Loading [MathJax]/extensions/TeX/newcommand.js
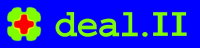 |
Reference documentation for deal.II version 9.2.0
|
\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}
\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}
\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}
\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}
Go to the documentation of this file.
16 #ifndef dealii_partitioner_h
17 #define dealii_partitioner_h
239 const IndexSet &read_write_vector_index_set,
289 std::pair<types::global_dof_index, types::global_dof_index>
353 const std::vector<std::pair<unsigned int, unsigned int>> &
361 const std::vector<std::pair<unsigned int, unsigned int>> &
372 const std::vector<std::pair<unsigned int, unsigned int>> &
391 const std::vector<std::pair<unsigned int, unsigned int>> &
454 #ifdef DEAL_II_WITH_MPI
490 template <
typename Number,
typename MemorySpaceType = MemorySpace::Host>
493 const unsigned int communication_channel,
497 std::vector<MPI_Request> & requests)
const;
516 template <
typename Number,
typename MemorySpaceType = MemorySpace::Host>
520 std::vector<MPI_Request> & requests)
const;
559 template <
typename Number,
typename MemorySpaceType = MemorySpace::Host>
563 const unsigned int communication_channel,
566 std::vector<MPI_Request> & requests)
const;
602 template <
typename Number,
typename MemorySpaceType = MemorySpace::Host>
609 std::vector<MPI_Request> & requests)
const;
624 <<
"Global index " << arg1
625 <<
" neither owned nor ghost on proc " << arg2 <<
".");
634 <<
"The size of the ghost index array (" << arg1
635 <<
") must either equal the number of ghost in the "
636 <<
"partitioner (" << arg2
637 <<
") or be equal in size to a more comprehensive index"
638 <<
"set which contains " << arg3
639 <<
" elements for this partitioner.");
663 std::pair<types::global_dof_index, types::global_dof_index>
702 std::pair<std::unique_ptr<
unsigned int[], void (*)(
unsigned int *)>,
741 std::vector<std::pair<unsigned int, unsigned int>>
787 inline std::pair<types::global_dof_index, types::global_dof_index>
802 return static_cast<unsigned int>(
size);
840 static_cast<unsigned int>(
879 inline const std::vector<std::pair<unsigned int, unsigned int>> &
887 inline const std::vector<std::pair<unsigned int, unsigned int>> &
894 inline const std::vector<std::pair<unsigned int, unsigned int>> &
910 inline const std::vector<std::pair<unsigned int, unsigned int>> &
954 #endif // ifndef DOXYGEN
unsigned int n_ghost_indices_in_larger_set
void set_ghost_indices(const IndexSet &ghost_indices, const IndexSet &larger_ghost_index_set=IndexSet())
unsigned int n_mpi_processes() const
bool is_ghost_entry(const types::global_dof_index global_index) const
static ::ExceptionBase & ExcNotImplemented()
std::vector< std::pair< unsigned int, unsigned int > > import_targets_data
#define AssertIndexRange(index, range)
types::global_dof_index global_size
types::global_dof_index size() const
static ::ExceptionBase & ExcIndexNotPresent(types::global_dof_index arg1, unsigned int arg2)
unsigned int n_import_indices_data
std::vector< std::pair< unsigned int, unsigned int > > import_indices_data
const std::vector< std::pair< unsigned int, unsigned int > > & import_indices() const
std::vector< std::pair< unsigned int, unsigned int > > ghost_targets_data
void export_to_ghosted_array_finish(const ArrayView< Number, MemorySpaceType > &ghost_array, std::vector< MPI_Request > &requests) const
const std::vector< std::pair< unsigned int, unsigned int > > & ghost_indices_within_larger_ghost_set() const
unsigned int n_ghost_indices_data
bool in_local_range(const types::global_dof_index global_index) const
void set_owned_indices(const IndexSet &locally_owned_indices)
unsigned int global_dof_index
std::vector< std::pair< unsigned int, unsigned int > > ghost_indices_subset_data
bool is_compatible(const Partitioner &part) const
std::size_t memory_consumption() const
unsigned int this_mpi_process() const
unsigned int n_ghost_indices() const
#define DEAL_II_NAMESPACE_OPEN
std::vector< unsigned int > ghost_indices_subset_chunks_by_rank_data
#define DeclException3(Exception3, type1, type2, type3, outsequence)
bool is_element(const size_type index) const
void import_from_ghosted_array_start(const VectorOperation::values vector_operation, const unsigned int communication_channel, const ArrayView< Number, MemorySpaceType > &ghost_array, const ArrayView< Number, MemorySpaceType > &temporary_storage, std::vector< MPI_Request > &requests) const
IndexSet locally_owned_range_data
unsigned int n_import_indices() const
unsigned int global_to_local(const types::global_dof_index global_index) const
unsigned int local_size() const
bool is_globally_compatible(const Partitioner &part) const
#define Assert(cond, exc)
size_type nth_index_in_set(const size_type local_index) const
IndexSet ghost_indices_data
const std::vector< std::pair< unsigned int, unsigned int > > & ghost_targets() const
void export_to_ghosted_array_start(const unsigned int communication_channel, const ArrayView< const Number, MemorySpaceType > &locally_owned_array, const ArrayView< Number, MemorySpaceType > &temporary_storage, const ArrayView< Number, MemorySpaceType > &ghost_array, std::vector< MPI_Request > &requests) const
const std::vector< std::pair< unsigned int, unsigned int > > & import_targets() const
static const unsigned int invalid_unsigned_int
std::pair< types::global_dof_index, types::global_dof_index > local_range() const
std::vector< std::pair< std::unique_ptr< unsigned int[], void(*)(unsigned int *)>, unsigned int > > import_indices_plain_dev
virtual void reinit(const IndexSet &vector_space_vector_index_set, const IndexSet &read_write_vector_index_set, const MPI_Comm &communicator) override
size_type index_within_set(const size_type global_index) const
static ::ExceptionBase & ExcGhostIndexArrayHasWrongSize(unsigned int arg1, unsigned int arg2, unsigned int arg3)
const IndexSet & ghost_indices() const
types::global_dof_index local_to_global(const unsigned int local_index) const
#define DEAL_II_NAMESPACE_CLOSE
const IndexSet & locally_owned_range() const
void import_from_ghosted_array_finish(const VectorOperation::values vector_operation, const ArrayView< const Number, MemorySpaceType > &temporary_storage, const ArrayView< Number, MemorySpaceType > &locally_owned_storage, const ArrayView< Number, MemorySpaceType > &ghost_array, std::vector< MPI_Request > &requests) const
TrilinosWrappers::types::int_type global_index(const Epetra_BlockMap &map, const ::types::global_dof_index i)
#define DeclException2(Exception2, type1, type2, outsequence)
void initialize_import_indices_plain_dev() const
bool ghost_indices_initialized() const
std::vector< unsigned int > import_indices_chunks_by_rank_data
T max(const T &t, const MPI_Comm &mpi_communicator)
std::pair< types::global_dof_index, types::global_dof_index > local_range_data
virtual const MPI_Comm & get_mpi_communicator() const override