Loading [MathJax]/extensions/TeX/AMSsymbols.js
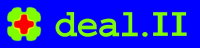 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_table_indices_h
17 #define dealii_table_indices_h
49 "TableIndices objects need to represent at least one index.");
64 template <
typename...
T>
100 template <
class Archive>
102 serialize(Archive &ar,
const unsigned int version);
116 template <
typename...
T>
118 : indices{
static_cast<const unsigned int>(args)...}
122 "Not all of the parameters have integral type!");
123 static_assert(
sizeof...(
T) ==
N,
"Wrong number of constructor arguments!");
159 return !(*
this == other);
172 template <
class Archive>
191 for (
unsigned int i = 0; i <
N; ++i)
#define AssertIndexRange(index, range)
void serialize(Archive &ar, const unsigned int version)
constexpr TableIndices()=default
#define DEAL_II_CONSTEXPR
VectorType::value_type * begin(VectorType &V)
#define DEAL_II_NAMESPACE_OPEN
VectorType::value_type * end(VectorType &V)
constexpr bool operator!=(const TableIndices< N > &other) const
OutputOperator< VectorType > & operator<<(OutputOperator< VectorType > &out, unsigned int step)
constexpr bool operator==(const TableIndices< N > &other) const
constexpr std::size_t operator[](const unsigned int i) const
#define DEAL_II_NAMESPACE_CLOSE