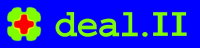 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_tridiagonal_matrix_h
17 #define dealii_tridiagonal_matrix_h
32 template <
typename number>
52 template <
typename number>
146 const bool adding =
false)
const;
169 const bool adding =
false)
const;
225 template <
class OutputStream>
227 print(OutputStream & s,
228 const unsigned int width = 5,
229 const unsigned int precision = 2)
const;
274 template <
typename number>
283 template <
typename number>
291 template <
typename number>
318 template <
typename number>
345 template <
typename number>
346 template <
class OutputStream>
349 const unsigned int width,
350 const unsigned int)
const
355 s << std::setw(width) << (*this)(i, i - 1);
357 s << std::setw(width) <<
"";
359 s <<
' ' << (*this)(i, i) <<
' ';
362 s << std::setw(width) << (*this)(i, i + 1);
@ diagonal
Matrix is diagonal.
void reinit(size_type n, bool symmetric=false)
std::vector< number > left
void Tvmult_add(Vector< number > &w, const Vector< number > &v) const
void compute_eigenvalues()
void print(OutputStream &s, const unsigned int width=5, const unsigned int precision=2) const
#define AssertIndexRange(index, range)
number matrix_scalar_product(const Vector< number > &u, const Vector< number > &v) const
Tensor< 2, dim, Number > w(const Tensor< 2, dim, Number > &F, const Tensor< 2, dim, Number > &dF_dt)
void vmult(Vector< number > &w, const Vector< number > &v, const bool adding=false) const
@ symmetric
Matrix is symmetric.
std::vector< number > right
static ::ExceptionBase & ExcIndexRange(int arg1, int arg2, int arg3)
number matrix_norm_square(const Vector< number > &v) const
unsigned int global_dof_index
#define DEAL_II_NAMESPACE_OPEN
number eigenvalue(const size_type i) const
LAPACKSupport::State state
TridiagonalMatrix(size_type n=0, bool symmetric=false)
static ::ExceptionBase & ExcInternalError()
types::global_dof_index size_type
number operator()(size_type i, size_type j) const
#define Assert(cond, exc)
void Tvmult(Vector< number > &w, const Vector< number > &v, const bool adding=false) const
std::vector< number > diagonal
void vmult_add(Vector< number > &w, const Vector< number > &v) const
#define DEAL_II_NAMESPACE_CLOSE