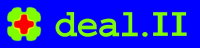 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_base_bounding_box_h
17 #define dealii_base_bounding_box_h
27 #include <boost/geometry/algorithms/envelope.hpp>
28 #include <boost/geometry/geometries/multi_point.hpp>
127 template <
int spacedim,
typename Number =
double>
152 template <
class Container>
201 extend(
const Number &amount);
237 bounds(
const unsigned int direction)
const;
244 vertex(
const unsigned int index)
const;
251 child(
const unsigned int index)
const;
266 template <
class Archive>
268 serialize(Archive &ar,
const unsigned int version);
279 template <
typename Number>
296 template <
class Container>
306 template <
int dim,
typename Number =
double>
348 const unsigned int coordiante_in_dim)
352 return (locked_coordinate + coordiante_in_dim + 1) % (dim + 1);
362 template <
int spacedim,
typename Number>
368 for (
unsigned int i = 0; i < spacedim; ++i)
369 Assert(boundary_points.first[i] <= boundary_points.second[i],
370 ExcMessage(
"Bounding Box can't be created: the points' "
371 "order should be bottom left, top right!"));
373 this->boundary_points = boundary_points;
378 template <
int spacedim,
typename Number>
379 template <
class Container>
382 boost::geometry::envelope(
390 template <
int spacedim,
typename Number>
394 for (
unsigned int d = 0;
d < spacedim; ++
d)
396 boundary_points.first[
d] -= amount;
397 boundary_points.second[
d] += amount;
398 Assert(boundary_points.first[
d] <= boundary_points.second[
d],
399 ExcMessage(
"Bounding Box can't be shrunk this much: the points' "
400 "order should remain bottom left, top right."));
405 template <
int spacedim,
typename Number>
406 template <
class Archive>
416 template <
typename Number>
424 template <
typename Number>
433 template <
typename Number>
434 template <
class Container>
Number lower_bound(const unsigned int direction) const
BoundingBox< spacedim, Number > child(const unsigned int index) const
#define AssertIndexRange(index, range)
Point< spacedim, Number > center() const
SymmetricTensor< 2, dim, Number > d(const Tensor< 2, dim, Number > &F, const Tensor< 2, dim, Number > &dF_dt)
void extend(const Number &amount)
#define DEAL_II_ENABLE_EXTRA_DIAGNOSTICS
void merge_with(const BoundingBox< spacedim, Number > &other_bbox)
static ::ExceptionBase & ExcMessage(std::string arg1)
Number upper_bound(const unsigned int direction) const
#define DEAL_II_NAMESPACE_OPEN
BoundingBox< spacedim - 1, Number > cross_section(const unsigned int direction) const
BoundingBox< dim, Number > create_unit_bounding_box()
bool point_inside(const Point< spacedim, Number > &p) const
std::pair< Point< spacedim, Number >, Point< spacedim, Number > > boundary_points
#define Assert(cond, exc)
NeighborType get_neighbor_type(const BoundingBox< spacedim, Number > &other_bbox) const
static ::ExceptionBase & ExcImpossibleInDim(int arg1)
#define DEAL_II_NAMESPACE_CLOSE
Number side_length(const unsigned int direction) const
BoundingBox< 1, Number > bounds(const unsigned int direction) const
std::pair< Point< spacedim, Number >, Point< spacedim, Number > > & get_boundary_points()
#define AssertThrow(cond, exc)
Point< spacedim, Number > vertex(const unsigned int index) const
unsigned int coordinate_to_one_dim_higher(const unsigned int locked_coordinate, const unsigned int coordiante_in_dim)
#define DEAL_II_DISABLE_EXTRA_DIAGNOSTICS
void serialize(Archive &ar, const unsigned int version)