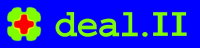 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_solution_transfer_h
17 # define dealii_solution_transfer_h
342 # ifndef DEAL_II_MSVC
343 static_assert(dim == DoFHandlerType::dimension,
344 "The dimension explicitly provided as a template "
345 "argument, and the dimension of the DoFHandlerType "
346 "template argument must match.");
426 std::vector<VectorType> & all_out)
const;
451 "You are attempting an operation for which this object is "
452 "not prepared. This may be because you either did not call "
453 "one of the prepare_*() functions at all, or because you "
454 "called the wrong one for the operation you are currently "
462 "You are attempting to call one of the prepare_*() functions "
463 "of this object to prepare it for an operation for which it "
464 "is already prepared. Specifically, the object was "
465 "previously prepared for pure refinement.");
472 "You are attempting to call one of the prepare_*() functions "
473 "of this object to prepare it for an operation for which it "
474 "is already prepared. Specifically, the object was "
475 "previously prepared for both coarsening and refinement.");
543 const unsigned int active_fe_index_in = 0)
550 const unsigned int active_fe_index_in = 0)
575 std::vector<std::vector<Vector<typename VectorType::value_type>>>
582 #endif // dealii_solutiontransfer_h
#define DeclExceptionMsg(Exception, defaulttext)
Pointerstruct(std::vector< types::global_dof_index > *indices_ptr_in, const unsigned int active_fe_index_in=0)
void prepare_for_pure_refinement()
std::size_t memory_consumption() const
Pointerstruct(std::vector< Vector< typename VectorType::value_type >> *dof_values_ptr_in, const unsigned int active_fe_index_in=0)
SolutionTransfer(const DoFHandlerType &dof)
static ::ExceptionBase & ExcAlreadyPrepForRef()
void prepare_for_coarsening_and_refinement(const std::vector< VectorType > &all_in)
void refine_interpolate(const VectorType &in, VectorType &out) const
std::vector< types::global_dof_index > * indices_ptr
PreparationState prepared_for
std::vector< std::vector< Vector< typename VectorType::value_type > > > dof_values_on_cell
std::size_t memory_consumption() const
static ::ExceptionBase & ExcAlreadyPrepForCoarseAndRef()
void interpolate(const std::vector< VectorType > &all_in, std::vector< VectorType > &all_out) const
#define DEAL_II_NAMESPACE_OPEN
unsigned int active_fe_index
types::global_dof_index n_dofs_old
SmartPointer< const DoFHandlerType, SolutionTransfer< dim, VectorType, DoFHandlerType > > dof_handler
std::vector< Vector< typename VectorType::value_type > > * dof_values_ptr
@ coarsening_and_refinement
std::map< std::pair< unsigned int, unsigned int >, Pointerstruct > cell_map
#define DEAL_II_NAMESPACE_CLOSE
std::vector< std::vector< types::global_dof_index > > indices_on_cell
static ::ExceptionBase & ExcNotPrepared()