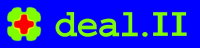 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_tensor_product_polynomials_h
17 #define dealii_tensor_product_polynomials_h
73 template <
int dim,
typename PolynomialType = Polynomials::Polynomial<
double>>
108 const std::vector<unsigned int> &
114 const std::vector<unsigned int> &
131 std::vector<double> & values,
204 name()
const override;
209 virtual std::unique_ptr<ScalarPolynomialsBase<dim>>
210 clone()
const override;
242 std::array<unsigned int, dim> &indices)
const;
324 std::vector<double> & values,
397 name()
const override;
402 virtual std::unique_ptr<ScalarPolynomialsBase<dim>>
403 clone()
const override;
409 const std::vector<std::vector<Polynomials::Polynomial<double>>>
polynomials;
419 std::array<unsigned int, dim> &indices)
const;
437 template <
int dim,
typename PolynomialType>
440 const std::vector<Pol> &pols)
442 , polynomials(pols.
begin(), pols.
end())
443 , index_map(this->n())
444 , index_map_inverse(this->n())
448 for (
unsigned int i = 0; i < this->
n(); ++i)
451 index_map_inverse[i] = i;
456 template <
int dim,
typename PolynomialType>
457 inline const std::vector<unsigned int> &
464 template <
int dim,
typename PolynomialType>
465 inline const std::vector<unsigned int> &
468 return index_map_inverse;
472 template <
int dim,
typename PolynomialType>
476 return "TensorProductPolynomials";
480 template <
int dim,
typename PolynomialType>
484 const unsigned int i,
487 std::array<unsigned int, dim> indices;
490 std::array<std::array<double, 5>, dim> v;
492 std::vector<double> tmp(5);
493 for (
unsigned int d = 0;
d < dim; ++
d)
495 polynomials[indices[
d]].value(p(
d), tmp);
511 for (
unsigned int d = 0;
d < dim; ++
d)
513 derivative_1[
d] = 1.;
514 for (
unsigned int x = 0; x < dim; ++x)
516 unsigned int x_order = 0;
520 derivative_1[
d] *= v[x][x_order];
530 for (
unsigned int d1 = 0; d1 < dim; ++d1)
531 for (
unsigned int d2 = 0; d2 < dim; ++d2)
533 derivative_2[d1][d2] = 1.;
534 for (
unsigned int x = 0; x < dim; ++x)
536 unsigned int x_order = 0;
542 derivative_2[d1][d2] *= v[x][x_order];
552 for (
unsigned int d1 = 0; d1 < dim; ++d1)
553 for (
unsigned int d2 = 0; d2 < dim; ++d2)
554 for (
unsigned int d3 = 0; d3 < dim; ++d3)
556 derivative_3[d1][d2][d3] = 1.;
557 for (
unsigned int x = 0; x < dim; ++x)
559 unsigned int x_order = 0;
567 derivative_3[d1][d2][d3] *= v[x][x_order];
577 for (
unsigned int d1 = 0; d1 < dim; ++d1)
578 for (
unsigned int d2 = 0; d2 < dim; ++d2)
579 for (
unsigned int d3 = 0; d3 < dim; ++d3)
580 for (
unsigned int d4 = 0; d4 < dim; ++d4)
582 derivative_4[d1][d2][d3][d4] = 1.;
583 for (
unsigned int x = 0; x < dim; ++x)
585 unsigned int x_order = 0;
595 derivative_4[d1][d2][d3][d4] *= v[x][x_order];
615 std::array<unsigned int, dim> indices;
618 std::vector<std::vector<double>> v(dim, std::vector<double>(order + 1));
619 for (
unsigned int d = 0;
d < dim; ++
d)
620 polynomials[
d][indices[
d]].
value(p(
d), v[
d]);
629 for (
unsigned int d = 0;
d < dim; ++
d)
631 derivative_1[
d] = 1.;
632 for (
unsigned int x = 0; x < dim; ++x)
634 unsigned int x_order = 0;
638 derivative_1[
d] *= v[x][x_order];
648 for (
unsigned int d1 = 0; d1 < dim; ++d1)
649 for (
unsigned int d2 = 0; d2 < dim; ++d2)
651 derivative_2[d1][d2] = 1.;
652 for (
unsigned int x = 0; x < dim; ++x)
654 unsigned int x_order = 0;
660 derivative_2[d1][d2] *= v[x][x_order];
670 for (
unsigned int d1 = 0; d1 < dim; ++d1)
671 for (
unsigned int d2 = 0; d2 < dim; ++d2)
672 for (
unsigned int d3 = 0; d3 < dim; ++d3)
674 derivative_3[d1][d2][d3] = 1.;
675 for (
unsigned int x = 0; x < dim; ++x)
677 unsigned int x_order = 0;
685 derivative_3[d1][d2][d3] *= v[x][x_order];
695 for (
unsigned int d1 = 0; d1 < dim; ++d1)
696 for (
unsigned int d2 = 0; d2 < dim; ++d2)
697 for (
unsigned int d3 = 0; d3 < dim; ++d3)
698 for (
unsigned int d4 = 0; d4 < dim; ++d4)
700 derivative_4[d1][d2][d3][d4] = 1.;
701 for (
unsigned int x = 0; x < dim; ++x)
703 unsigned int x_order = 0;
713 derivative_4[d1][d2][d3][d4] *= v[x][x_order];
732 return "AnisotropicPolynomials";
std::string name() const override
static ::ExceptionBase & ExcNotImplemented()
std::string name() const override
Tensor< 1, dim > compute_grad(const unsigned int i, const Point< dim > &p) const
virtual std::unique_ptr< ScalarPolynomialsBase< dim > > clone() const override
Tensor< 2, dim > compute_grad_grad(const unsigned int i, const Point< dim > &p) const
virtual std::size_t memory_consumption() const override
SymmetricTensor< 2, dim, Number > d(const Tensor< 2, dim, Number > &F, const Tensor< 2, dim, Number > &dF_dt)
Tensor< order, dim > compute_derivative(const unsigned int i, const Point< dim > &p) const
Tensor< 1, dim > compute_grad(const unsigned int i, const Point< dim > &p) const
std::vector< PolynomialType > polynomials
void output_indices(std::ostream &out) const
const std::vector< std::vector< Polynomials::Polynomial< double > > > polynomials
VectorType::value_type * begin(VectorType &V)
#define DEAL_II_NAMESPACE_OPEN
void compute_index(const unsigned int i, std::array< unsigned int, dim > &indices) const
double compute_value(const unsigned int i, const Point< dim > &p) const
const std::vector< unsigned int > & get_numbering_inverse() const
void evaluate(const Point< dim > &unit_point, std::vector< double > &values, std::vector< Tensor< 1, dim >> &grads, std::vector< Tensor< 2, dim >> &grad_grads, std::vector< Tensor< 3, dim >> &third_derivatives, std::vector< Tensor< 4, dim >> &fourth_derivatives) const override
VectorType::value_type * end(VectorType &V)
unsigned int compute_index()
AnisotropicPolynomials(const std::vector< std::vector< Polynomials::Polynomial< double >>> &base_polynomials)
void evaluate(const Point< dim > &unit_point, std::vector< double > &values, std::vector< Tensor< 1, dim >> &grads, std::vector< Tensor< 2, dim >> &grad_grads, std::vector< Tensor< 3, dim >> &third_derivatives, std::vector< Tensor< 4, dim >> &fourth_derivatives) const override
virtual std::unique_ptr< ScalarPolynomialsBase< dim > > clone() const override
#define Assert(cond, exc)
const std::vector< unsigned int > & get_numbering() const
TensorProductPolynomials(const std::vector< Pol > &pols)
std::vector< unsigned int > index_map
std::vector< unsigned int > index_map_inverse
void compute_index(const unsigned int i, std::array< unsigned int, dim > &indices) const
static const unsigned int dimension
Tensor< order, dim > compute_derivative(const unsigned int i, const Point< dim > &p) const
#define DEAL_II_NAMESPACE_CLOSE
static unsigned int get_n_tensor_pols(const std::vector< std::vector< Polynomials::Polynomial< double >>> &pols)
double compute_value(const unsigned int i, const Point< dim > &p) const
Tensor< 2, dim > compute_grad_grad(const unsigned int i, const Point< dim > &p) const
void set_numbering(const std::vector< unsigned int > &renumber)