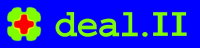 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_differentiation_sd_symengine_math_h
17 #define dealii_differentiation_sd_symengine_math_h
21 #ifdef DEAL_II_WITH_SYMENGINE
25 # include <type_traits>
70 template <
typename NumberType,
71 typename =
typename std::enable_if<
89 template <
typename NumberType,
90 typename =
typename std::enable_if<
106 sqrt(
const Expression &x);
115 cbrt(
const Expression &x);
125 exp(
const Expression &exponent);
134 log(
const Expression &x);
144 log(
const Expression &x,
const Expression &base);
155 template <
typename NumberType,
156 typename =
typename std::enable_if<
174 template <
typename NumberType,
175 typename =
typename std::enable_if<
191 log10(
const Expression &x);
208 sin(
const Expression &x);
218 cos(
const Expression &x);
228 tan(
const Expression &x);
238 csc(
const Expression &x);
248 sec(
const Expression &x);
258 cot(
const Expression &x);
269 asin(
const Expression &x);
280 acos(
const Expression &x);
291 atan(
const Expression &x);
302 atan2(
const Expression &y,
const Expression &x);
314 template <
typename NumberType,
315 typename =
typename std::enable_if<
334 template <
typename NumberType,
335 typename =
typename std::enable_if<
353 acsc(
const Expression &x);
364 asec(
const Expression &x);
375 acot(
const Expression &x);
393 sinh(
const Expression &x);
404 cosh(
const Expression &x);
415 tanh(
const Expression &x);
426 csch(
const Expression &x);
437 sech(
const Expression &x);
448 coth(
const Expression &x);
459 asinh(
const Expression &x);
470 acosh(
const Expression &x);
481 atanh(
const Expression &x);
492 acsch(
const Expression &x);
503 asech(
const Expression &x);
514 acoth(
const Expression &x);
530 abs(
const Expression &x);
540 fabs(
const Expression &x);
551 sign(
const Expression &x);
572 floor(
const Expression &x);
582 ceil(
const Expression &x);
592 max(
const Expression &a,
const Expression &
b);
603 template <
typename NumberType,
604 typename =
typename std::enable_if<
622 template <
typename NumberType,
623 typename =
typename std::enable_if<
640 min(
const Expression &a,
const Expression &
b);
651 template <
typename NumberType,
652 typename =
typename std::enable_if<
670 template <
typename NumberType,
671 typename =
typename std::enable_if<
688 erf(
const Expression &x);
698 erfc(
const Expression &x);
718 # define DEAL_II_EXPOSE_SYMENGINE_UNARY_MATH_FUNCTION(func) \
719 using ::Differentiation::SD::func;
721 # define DEAL_II_EXPOSE_SYMENGINE_BINARY_MATH_FUNCTION(func) \
722 using ::Differentiation::SD::func;
724 DEAL_II_EXPOSE_SYMENGINE_BINARY_MATH_FUNCTION(
pow)
725 DEAL_II_EXPOSE_SYMENGINE_BINARY_MATH_FUNCTION(
max)
726 DEAL_II_EXPOSE_SYMENGINE_BINARY_MATH_FUNCTION(
min)
727 DEAL_II_EXPOSE_SYMENGINE_BINARY_MATH_FUNCTION(
copysign)
729 DEAL_II_EXPOSE_SYMENGINE_UNARY_MATH_FUNCTION(
exp)
730 DEAL_II_EXPOSE_SYMENGINE_UNARY_MATH_FUNCTION(
log10)
731 DEAL_II_EXPOSE_SYMENGINE_UNARY_MATH_FUNCTION(
sqrt)
732 DEAL_II_EXPOSE_SYMENGINE_UNARY_MATH_FUNCTION(
cbrt)
733 DEAL_II_EXPOSE_SYMENGINE_UNARY_MATH_FUNCTION(
erf)
734 DEAL_II_EXPOSE_SYMENGINE_UNARY_MATH_FUNCTION(
erfc)
736 DEAL_II_EXPOSE_SYMENGINE_UNARY_MATH_FUNCTION(
log)
738 DEAL_II_EXPOSE_SYMENGINE_UNARY_MATH_FUNCTION(
abs)
739 DEAL_II_EXPOSE_SYMENGINE_UNARY_MATH_FUNCTION(
fabs)
740 DEAL_II_EXPOSE_SYMENGINE_UNARY_MATH_FUNCTION(
sign)
741 DEAL_II_EXPOSE_SYMENGINE_UNARY_MATH_FUNCTION(
floor)
742 DEAL_II_EXPOSE_SYMENGINE_UNARY_MATH_FUNCTION(
ceil)
744 DEAL_II_EXPOSE_SYMENGINE_UNARY_MATH_FUNCTION(
sin)
745 DEAL_II_EXPOSE_SYMENGINE_UNARY_MATH_FUNCTION(
cos)
746 DEAL_II_EXPOSE_SYMENGINE_UNARY_MATH_FUNCTION(
tan)
747 DEAL_II_EXPOSE_SYMENGINE_UNARY_MATH_FUNCTION(
csc)
748 DEAL_II_EXPOSE_SYMENGINE_UNARY_MATH_FUNCTION(
sec)
749 DEAL_II_EXPOSE_SYMENGINE_UNARY_MATH_FUNCTION(
cot)
751 DEAL_II_EXPOSE_SYMENGINE_UNARY_MATH_FUNCTION(
asin)
752 DEAL_II_EXPOSE_SYMENGINE_UNARY_MATH_FUNCTION(
acos)
753 DEAL_II_EXPOSE_SYMENGINE_UNARY_MATH_FUNCTION(
atan)
754 DEAL_II_EXPOSE_SYMENGINE_BINARY_MATH_FUNCTION(
atan2)
755 DEAL_II_EXPOSE_SYMENGINE_UNARY_MATH_FUNCTION(
acsc)
756 DEAL_II_EXPOSE_SYMENGINE_UNARY_MATH_FUNCTION(
asec)
757 DEAL_II_EXPOSE_SYMENGINE_UNARY_MATH_FUNCTION(
acot)
759 DEAL_II_EXPOSE_SYMENGINE_UNARY_MATH_FUNCTION(
sinh)
760 DEAL_II_EXPOSE_SYMENGINE_UNARY_MATH_FUNCTION(
cosh)
761 DEAL_II_EXPOSE_SYMENGINE_UNARY_MATH_FUNCTION(
tanh)
762 DEAL_II_EXPOSE_SYMENGINE_UNARY_MATH_FUNCTION(
csch)
763 DEAL_II_EXPOSE_SYMENGINE_UNARY_MATH_FUNCTION(
sech)
764 DEAL_II_EXPOSE_SYMENGINE_UNARY_MATH_FUNCTION(
coth)
766 DEAL_II_EXPOSE_SYMENGINE_UNARY_MATH_FUNCTION(
asinh)
767 DEAL_II_EXPOSE_SYMENGINE_UNARY_MATH_FUNCTION(
acosh)
768 DEAL_II_EXPOSE_SYMENGINE_UNARY_MATH_FUNCTION(
atanh)
769 DEAL_II_EXPOSE_SYMENGINE_UNARY_MATH_FUNCTION(
acsch)
770 DEAL_II_EXPOSE_SYMENGINE_UNARY_MATH_FUNCTION(
asech)
771 DEAL_II_EXPOSE_SYMENGINE_UNARY_MATH_FUNCTION(
acoth)
773 # undef DEAL_II_EXPOSE_SYMENGINE_BINARY_MATH_FUNCTION
774 # undef DEAL_II_EXPOSE_SYMENGINE_UNARY_MATH_FUNCTION
780 #endif // DEAL_II_WITH_SYMENGINE
Expression floor(const Expression &x)
Expression csch(const Expression &x)
Expression atan2(const Expression &y, const NumberType &x)
Expression copysign(const Expression &value, const Expression &sign)
Expression coth(const Expression &x)
Expression atanh(const Expression &x)
Expression atan(const Expression &x)
Expression erfc(const Expression &x)
Expression tanh(const Expression &x)
Expression erf(const Expression &x)
Expression acoth(const Expression &x)
Expression sech(const Expression &x)
Expression csc(const Expression &x)
Expression asinh(const Expression &x)
Expression pow(const NumberType &base, const Expression &exponent)
Expression sinh(const Expression &x)
Expression cosh(const Expression &x)
Expression fabs(const Expression &x)
Expression max(const Expression &a, const Expression &b)
Expression log(const NumberType &x, const Expression &base)
Expression atan2(const Expression &y, const Expression &x)
Expression min(const Expression &a, const Expression &b)
Expression asech(const Expression &x)
#define DEAL_II_NAMESPACE_OPEN
SymmetricTensor< 2, dim, Number > b(const Tensor< 2, dim, Number > &F)
Expression acot(const Expression &x)
Expression abs(const Expression &x)
Expression sin(const Expression &x)
Expression max(const NumberType &a, const Expression &b)
Expression cot(const Expression &x)
Expression ceil(const Expression &x)
Expression acosh(const Expression &x)
Expression sqrt(const Expression &x)
Expression pow(const Expression &base, const Expression &exponent)
Expression asec(const Expression &x)
Expression cbrt(const Expression &x)
Expression min(const NumberType &a, const Expression &b)
Expression log(const Expression &x)
Expression sign(const Expression &x)
Expression acsc(const Expression &x)
Expression acos(const Expression &x)
Expression cos(const Expression &x)
#define DEAL_II_NAMESPACE_CLOSE
Expression log10(const Expression &x)
Expression sec(const Expression &x)
Expression tan(const Expression &x)
Expression exp(const Expression &exponent)
Expression asin(const Expression &x)
Expression acsch(const Expression &x)