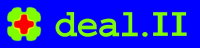 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_vector_space_vector_h
17 #define dealii_vector_space_vector_h
35 class CommunicationPatternBase;
36 template <
typename Number>
37 class ReadWriteVector;
56 template <
typename Number>
70 const bool omit_zeroing_entries =
false) = 0;
115 std::shared_ptr<const CommunicationPatternBase> communication_pattern =
116 std::shared_ptr<const CommunicationPatternBase>()) = 0;
127 add(
const Number a) = 0;
256 print(std::ostream & out,
257 const unsigned int precision = 3,
258 const bool scientific =
true,
259 const bool across =
true)
const = 0;
284 template <
typename Number>
virtual ::IndexSet locally_owned_elements() const =0
types::global_dof_index size_type
virtual void equ(const Number a, const VectorSpaceVector< Number > &V)=0
virtual VectorSpaceVector< Number > & operator+=(const VectorSpaceVector< Number > &V)=0
virtual ~VectorSpaceVector()=default
virtual real_type l2_norm() const =0
typename numbers::NumberTraits< float >::real_type real_type
virtual size_type size() const =0
virtual void compress(VectorOperation::values)
virtual VectorSpaceVector< Number > & operator-=(const VectorSpaceVector< Number > &V)=0
virtual Number operator*(const VectorSpaceVector< Number > &V) const =0
virtual real_type l1_norm() const =0
unsigned int global_dof_index
virtual VectorSpaceVector< Number > & operator/=(const Number factor)=0
virtual void scale(const VectorSpaceVector< Number > &scaling_factors)=0
virtual void reinit(const VectorSpaceVector< Number > &V, const bool omit_zeroing_entries=false)=0
virtual real_type linfty_norm() const =0
#define DEAL_II_NAMESPACE_OPEN
SymmetricTensor< 2, dim, Number > b(const Tensor< 2, dim, Number > &F)
void set_zero_mean_value(VectorSpaceVector< Number > &vector)
virtual Number add_and_dot(const Number a, const VectorSpaceVector< Number > &V, const VectorSpaceVector< Number > &W)=0
virtual bool all_zero() const =0
virtual std::size_t memory_consumption() const =0
virtual void print(std::ostream &out, const unsigned int precision=3, const bool scientific=true, const bool across=true) const =0
virtual void add(const Number a)=0
virtual VectorSpaceVector< Number > & operator*=(const Number factor)=0
#define DEAL_II_NAMESPACE_CLOSE
virtual void sadd(const Number s, const Number a, const VectorSpaceVector< Number > &V)=0
virtual VectorSpaceVector< Number > & operator=(const Number s)=0
virtual value_type mean_value() const =0