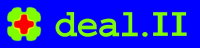 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_relaxation_block_h
17 #define dealii_relaxation_block_h
56 template <
typename MatrixType,
57 typename InverseNumberType =
typename MatrixType::value_type,
65 using number =
typename MatrixType::value_type;
175 std::vector<std::vector<unsigned int>>
order;
245 const bool backward)
const;
288 template <
typename MatrixType,
289 typename InverseNumberType =
typename MatrixType::value_type,
304 using number =
typename MatrixType::value_type;
386 template <
typename MatrixType,
387 typename InverseNumberType =
typename MatrixType::value_type,
402 using number =
typename MatrixType::value_type;
484 template <
typename MatrixType,
485 typename InverseNumberType =
typename MatrixType::value_type,
495 using number =
typename MatrixType::value_type;
void block_kernel(const size_type block_begin, const size_type block_end)
std::vector< std::vector< unsigned int > > order
typename MatrixType::value_type value_type
void Tstep(VectorType &dst, const VectorType &rhs) const
Householder< typename MatrixType::value_type > & inverse_householder(size_type i)
SparsityPattern block_list
SmartPointer< const AdditionalData, RelaxationBlock< MatrixType, InverseNumberType, VectorType > > additional_data
VectorType * temp_ghost_vector
SmartPointer< const MatrixType, RelaxationBlock< MatrixType, InverseNumberType, VectorType > > A
typename MatrixType::value_type number
void Tvmult(VectorType &dst, const VectorType &rhs) const
unsigned int global_dof_index
#define DEAL_II_NAMESPACE_OPEN
void vmult(VectorType &dst, const VectorType &rhs) const
void step(VectorType &dst, const VectorType &rhs) const
typename MatrixType::value_type number
typename MatrixType::value_type number
void vmult(VectorType &dst, const VectorType &rhs) const
void Tvmult(VectorType &dst, const VectorType &rhs) const
void step(VectorType &dst, const VectorType &rhs) const
void Tstep(VectorType &dst, const VectorType &rhs) const
void initialize(const MatrixType &A, const AdditionalData ¶meters)
PreconditionBlockBase< InverseNumberType >::Inversion inversion
void step(VectorType &dst, const VectorType &rhs) const
std::size_t memory_consumption() const
void Tvmult(VectorType &dst, const VectorType &rhs) const
typename MatrixType::value_type number
AdditionalData(const double relaxation=1., const bool invert_diagonal=true, const bool same_diagonal=false, const typename PreconditionBlockBase< InverseNumberType >::Inversion inversion=PreconditionBlockBase< InverseNumberType >::gauss_jordan, const double threshold=0., VectorType *temp_ghost_vector=nullptr)
#define DEAL_II_NAMESPACE_CLOSE
void Tstep(VectorType &dst, const VectorType &rhs) const
void vmult(VectorType &dst, const VectorType &rhs) const
void do_step(VectorType &dst, const VectorType &prev, const VectorType &src, const bool backward) const