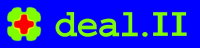 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_sparse_vanka_h
17 #define dealii_sparse_vanka_h
33 template <
typename number>
35 template <
typename number>
37 template <
typename number>
40 template <
typename number>
42 template <
typename number>
139 template <
typename number>
183 const bool conserve_memory =
false,
201 const bool conserve_memory =
false,
240 template <
typename number2>
248 template <
typename number2>
294 template <
typename number2>
298 const std::vector<bool> *
const dof_mask =
nullptr)
const;
381 template <
typename T>
520 template <
typename number>
552 const bool conserve_memory =
false,
558 template <
typename number2>
560 vmult(Vector<number2> &dst,
const Vector<number2> &src)
const;
599 template <
typename number>
607 template <
typename number>
615 template <
typename number>
616 template <
typename number2>
619 const Vector<number2> & )
const
void Tvmult(Vector< number2 > &dst, const Vector< number2 > &src) const
const unsigned int n_blocks
static unsigned int n_threads()
static ::ExceptionBase & ExcNotImplemented()
AdditionalData(const std::vector< bool > &selected, const bool conserve_memory=false, const unsigned int n_threads=MultithreadInfo::n_threads())
void initialize(const SparseMatrix< number > &M, const AdditionalData &additional_data)
void compute_dof_masks(const SparseMatrix< number > &M, const std::vector< bool > &selected, const BlockingStrategy blocking_strategy)
void compute_inverse(const size_type row, std::vector< size_type > &local_indices)
std::vector< SmartPointer< FullMatrix< float >, SparseVanka< number > > > inverses
const std::vector< bool > & selected
const std::vector< bool > * selected
std::vector< std::vector< bool > > dof_masks
void vmult(Vector< number2 > &dst, const Vector< number2 > &src) const
unsigned int global_dof_index
VectorType::value_type * begin(VectorType &V)
void vmult(Vector< number2 > &dst, const Vector< number2 > &src) const
std::size_t memory_consumption() const
SmartPointer< const SparseMatrix< number >, SparseVanka< number > > matrix
#define DEAL_II_NAMESPACE_OPEN
VectorType::value_type * end(VectorType &V)
static ::ExceptionBase & ExcNotInitialized()
friend class SparseBlockVanka
#define Assert(cond, exc)
const unsigned int n_threads
#define DEAL_II_NAMESPACE_CLOSE
void apply_preconditioner(Vector< number2 > &dst, const Vector< number2 > &src, const std::vector< bool > *const dof_mask=nullptr) const
#define AssertThrow(cond, exc)
std::size_t memory_consumption() const