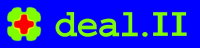 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
35 , object_info(subscriptor.object_info)
37 for (
const auto validity_ptr : subscriptor.validity_pointers)
38 *validity_ptr =
false;
46 *validity_ptr =
false;
76 # if __cpp_lib_uncaught_exceptions >= 201411
78 if (std::uncaught_exceptions() == 0)
80 if (std::uncaught_exception() ==
false)
83 std::string infostring;
86 if (map_entry.second > 0)
87 infostring += std::string(
"\n from Subscriber ") +
88 std::string(map_entry.first);
91 if (infostring.empty())
92 infostring =
"<none>";
102 <<
"---------------------------------------------------------"
104 <<
"An object pointed to by a SmartPointer is being destroyed."
106 <<
"Under normal circumstances, this would abort the program."
108 <<
"However, another exception is being processed at the"
110 <<
"moment, so the program will continue to run to allow"
112 <<
"this exception to be processed." << std::endl
113 <<
"---------------------------------------------------------"
125 for (
const auto validity_ptr : s.validity_pointers)
126 *validity_ptr =
false;
135 const std::string &
id)
const
137 std::lock_guard<std::mutex> lock(
mutex);
155 const std::string &
id)
const
166 std::lock_guard<std::mutex> lock(
mutex);
183 auto validity_ptr_it =
190 "This Subscriptor object does not know anything about the supplied pointer!"));
static ::ExceptionBase & ExcInUse(int arg1, std::string arg2, std::string arg3)
const std::type_info * object_info
void unsubscribe(std::atomic< bool > *const validity, const std::string &identifier="") const
decltype(counter_map)::iterator map_iterator
Subscriptor & operator=(const Subscriptor &)
static const char * unknown_subscriber
std::vector< std::atomic< bool > * > validity_pointers
void subscribe(std::atomic< bool > *const validity, const std::string &identifier="") const
void check_no_subscribers() const noexcept
void list_subscribers() const
static ::ExceptionBase & ExcMessage(std::string arg1)
#define DEAL_II_NAMESPACE_OPEN
std::map< std::string, unsigned int > counter_map
static ::ExceptionBase & ExcNoSubscriber(std::string arg1, std::string arg2)
#define AssertNothrow(cond, exc)
#define DEAL_II_NAMESPACE_CLOSE
std::atomic< unsigned int > counter