Loading [MathJax]/extensions/TeX/newcommand.js
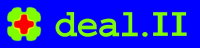 |
Reference documentation for deal.II version 9.2.0
|
\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}
\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}
\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}
\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}
Go to the documentation of this file.
16 #ifndef dealii_hp_dof_level_h
17 #define dealii_hp_dof_level_h
44 namespace DoFHandlerImplementation
46 struct Implementation;
49 namespace DoFCellAccessorImplementation
51 struct Implementation;
225 const unsigned int fe_index,
226 const unsigned int local_index,
242 const unsigned int fe_index,
243 const unsigned int local_index)
const;
257 const unsigned int fe_index)
const;
264 const unsigned int fe_index);
278 const unsigned int fe_index);
305 const unsigned int dofs_per_cell)
const;
318 template <
class Archive>
320 serialize(Archive &ar,
const unsigned int version);
331 template <
int dim,
int spacedim>
334 const ::hp::FECollection<dim, spacedim> &fe_collection);
344 template <
int dim,
int spacedim>
347 const ::hp::FECollection<dim, spacedim> &fe_collection);
374 friend class ::hp::DoFHandler;
375 friend struct ::internal::hp::DoFHandlerImplementation::
377 friend struct ::internal::DoFCellAccessorImplementation::
407 const unsigned int fe_index,
408 const unsigned int local_index)
const
416 ExcMessage(
"You are trying to access degree of freedom "
417 "information for an object on which no such "
418 "information is available"));
424 ExcMessage(
"FE index does not match that of the present cell"));
438 const unsigned int fe_index,
439 const unsigned int local_index,
449 ExcMessage(
"You are trying to access degree of freedom "
450 "information for an object on which no such "
451 "information is available"));
455 "This function can no longer be called after compressing the dof_indices array"));
457 ExcMessage(
"FE index does not match that of the present cell"));
478 const unsigned int fe_index)
const
487 const unsigned int fe_index)
498 "You are using an active_fe_index that is larger than an "
499 "internal limitation for these objects. Try to work with "
500 "hp::FECollection objects that have a more modest size."));
503 "You are using an active_fe_index that is reserved for "
504 "internal purposes for these objects. Try to work with "
505 "hp::FECollection objects that have a more modest size."));
527 const unsigned int fe_index)
538 "You are using a future_fe_index that is larger than an "
539 "internal limitation for these objects. Try to work with "
540 "hp::FECollection objects that have a more modest size."));
543 "You are using a future_fe_index that is reserved for "
544 "internal purposes for these objects. Try to work with "
545 "hp::FECollection objects that have a more modest size."));
574 const unsigned int dofs_per_cell)
const
582 "You are trying to access an element of the cache that stores "
583 "the indices of all degrees of freedom that live on one cell. "
584 "However, this element does not exist. Did you forget to call "
585 "DoFHandler::distribute_dofs(), or did you forget to call it "
586 "again after changing the active_fe_index of one of the cells?"));
593 template <
class Archive>
std::vector< types::global_dof_index > dof_indices
unsigned int future_fe_index(const unsigned int obj_index) const
std::vector< offset_type > dof_offsets
void set_dof_index(const unsigned int obj_index, const unsigned int fe_index, const unsigned int local_index, const types::global_dof_index global_index)
void normalize_active_fe_indices()
signed short int signed_active_fe_index_type
types::global_dof_index get_dof_index(const unsigned int obj_index, const unsigned int fe_index, const unsigned int local_index) const
bool fe_index_is_active(const unsigned int obj_index, const unsigned int fe_index) const
std::vector< offset_type > cell_cache_offsets
void set_future_fe_index(const unsigned int obj_index, const unsigned int fe_index)
#define AssertIndexRange(index, range)
static const active_fe_index_type invalid_active_fe_index
static bool is_compressed_entry(const active_fe_index_type active_fe_index)
const types::global_dof_index * get_cell_cache_start(const unsigned int obj_index, const unsigned int dofs_per_cell) const
static ::ExceptionBase & ExcMessage(std::string arg1)
unsigned int active_fe_index(const unsigned int obj_index) const
#define DEAL_II_NAMESPACE_OPEN
void serialize(Archive &ar, const unsigned int version)
std::vector< active_fe_index_type > future_fe_indices
static active_fe_index_type get_toggled_compression_state(const active_fe_index_type active_fe_index)
void set_active_fe_index(const unsigned int obj_index, const unsigned int fe_index)
std::size_t memory_consumption() const
std::vector< active_fe_index_type > active_fe_indices
std::vector< types::global_dof_index > cell_dof_indices_cache
bool future_fe_index_set(const unsigned int obj_index) const
#define Assert(cond, exc)
void uncompress_data(const ::hp::FECollection< dim, spacedim > &fe_collection)
unsigned short int active_fe_index_type
void clear_future_fe_index(const unsigned int obj_index)
#define DEAL_II_NAMESPACE_CLOSE
TrilinosWrappers::types::int_type global_index(const Epetra_BlockMap &map, const ::types::global_dof_index i)
void compress_data(const ::hp::FECollection< dim, spacedim > &fe_collection)