, outsequence)