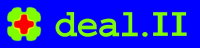 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_mg_block_smoother_h
17 #define dealii_mg_block_smoother_h
50 template <
typename MatrixType,
class RelaxationType,
typename number>
76 template <
class MGMatrixType,
class MGRelaxationType>
138 template <
typename MatrixType,
class RelaxationType,
typename number>
140 const unsigned int steps,
147 , mem(&this->vector_memory)
151 template <
typename MatrixType,
class RelaxationType,
typename number>
155 unsigned int i = matrices.min_level(), max_level = matrices.max_level();
156 for (; i <= max_level; ++i)
164 template <
typename MatrixType,
class RelaxationType,
typename number>
165 template <
class MGMatrixType,
class MGRelaxationType>
168 const MGMatrixType & m,
169 const MGRelaxationType &s)
171 const unsigned int min = m.min_level();
172 const unsigned int max = m.max_level();
174 matrices.resize(
min,
max);
175 smoothers.resize(
min,
max);
177 for (
unsigned int i =
min; i <=
max; ++i)
182 matrices[i] = linear_operator<BlockVector<number>>(
184 smoothers[i] = linear_operator<BlockVector<number>>(matrices[i], s[i]);
189 template <
typename MatrixType,
class RelaxationType,
typename number>
198 template <
typename MatrixType,
class RelaxationType,
typename number>
202 return sizeof(*this) + matrices.memory_consumption() +
203 smoothers.memory_consumption() +
204 this->vector_memory.memory_consumption();
208 template <
typename MatrixType,
class RelaxationType,
typename number>
211 const unsigned int level,
217 unsigned int maxlevel = matrices.max_level();
218 unsigned int steps2 = this->steps;
221 steps2 *= (1 << (maxlevel -
level));
229 if (this->
symmetric && (steps2 % 2 == 0))
232 for (
unsigned int i = 0; i < steps2; ++i)
236 matrices[
level].vmult(*r, u);
237 r->sadd(-1., 1., rhs);
238 smoothers[
level].Tvmult(*
d, *r);
242 matrices[
level].vmult(*r, u);
243 r->sadd(-1., 1., rhs);
244 smoothers[
level].vmult(*
d, *r);
VectorizedArray< Number, width > max(const ::VectorizedArray< Number, width > &x, const ::VectorizedArray< Number, width > &y)
VectorizedArray< Number, width > min(const ::VectorizedArray< Number, width > &x, const ::VectorizedArray< Number, width > &y)
SmartPointer< VectorMemory< BlockVector< number > >, MGSmootherBlock< MatrixType, RelaxationType, number > > mem
SymmetricTensor< 2, dim, Number > d(const Tensor< 2, dim, Number > &F, const Tensor< 2, dim, Number > &dF_dt)
@ symmetric
Matrix is symmetric.
#define DEAL_II_NAMESPACE_OPEN
std::size_t memory_consumption() const
virtual void smooth(const unsigned int level, BlockVector< number > &u, const BlockVector< number > &rhs) const
MGLevelObject< LinearOperator< BlockVector< number > > > smoothers
MGSmootherBlock(const unsigned int steps=1, const bool variable=false, const bool symmetric=false, const bool transpose=false, const bool reverse=false)
void set_reverse(const bool)
void initialize(const MGMatrixType &matrices, const MGRelaxationType &smoothers)
#define DEAL_II_NAMESPACE_CLOSE
MGLevelObject< LinearOperator< BlockVector< number > > > matrices